hw06
.pdf
keyboard_arrow_up
School
Palomar College *
*We aren’t endorsed by this school
Course
128
Subject
Computer Science
Date
May 17, 2024
Type
Pages
17
Uploaded by ConstableSteel14439 on coursehero.com
hw06
May 16, 2024
[1]:
# Initialize Otter
import
otter
grader
=
otter
.
Notebook(
"hw06.ipynb"
)
1
Homework 6: Probability, Simulation, Estimation, and Assess-
ing Models
Please complete this notebook by filling in the cells provided. Before you begin, execute the previous
cell to load the provided tests.
Helpful Resource:
-
Python Reference
: Cheat sheet of helpful array & table methods used in
Data 8!
Recommended Readings
:
*
Randomness
*
Sampling and Empirical Distributions
*
Testing
Hypotheses
Please complete this notebook by filling in the cells provided.
Before you begin, execute the
following cell to setup the notebook by importing some helpful libraries. Each time you start your
server, you will need to execute this cell again.
For all problems that you must write explanations and sentences for, you
must
provide your answer
in the designated space.
Moreover, throughout this homework and all future ones, please
be sure to not re-assign variables throughout the notebook!
For example, if you use
max_temperature
in your answer to one question, do not reassign it later on. Otherwise, you will
fail tests that you thought you were passing previously!
Note: This homework has hidden tests on it. That means even though the tests may
say 100% passed, it doesn’t mean your final grade will be 100%. We will be running
more tests for correctness once everyone turns in the homework.
Directly sharing answers is not okay, but discussing problems with the course staff or with other
students is encouraged.
You should start early so that you have time to get help if you’re stuck.
1
1.1
1. Roulette
[2]:
# Run this cell to set up the notebook, but please don't change it.
# These lines import the Numpy and Datascience modules.
import
numpy
as
np
from
datascience
import
*
import
d8error
# These lines do some fancy plotting magic.
import
matplotlib
%
matplotlib
inline
import
matplotlib.pyplot
as
plt
plt
.
style
.
use(
'fivethirtyeight'
)
import
warnings
warnings
.
simplefilter(
'ignore'
,
FutureWarning
)
A Nevada roulette wheel has 38 pockets and a small ball that rests on the wheel. When the wheel
is spun, the ball comes to rest in one of the 38 pockets. That pocket is declared the winner.
The pockets are labeled 0, 00, 1, 2, 3, 4, … , 36. Pockets 0 and 00 are green, and the other pockets
are alternately red and black.
The table
wheel
is a representation of a Nevada roulette wheel.
Note that
both
columns consist of strings.
Below is an example of a roulette wheel!
Run the cell below to load the
wheel
table.
[3]:
wheel
=
Table
.
read_table(
'roulette_wheel.csv'
, dtype
=
str
)
wheel
[3]:
Pocket | Color
00
| green
0
| green
1
| red
2
| black
3
| red
4
| black
5
| red
6
| black
7
| red
8
| black
… (28 rows omitted)
1.1.1
Betting on Red
If you bet on
red
, you are betting that the winning pocket will be red. This bet
pays 1 to 1
. That
means if you place a one-dollar bet on red, then:
• If the winning pocket is red, you gain 1 dollar. That is, you get your original dollar back,
plus one more dollar.
• if the winning pocket is not red, you lose your dollar. In other words, you gain -1 dollars.
2
Let’s see if you can make money by betting on red at roulette.
Question 1.
Define a function
dollar_bet_on_red
that takes the name of a color and returns
your gain in dollars if that color had won and you had placed a one-dollar bet on red. Remember
that the gain can be negative. Make sure your function returns an integer.
(4 points)
Note:
You can assume that the only colors that will be passed as arguments are red, black, and
green. Your function doesn’t have to check that.
[10]:
def
dollar_bet_on_red
(color):
if
color
==
"red"
:
return
1
else
:
return
-1
[11]:
grader
.
check(
"q1_1"
)
[11]:
q1_1 results: All test cases passed!
Run the cell below to make sure your function is working.
[12]:
print
(dollar_bet_on_red(
'green'
))
print
(dollar_bet_on_red(
'black'
))
print
(dollar_bet_on_red(
'red'
))
-1
-1
1
Question 2.
Add a column labeled
Winnings: Red
to the table
wheel
.
For each pocket, the
column should contain your gain in dollars if that pocket won and you had bet one dollar on red.
Your code should use the function
dollar_bet_on_red
.
