Help with Python Turtle, struggling with last few steps to complete what I want, the following:1. Make python window when generating island bigger to view entire map, or zooming out to view entire island.(Currently only bottom half is visible, need to see and show the whole island).2. Combining the three popup GUIs into one where you can input all the values at once (mountains, lakes, rivers.3. River code needs fix, actually flow through the entire island from beach to beach, number of rivers = cuts through the island on a horizontal.4. Finally, being able to export the final result of the island as a PNG/jpeg to desktop files, if possible.The code: import turtleimport randomimport tkinter as tkfrom tkinter import simpledialog # Define colorsocean = "#000066"sand = "#ffff66"grass = "#00cc00"lake = "#0066ff"mountain_color = "#808080" # Gray color for mountains # Store mountain positionsmountain_positions = [] def draw_irregular_circle(radius, line_color, fill_color, irregularity=10, spikeyness=0.2): my_turtle.color(line_color) my_turtle.fillcolor(fill_color) my_turtle.begin_fill() angle = 0 while angle < 360: angle += random.uniform(10, 60) if angle > 360: angle = 360 actual_radius = radius + random.uniform(irregularity * spikeyness, irregularity) * random.choice([-1, 1]) my_turtle.circle(actual_radius, angle - my_turtle.heading()) my_turtle.end_fill() def draw_triangle(size, line_color, fill_color): my_turtle.color(line_color) my_turtle.fillcolor(fill_color) my_turtle.begin_fill() for _ in range(3): my_turtle.forward(size) my_turtle.left(120) my_turtle.end_fill() def move_turtle(x, y): my_turtle.penup() my_turtle.goto(x, y) my_turtle.pendown() def draw_lake(): x = random.randint(-200, 200) y = random.randint(-150, 200) move_turtle(x, y) draw_irregular_circle(60, lake, lake, 10, 0.3) # Increased radius and irregularity def draw_mountain(): x = random.randint(-200, 200) y = random.randint(-150, 200) mountain_positions.append((x, y)) def draw_river(): move_turtle(-200, 200) my_turtle.setheading(0) my_turtle.color(ocean) my_turtle.pendown() my_turtle.forward(400) my_turtle.penup() def get_user_input(): root = tk.Tk() root.withdraw() num_lakes = simpledialog.askinteger("Input", "How many lakes?", minvalue=1, maxvalue=10) num_mountains = simpledialog.askinteger("Input", "How many mountains?", minvalue=1, maxvalue=10) num_rivers = simpledialog.askinteger("Input", "How many rivers?", minvalue=1, maxvalue=10) root.destroy() return num_lakes, num_mountains, num_rivers # Get user input for number of lakes, mountains, and riversnum_lakes, num_mountains, num_rivers = get_user_input() # Initialize screen and turtlescreen = turtle.Screen()screen.bgcolor(ocean)screen.title("Island Generator")my_turtle = turtle.Turtle()my_turtle.pensize(4)my_turtle.shape("circle") # Draw the sand and grass areas with irregular bordersdraw_irregular_circle(450, sand, sand, 30, 0.4) # Increased sizemove_turtle(0, 120)draw_irregular_circle(360, grass, grass, 25, 0.4) # Increased size # Draw lakes, mountains, and riversfor _ in range(num_lakes): draw_lake()for _ in range(num_mountains): draw_mountain()for pos in mountain_positions: move_turtle(pos[0], pos[1]) draw_triangle(135, mountain_color, mountain_color) # Increased sizefor _ in range(num_rivers): draw_river() turtle.done()
Help with Python Turtle, struggling with last few steps to complete what I want, the following:
1. Make python window when generating island bigger to view entire map, or zooming out to view entire island.
(Currently only bottom half is visible, need to see and show the whole island).
2. Combining the three popup GUIs into one where you can input all the values at once (mountains, lakes, rivers.
3. River code needs fix, actually flow through the entire island from beach to beach, number of rivers = cuts through the island on a horizontal.
