Design a Client class that interfaces with your SQLDatabase Server (from the class Server). The Client sends a SQLQuery to the SQLDatabase and receives a Dataframe from the SQLDatabase. Your class should contain the default __dunders__ and potentially introduce some custom __dunders__. classServer.py (Just change if this file has something doesn't run or doesn't fit with new code) import sqlite3 import pandas as pd class Server: def __init__(self, db_name): self.db = sqlite3.connect(db_name) self.cursor = self.db.cursor() def __enter__(self): return self def __exit__(self, exc_type, exc_val, exc_tb): self.db.close() def create_table(self, table_schema): self.cursor.execute(table_schema) self.db.commit() def insert_data(self, table_name, data): for i in range(len(data)): keys = ", ".join(data.columns) values = ", ".join([f"'{value}'" if pd.isna(value) else str(value) for value in data.iloc[i]]) self.cursor.execute(f"INSERT INTO {table_name} ({keys}) VALUES ({values})") self.db.commit() def search_data(self, table_name, year): self.cursor.execute(f"SELECT * FROM {table_name} WHERE year={year}") results = self.cursor.fetchall() if results: # Convert the fetched data into a DataFrame df = pd.DataFrame(results, columns=[desc[0] for desc in self.cursor.description]) return df else: return None def delete_data(self, table_name, year): self.cursor.execute(f"DELETE FROM {table_name} WHERE year={year}") self.db.commit() return self.cursor.rowcount > 0
Design a Client class that interfaces with your SQLDatabase Server (from the class Server). The Client sends a SQLQuery to the SQLDatabase and receives a Dataframe from the SQLDatabase. Your class should contain the default __dunders__ and potentially introduce some custom __dunders__. classServer.py (Just change if this file has something doesn't run or doesn't fit with new code) import sqlite3 import pandas as pd class Server: def __init__(self, db_name): self.db = sqlite3.connect(db_name) self.cursor = self.db.cursor() def __enter__(self): return self def __exit__(self, exc_type, exc_val, exc_tb): self.db.close() def create_table(self, table_schema): self.cursor.execute(table_schema) self.db.commit() def insert_data(self, table_name, data): for i in range(len(data)): keys = ", ".join(data.columns) values = ", ".join([f"'{value}'" if pd.isna(value) else str(value) for value in data.iloc[i]]) self.cursor.execute(f"INSERT INTO {table_name} ({keys}) VALUES ({values})") self.db.commit() def search_data(self, table_name, year): self.cursor.execute(f"SELECT * FROM {table_name} WHERE year={year}") results = self.cursor.fetchall() if results: # Convert the fetched data into a DataFrame df = pd.DataFrame(results, columns=[desc[0] for desc in self.cursor.description]) return df else: return None def delete_data(self, table_name, year): self.cursor.execute(f"DELETE FROM {table_name} WHERE year={year}") self.db.commit() return self.cursor.rowcount > 0
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Design a Client class that interfaces with your SQLDatabase Server (from the class Server). The Client sends a SQLQuery to the SQLDatabase and receives a Dataframe from the SQLDatabase. Your class should contain the default __dunders__ and potentially introduce some custom __dunders__.
classServer.py (Just change if this file has something doesn't run or doesn't fit with new code)
import sqlite3
import pandas as pd
class Server:
def __init__(self, db_name):
self.db = sqlite3.connect(db_name)
self.cursor = self.db.cursor()
def __enter__(self):
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.db.close()
def create_table(self, table_schema):
self.cursor.execute(table_schema)
self.db.commit()
def insert_data(self, table_name, data):
for i in range(len(data)):
keys = ", ".join(data.columns)
values = ", ".join([f"'{value}'" if pd.isna(value) else str(value) for value in data.iloc[i]])
self.cursor.execute(f"INSERT INTO {table_name} ({keys}) VALUES ({values})")
self.db.commit()
def search_data(self, table_name, year):
self.cursor.execute(f"SELECT * FROM {table_name} WHERE year={year}")
results = self.cursor.fetchall()
if results:
# Convert the fetched data into a DataFrame
df = pd.DataFrame(results, columns=[desc[0] for desc in self.cursor.description])
return df
else:
return None
def delete_data(self, table_name, year):
self.cursor.execute(f"DELETE FROM {table_name} WHERE year={year}")
self.db.commit()
return self.cursor.rowcount > 0
import pandas as pd
class Server:
def __init__(self, db_name):
self.db = sqlite3.connect(db_name)
self.cursor = self.db.cursor()
def __enter__(self):
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.db.close()
def create_table(self, table_schema):
self.cursor.execute(table_schema)
self.db.commit()
def insert_data(self, table_name, data):
for i in range(len(data)):
keys = ", ".join(data.columns)
values = ", ".join([f"'{value}'" if pd.isna(value) else str(value) for value in data.iloc[i]])
self.cursor.execute(f"INSERT INTO {table_name} ({keys}) VALUES ({values})")
self.db.commit()
def search_data(self, table_name, year):
self.cursor.execute(f"SELECT * FROM {table_name} WHERE year={year}")
results = self.cursor.fetchall()
if results:
# Convert the fetched data into a DataFrame
df = pd.DataFrame(results, columns=[desc[0] for desc in self.cursor.description])
return df
else:
return None
def delete_data(self, table_name, year):
self.cursor.execute(f"DELETE FROM {table_name} WHERE year={year}")
self.db.commit()
return self.cursor.rowcount > 0
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
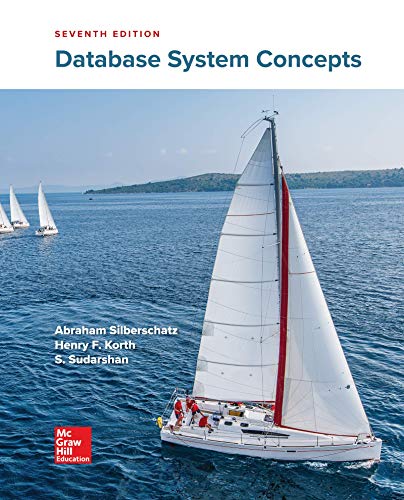
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
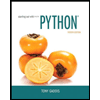
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
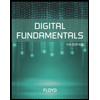
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
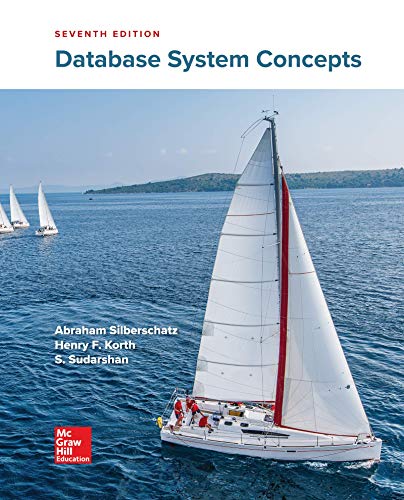
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
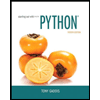
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
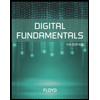
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
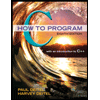
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
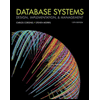
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
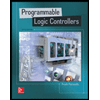
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education