(4 points)
[14]:
red_winnings
=
wheel
.
apply(dollar_bet_on_red,
"Color"
)
wheel
=
wheel
.
with_column(
"Winnings: Red"
,red_winnings)
wheel
[14]:
Pocket | Color | Winnings: Red
00
| green | -1
0
| green | -1
1
| red
| 1
2
| black | -1
3
| red
| 1
4
| black | -1
5
| red
| 1
6
| black | -1
7
| red
| 1
8
| black | -1
… (28 rows omitted)
3
[15]:
grader
.
check(
"q1_2"
)
[15]:
q1_2 results: All test cases passed!
1.1.2
Simulating 10 Bets on Red
Roulette wheels are set up so that each time they are spun, the winning pocket is equally likely to
be any of the 38 pockets regardless of the results of all other spins. Let’s see what would happen
if we decided to bet one dollar on red each round.
Question 3.
Create a table
ten_bets
by sampling the table
wheel
to simulate 10 spins of the
roulette wheel.
Your table should have the same three column labels as in
wheel
.
Once you’ve
created that table, set
sum_bets
to your net gain in all 10 bets, assuming that you bet one dollar
on red each time.
(4 points)
Hint:
It may be helpful to print out
ten_bets
after you create it!
[21]:
ten_bets
=
wheel
.
sample(
10
)
sum_bets
=
sum
(ten_bets
.
column(
'Winnings: Red'
))
sum_bets
[21]:
4
[22]:
grader
.
check(
"q1_3"
)
[22]:
q1_3 results: All test cases passed!
Run the cells above a few times to see how much money you would make if you made 10 one-dollar
bets on red. Making a negative amount of money doesn’t feel good, but it is a reality in gambling.
Casinos are a business, and they make money when gamblers lose.
Question 4.
Let’s see what would happen if you made more bets. Define a function
net_gain_red
that takes the number of bets and returns the net gain in that number of one-dollar bets on red.
(4 points)
Hint:
You should use your
wheel
table within your function.
[24]:
def
net_gain_red
(number_bets):
bets_on_red
=
wheel
.
sample(number_bets)
net_gain
=
sum
(bets_on_red
.
column(
"Winnings: Red"
))
return
net_gain
[25]:
grader
.
check(
"q1_4"
)
[25]:
q1_4 results: All test cases passed!
Run the cell below a few times to make sure that the results are similar to those you observed in
the previous exercise.
[28]:
net_gain_red(
10
)
4
[28]:
0
Question 5.
Complete the cell below to simulate the net gain in 200 one-dollar bets on red,
repeating the process 10,000 times. After the cell is run,
all_gains_red
should be an array with
10,000 entries, each of which is the net gain in 200 one-dollar bets on red.
(4 points)
Hint:
Think about which computational tool might be helpful for simulating a process multiple
times. Lab 5 might be a good resource to look at!
Note:
This cell might take a few seconds to run.
[33]:
num_bets
= 200
repetitions
= 10000
all_gains_red
=
make_array()
for
i
in
np
.
arange(repetitions):
sum_red_gain
=
net_gain_red(num_bets)
all_gains_red
=
np
.
append(all_gains_red,sum_red_gain)
len
(all_gains_red)
# Do not change this line! Check that all_gains_red is
␣
↪
length 10000.
[33]:
10000
[34]:
grader
.
check(
"q1_5"
)
[34]:
q1_5 results: All test cases passed!
Run the cell below to visualize the results of your simulation.
[35]:
gains
=
Table()
.
with_columns(
'Net Gain on Red'
, all_gains_red)
gains
.
hist(bins
=
np
.
arange(
-80
,
41
,
4
))
5
Question 6:
Using the histogram above, decide whether the following statement is true or false:
If you make 200 one-dollar bets on red, your chance of losing money is more than 50%.
Assign
loss_more_than_50
to either
True
or
False
depending on your answer to the question.
(4
points)
[37]:
loss_more_than_50
=
True
[38]:
grader
.
check(
"q1_6"
)
[38]:
q1_6 results: All test cases passed!
1.1.3
Betting on a Split
If betting on red doesn’t seem like a good idea, maybe a gambler might want to try a different bet.
A bet on a
split
is a bet on two consecutive numbers such as 5 and 6. This bets pays 17 to 1. That
means if you place a one-dollar bet on the split 5 and 6, then:
• If the winning pocket is either 5 or 6, your gain is 17 dollars.