4. Finally, being able to export the final result of the island as a PNG/jpeg to desktop files, if possible.
The code:
import turtle
import random
import tkinter as tk
from tkinter import simpledialog
# Define colors
ocean = "#000066"
sand = "#ffff66"
grass = "#00cc00"
lake = "#0066ff"
mountain_color = "#808080" # Gray color for mountains
# Store mountain positions
mountain_positions = []
def draw_irregular_circle(radius, line_color, fill_color, irregularity=10, spikeyness=0.2):
my_turtle.color(line_color)
my_turtle.fillcolor(fill_color)
my_turtle.begin_fill()
angle = 0
while angle < 360:
angle += random.uniform(10, 60)
if angle > 360:
angle = 360
actual_radius = radius + random.uniform(irregularity * spikeyness, irregularity) * random.choice([-1, 1])
my_turtle.circle(actual_radius, angle - my_turtle.heading())
my_turtle.end_fill()
def draw_triangle(size, line_color, fill_color):
my_turtle.color(line_color)
my_turtle.fillcolor(fill_color)
my_turtle.begin_fill()
for _ in range(3):
my_turtle.forward(size)
my_turtle.left(120)
my_turtle.end_fill()
def move_turtle(x, y):
my_turtle.penup()
my_turtle.goto(x, y)
my_turtle.pendown()
def draw_lake():
x = random.randint(-200, 200)
y = random.randint(-150, 200)
move_turtle(x, y)
draw_irregular_circle(60, lake, lake, 10, 0.3) # Increased radius and irregularity
def draw_mountain():
x = random.randint(-200, 200)
y = random.randint(-150, 200)
mountain_positions.append((x, y))
def draw_river():
move_turtle(-200, 200)
my_turtle.setheading(0)
my_turtle.color(ocean)
my_turtle.pendown()
my_turtle.forward(400)
my_turtle.penup()
def get_user_input():
root = tk.Tk()
root.withdraw()
num_lakes = simpledialog.askinteger("Input", "How many lakes?", minvalue=1, maxvalue=10)
num_mountains = simpledialog.askinteger("Input", "How many mountains?", minvalue=1, maxvalue=10)
num_rivers = simpledialog.askinteger("Input", "How many rivers?", minvalue=1, maxvalue=10)
root.destroy()
return num_lakes, num_mountains, num_rivers
# Get user input for number of lakes, mountains, and rivers
num_lakes, num_mountains, num_rivers = get_user_input()
# Initialize screen and turtle
screen = turtle.Screen()
screen.bgcolor(ocean)
screen.title("Island Generator")
my_turtle = turtle.Turtle()
my_turtle.pensize(4)
my_turtle.shape("circle")
# Draw the sand and grass areas with irregular borders
draw_irregular_circle(450, sand, sand, 30, 0.4) # Increased size
move_turtle(0, 120)
draw_irregular_circle(360, grass, grass, 25, 0.4) # Increased size
# Draw lakes, mountains, and rivers
for _ in range(num_lakes):
draw_lake()
for _ in range(num_mountains):
draw_mountain()
for pos in mountain_positions:
move_turtle(pos[0], pos[1])
draw_triangle(135, mountain_color, mountain_color) # Increased size
for _ in range(num_rivers):
draw_river()
turtle.done()

Step by step
Solved in 2 steps

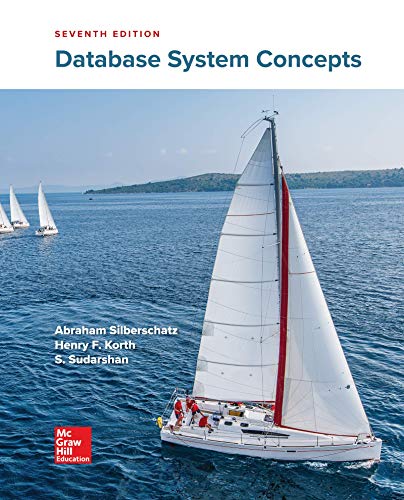
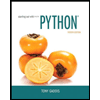
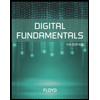
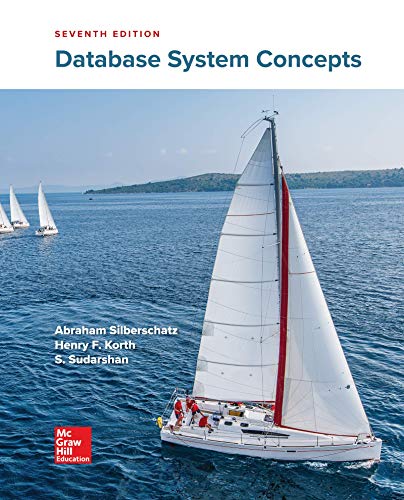
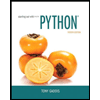
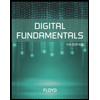
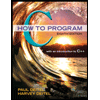
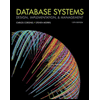
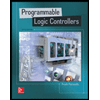