• If any other pocket wins, you lose your dollar, so your gain is -1 dollars.
Question 7.
Define a function
dollar_bet_on_split
that takes a pocket number and returns
your gain in dollars if that pocket won and you had bet one dollar on the 5-6 split.
(4 points)
Hint:
Remember that the pockets are represented as strings.
6
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Task 3: Statistics using arrays:
by java programming
With the spread of COVID 19, the HR department in a company has decided to conduct some statistics
among the employees in order to determine the number of infections according to some conditions.
For each employee, they have to record the code, name, age, whether he/she was infected or no and
the remaining days of leaves for him/her.
You are requested to write the program that maintains the lists of details for the employees as
mentioned above using the concept of arrays. The program repeats the display of a menu of services
until the user decides to exit.
1. Start by initializing the employee details by reading them from the keyboard.
2. Repeat the display of a menu of 4 services, perform the required task according to the user’s
choice and asks the user whether he/she wants to repeat or no. You need to choose one service
from each category (‘A’,’B’,’C’,’D’)
a. A. Display the total number of employees that were infected
b. B.…
arrow_forward
DESIGN YOUR OWN SETTING
Task 5: Devise your own setting for storing and searching the data in an array of non-negative integers redundantly. You may just describe the setting without having to give an explicit algorithm to explain the process by which data is stored. You should explain how hardware failures can be detected in your method. Once you have described the setting, complete the following:
Write a pseudocode function to describe an algorithm where the stored data can be searched for a value key: if the data is found, its location in the original array should be returned; -1 should be returned if the data is not found; -2 should be returned if there is a data storage error
Include a short commentary explaining why your pseudocode works
Describe the worst-case and best-case inputs to your search algorithm
Derive the worst-case and best-case running times for the search algorithm
Derive the Theta notation for the worst-case and best-case running times
Maximum word…
arrow_forward
C++ Progrmaing
Instructions
Redo Programming Exercise 6 of Chapter 8 using dynamic arrays. The instructions have been posted for your convenience.
The history teacher at your school needs help in grading a True/False test. The students’ IDs and test answers are stored in a file. The first entry in the file contains answers to the test in the form:
TFFTFFTTTTFFTFTFTFTT
Every other entry in the file is the student ID, followed by a blank, followed by the student’s responses. For example, the entry:
ABC54301 TFTFTFTT TFTFTFFTTFT
indicates that the student ID is ABC54301 and the answer to question 1 is True, the answer to question 2 is False, and so on. This student did not answer question 9 (note the empty space). The exam has 20 questions, and the class has more than 150 students. Each correct answer is awarded two points, each wrong answer gets one point deducted, and no answer gets zero points. Write a program that processes the test data. The output should be the student’s ID,…
arrow_forward
Programming Language: C++
Please output it in the console not a text file.
For this exerc ise, you will define a struct. It will contain a first name, last name, ID, and GPA. You will ask the user how many students to enter, create a dynamic
array of that size to get data from the user. The array data will initially be inserted into the array in ID order. Then you will display the array in a table, sort it by last
name with std::sort, redisplay it, and then search for five students (using last name and a binary search) and for any names found display the first name, last name,
and GPA,
The struct will be named Student and include strings for firstName, lastName, ID, and a float for the GPA. The ID will be in the format 00xxxxxx where the x's are
replaced by digits and in the range between 111111 and 999999. The GPA will be a floating-point number in the range 2.0 to 4.2 and will be displayed to 2 digits
after the decimal point.
arrow_forward
PYTHON
In order to parse data to start a simulation, users must enter data correctly.
Suppose that the simulation requires 2 information from the user, which is called 'soil_key' and 'soil_data.' The user will enter 'soil_key' first, following with 'soil_data.' We need to check if the user enters the 'soil_data' data correctly. All elements in soil data ('soil_data') must be characters defined in the soil keys ('soil_key'). Both soil_key and soil_data are lists. Consider an example:
INPUT:
soil_key = ['C', 'Clay', ' B', 'Bedrock', ' V', 'Void']
soil_data1 = [ ['V', 'C', 'V'], ['C', 'V', ' '], ['B', 'M', 'B'] ]
soil_data2 = [ ['B', 'C', 'V'], ['C', 'V', ' B'], ['B', 'C', 'B'] ]
OUTPUT:
soil_data1: check data again. Parsing failed!
soil_data2: Parsing successfully.
Write a python function that checks if the soil data is being entered correctly. Return True and print 'Parsing successfully' for correct data. Otherwise, return False and tells the user 'check data again. Parsing…
arrow_forward
User-defined ordinal data types: pros and cons? Associative arrays have pros and cons, which are discussed below.
arrow_forward
Array Challenge
4. Write a program in C# to copy the elements of one array into another array.Test Data :Input the number of elements to be stored in the array :3Input 3 elements in the array :element - 0 : 15element - 1 : 10element - 2 : 12Expected Output :The elements stored in the first array are :15 10 12The elements copied into the second array are :15 10 12
arrow_forward
ASSEMBLY
It is preferable to pass Arrays by reference when calling subroutines.
True
False
arrow_forward
C++ Language
Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks
Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…
arrow_forward
C++ Language
Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks
Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…
arrow_forward
Array Challenge
3. Write a program in C# to find the sum of all elements of the array.Test Data :Input the number of elements to be stored in the array :3Input 3 elements in the array :element - 0 : 2element - 1 : 5element - 2 : 8Expected Output :Sum of all elements stored in the array is : 15
arrow_forward
I need a code of this in C language.
Input
1. Number of rows
Constraints
The value is guaranteed to be >= 3 and <= 100.
Sample
3
2. Number of columns
Constraints
The value is guaranteed to be >= 3 and <= 100.
Sample
3
3. Elements
Description
The elements of the multidimensional array depending on the number of rows and columns
Constraints
All elements are non-negative and are all less than 100.
Output
The first line will contain a message prompt to input the number of rows. The second line will contain a message prompt to input the number of columns. The succeeding lines will contain message prompts for the elements of the multidimensional array. Lastly, a blank line is printed before the rotated 90-degrees anti-clockwise 2D array.
For example this should be the output.
Enter the number of rows: 3
Enter the number of columns: 3
Row #1: 1 2 3
Row #2:·4 5 6
Row #3: 7 8 9
3 6 9
2 5 8
1 4 7
arrow_forward
Finding the common members of two dynamic arrays: Write a program that first reads two arrays and then finds their common members (intersection). The program should first read an integer for the size of the array and then should create the two arrays dynamically (using new) and should read the numbers into array1 and array2 and then should find the common members and show it.
Note: The list of the common members should be unique. That is no member should be listed more than once.
Tip: A simple for loop is needed on one of the arrays (like array1) and one inner for loop for on array2. Then for each member of array1, if we find any matching member in array2, we record it.
Sample run:
Enter the number of elements of array 1: 7
Enter the elements of array 1: 10 12 13 10 14 15 12
Enter the number of elements of array 2: 5
Enter the elements of array 2: 17 12 10 19 12
The common members of array1 and array2 are: 12 10
arrow_forward
Code in Perl
Create an array which holds a list of Video Games, consisting of title, the year it was released (guess if you don’t know) , platform (NES, XBOX One, etc) and publisher (in that order). Add at least ten albums to the list. Then, use the split function and a for or foreach loop to display the publisher followed by the title and platform for each list element.
arrow_forward
Query Board Python or Java(Preferred Python please) Thank you!
Programming challenge description:
There is a board (matrix). Every cell of the board contains one integer, which is 0 initially.The following operations can be applied to the Query Board:SetRow i x: change all values in the cells on row "i" to value "x".SetCol j x: change all values in the cells on column "j" to value "x".QueryRow i: output the sum of values on row "i".QueryCol j: output the sum of values on column "j".The board's dimensions are 256x256."i" and "j" are integers from 0 to 255."x" is an integer from 0 to 31.
Input:
Your program should read lines from standard input. Each line contains one of the above operations.
Output:
For each query, output the result of the query.
Test 1
Test InputDownload Test 1 Input
SetCol 32 20 SetRow 15 7 SetRow 16 31 QueryCol 32 SetCol 2 14 QueryRow 10
Expected OutputDownload Test 1 Input
5118 34
arrow_forward
A dequeue is a list from which elements can be inserted or deleted at either end
a. Develop an array based implementation for dequeue.
b. Develop a pointer based implementation dequeue.
arrow_forward
please code in python
Forbidden concepts: arrays/lists (data structures), recursion, custom classes
Watch this video on the Fibonacci sequence. The first two numbers of the sequence are 1 and 1. The subsequent numbers are the sum of the previous two numbers. Create a loop that calculates the Fibonacci sequence of the first 25 numbers and prints each one.Lastly, state the 25th and 24th sequence in a sentence, and explain how the golden ratio is conducted from it, including what it is.Note: you don’t know what the 24th and 25th numbers are, get the program to find out and calculate itself what the ratio is.
arrow_forward
Q3] Create a new function with the name DrawHPyramid that takes one parameter r, which is the
number of rows. This function rather than using the ghạracter it uses + and - to draw the pyramid,
use recursion when implementing this function
arrow_forward
Grades 0-100 will be assigned randomly in a series with 30 elements. Taking their averages (shown on the screen), those above the average will be divided into an array and the ones below will be divided into an array by the function named "plenty" (two different sequences will be obtained). (Pointer is required.)Then, with the function named "gor", the grades of the whole class, those above the average, those below the average will be displayed on the screen.Write the C program code that performs the above operations.
arrow_forward
Write JAVA code
General Problem Description:
It is desired to develop a directory application based on the use of a double-linked list data structure, in which students are kept in order according to their student number. In this context, write the Java codes that will meet the requirements given in detail below.
Requirements:
Create a class named Student to represent students. In the Student class; student number, name and surname and phone numbers for communication are kept. Student's multiple phones
number (multiple mobile phones, home phones, etc.) so phone numbers information will be stored in an “ArrayList”. In the Student class; parameterless, taking all parameters and
It is sufficient to have 3 constructor methods, including a copy constructor, get/set methods and toString.
arrow_forward
Input:
The first line will consists of one integer T denoting the number of
test cases.
For each test case:
1) The first line consists of two integers N and K, N being the
number of elements in the array and K denotes the number of
steps of rotation.
2) The next line consists of N space separated integers, denoting
the elements of the array A.
Output:
Print the required array.
Constraints:
arrow_forward
def drop_uninformative_objects(X, y):# your code herereturn X_subset, y_subset
# TEST drop_uninformative_objects functionA = pd.DataFrame(np.array([[0, 3, np.nan],[4, np.nan, np.nan],[np.nan, 6, 7],[np.nan, np.nan, np.nan],[5, 5, 5],[np.nan, 8, np.nan],]))b = pd.Series(np.arange(6))A_subset, b_subset = drop_uninformative_objects(A, b)
assert A_subset.shape == (3, 3)assert b_subset.shape == (3,)
arrow_forward
Q# 3: Write the pre and post-conditions for the following design specification:
A procedure search has three parameters: arr, lookfor, and found. Parameter arr is an integer
array with a range of 1 .. 200. Parameter lookfor is an integer parameter. If lookfor occur in arr
then found is set to one otherwise, it is set to zero.
arrow_forward
Array Challenge
5. Write a program in C# to count a total number of duplicate elements in an array.Test Data :Input the number of elements to be stored in the array :3Input 3 elements in the array :element - 0 : 5element - 1 : 1element - 2 : 1Expected Output :Total number of duplicate elements found in the array is : 1
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
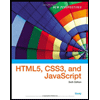
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Related Questions
- Task 3: Statistics using arrays: by java programming With the spread of COVID 19, the HR department in a company has decided to conduct some statistics among the employees in order to determine the number of infections according to some conditions. For each employee, they have to record the code, name, age, whether he/she was infected or no and the remaining days of leaves for him/her. You are requested to write the program that maintains the lists of details for the employees as mentioned above using the concept of arrays. The program repeats the display of a menu of services until the user decides to exit. 1. Start by initializing the employee details by reading them from the keyboard. 2. Repeat the display of a menu of 4 services, perform the required task according to the user’s choice and asks the user whether he/she wants to repeat or no. You need to choose one service from each category (‘A’,’B’,’C’,’D’) a. A. Display the total number of employees that were infected b. B.…arrow_forwardDESIGN YOUR OWN SETTING Task 5: Devise your own setting for storing and searching the data in an array of non-negative integers redundantly. You may just describe the setting without having to give an explicit algorithm to explain the process by which data is stored. You should explain how hardware failures can be detected in your method. Once you have described the setting, complete the following: Write a pseudocode function to describe an algorithm where the stored data can be searched for a value key: if the data is found, its location in the original array should be returned; -1 should be returned if the data is not found; -2 should be returned if there is a data storage error Include a short commentary explaining why your pseudocode works Describe the worst-case and best-case inputs to your search algorithm Derive the worst-case and best-case running times for the search algorithm Derive the Theta notation for the worst-case and best-case running times Maximum word…arrow_forwardC++ Progrmaing Instructions Redo Programming Exercise 6 of Chapter 8 using dynamic arrays. The instructions have been posted for your convenience. The history teacher at your school needs help in grading a True/False test. The students’ IDs and test answers are stored in a file. The first entry in the file contains answers to the test in the form: TFFTFFTTTTFFTFTFTFTT Every other entry in the file is the student ID, followed by a blank, followed by the student’s responses. For example, the entry: ABC54301 TFTFTFTT TFTFTFFTTFT indicates that the student ID is ABC54301 and the answer to question 1 is True, the answer to question 2 is False, and so on. This student did not answer question 9 (note the empty space). The exam has 20 questions, and the class has more than 150 students. Each correct answer is awarded two points, each wrong answer gets one point deducted, and no answer gets zero points. Write a program that processes the test data. The output should be the student’s ID,…arrow_forward
- Programming Language: C++ Please output it in the console not a text file. For this exerc ise, you will define a struct. It will contain a first name, last name, ID, and GPA. You will ask the user how many students to enter, create a dynamic array of that size to get data from the user. The array data will initially be inserted into the array in ID order. Then you will display the array in a table, sort it by last name with std::sort, redisplay it, and then search for five students (using last name and a binary search) and for any names found display the first name, last name, and GPA, The struct will be named Student and include strings for firstName, lastName, ID, and a float for the GPA. The ID will be in the format 00xxxxxx where the x's are replaced by digits and in the range between 111111 and 999999. The GPA will be a floating-point number in the range 2.0 to 4.2 and will be displayed to 2 digits after the decimal point.arrow_forwardPYTHON In order to parse data to start a simulation, users must enter data correctly. Suppose that the simulation requires 2 information from the user, which is called 'soil_key' and 'soil_data.' The user will enter 'soil_key' first, following with 'soil_data.' We need to check if the user enters the 'soil_data' data correctly. All elements in soil data ('soil_data') must be characters defined in the soil keys ('soil_key'). Both soil_key and soil_data are lists. Consider an example: INPUT: soil_key = ['C', 'Clay', ' B', 'Bedrock', ' V', 'Void'] soil_data1 = [ ['V', 'C', 'V'], ['C', 'V', ' '], ['B', 'M', 'B'] ] soil_data2 = [ ['B', 'C', 'V'], ['C', 'V', ' B'], ['B', 'C', 'B'] ] OUTPUT: soil_data1: check data again. Parsing failed! soil_data2: Parsing successfully. Write a python function that checks if the soil data is being entered correctly. Return True and print 'Parsing successfully' for correct data. Otherwise, return False and tells the user 'check data again. Parsing…arrow_forwardUser-defined ordinal data types: pros and cons? Associative arrays have pros and cons, which are discussed below.arrow_forward
- Array Challenge 4. Write a program in C# to copy the elements of one array into another array.Test Data :Input the number of elements to be stored in the array :3Input 3 elements in the array :element - 0 : 15element - 1 : 10element - 2 : 12Expected Output :The elements stored in the first array are :15 10 12The elements copied into the second array are :15 10 12arrow_forwardASSEMBLY It is preferable to pass Arrays by reference when calling subroutines. True Falsearrow_forwardC++ Language Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…arrow_forward
- C++ Language Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…arrow_forwardArray Challenge 3. Write a program in C# to find the sum of all elements of the array.Test Data :Input the number of elements to be stored in the array :3Input 3 elements in the array :element - 0 : 2element - 1 : 5element - 2 : 8Expected Output :Sum of all elements stored in the array is : 15arrow_forwardI need a code of this in C language. Input 1. Number of rows Constraints The value is guaranteed to be >= 3 and <= 100. Sample 3 2. Number of columns Constraints The value is guaranteed to be >= 3 and <= 100. Sample 3 3. Elements Description The elements of the multidimensional array depending on the number of rows and columns Constraints All elements are non-negative and are all less than 100. Output The first line will contain a message prompt to input the number of rows. The second line will contain a message prompt to input the number of columns. The succeeding lines will contain message prompts for the elements of the multidimensional array. Lastly, a blank line is printed before the rotated 90-degrees anti-clockwise 2D array. For example this should be the output. Enter the number of rows: 3 Enter the number of columns: 3 Row #1: 1 2 3 Row #2:·4 5 6 Row #3: 7 8 9 3 6 9 2 5 8 1 4 7arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
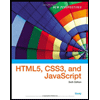
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning