Modify the inventory.c program by adding a price member to the part structure. The insert function should ask the user for the price of a new item. The search and print functions should display the price. The print function calls qsort to sort the inventory array before it prints the parts. The qsort function uses a comparison function. The prototype of the comparison function is int compare parts (const void *p, const void q); that sorts parts by using price member. Expected output: Enter operation code: i Enter part number: 528 Enter part name: Disk drive Enter price: 20 Enter quantity on hand: 10 Enter operation code: i Enter part number: 914 Enter part name: Printer cable Enter price: 5 Enter quantity on hand: 20 Enter operation code: i Enter part number: 25 Enter part name: Printer Enter price: 150 Enter quantity on hand: 4 Enter operation code: p Part Number Part Name 914 Printer cable 528 Disk drive 25 Printer Enter operation code: s Enter part number: 528 Part name: Disk drive Price: $20.00 Quantity on hand: 10 Enter operation code: q Price Quantity on Hand 20 $ 5.00 $ 20.00 10 $ 150.00 4
Modify the inventory.c program by adding a price member to the part structure. The insert function should ask the user for the price of a new item. The search and print functions should display the price. The print function calls qsort to sort the inventory array before it prints the parts. The qsort function uses a comparison function. The prototype of the comparison function is int compare parts (const void *p, const void q); that sorts parts by using price member. Expected output: Enter operation code: i Enter part number: 528 Enter part name: Disk drive Enter price: 20 Enter quantity on hand: 10 Enter operation code: i Enter part number: 914 Enter part name: Printer cable Enter price: 5 Enter quantity on hand: 20 Enter operation code: i Enter part number: 25 Enter part name: Printer Enter price: 150 Enter quantity on hand: 4 Enter operation code: p Part Number Part Name 914 Printer cable 528 Disk drive 25 Printer Enter operation code: s Enter part number: 528 Part name: Disk drive Price: $20.00 Quantity on hand: 10 Enter operation code: q Price Quantity on Hand 20 $ 5.00 $ 20.00 10 $ 150.00 4
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#define NAME_LEN 25
#define MAX_PARTS 100
struct part {
int number;
char name[NAME_LEN + 1];
int on_hand;
double price;
} inventory[MAX_PARTS];
int num_parts = 0;
int find_part(int number);
void insert(void);
void search(void);
void update(void);
void print(void);
int read_line(char str[], int n);
int main(void)
{
char code;
for (;;) {
printf("Enter operation code: ");
scanf(" %c", &code);
while (getchar() != '\n') /* skips to end of line */
;
switch (code) {
case 'i': insert();
break;
case 's': search();
break;
case 'u': update();
break;
case 'p': print();
break;
case 'q': return 0;
default: printf("Illegal code\n");
}
printf("\n");
}
}
int find_part(int number)
{
int i;
for (i = 0; i < num_parts; i++)
if (inventory[i].number == number)
return i;
return -1;
}
void insert(void)
{
int part_number;
if (num_parts == MAX_PARTS) {
printf("Database is full; can't add more parts.\n");
return;
}
printf("Enter part number: ");
scanf("%d", &part_number);
if (find_part(part_number) >= 0) {
printf("Part already exists.\n");
return;
}
inventory[num_parts].number = part_number;
printf("Enter part name: ");
read_line(inventory[num_parts].name, NAME_LEN);
printf("Enter price: ");
scanf("%lf", &inventory[num_parts].price);
printf("Enter quantity on hand: ");
scanf("%d", &inventory[num_parts].on_hand);
num_parts++;
}
void search(void)
{
int i, number;
printf("Enter part number: ");
scanf("%d", &number);
i = find_part(number);
if (i >= 0) {
printf("Part name: %s\n", inventory[i].name);
printf("Price: $%.2f\n", inventory[i].price);
printf("Quantity on hand: %d\n", inventory[i].on_hand);
}
else
printf("Part not found.\n");
}
void update(void)
{
int i, number, change;
printf("Enter part number: ");
scanf("%d", &number);
i = find_part(number);
if (i >= 0) {
printf("Enter change in quantity on hand: ");
scanf("%d", &change);
inventory[i].on_hand += change;
}
else
printf("Part not found.\n");
}
void print(void)
{
int i;
double price;
// sort the inventory array by price
qsort(inventory, num_parts, sizeof(struct part), compare_parts);
printf("Part Number Part Name "
"Price Quantity on Hand\n");
for (i = 0; i < num_parts; i++) {
price = inventory[i].price;
printf("%7d %-25s$%11.2f%11d\n", inventory[i].number,
inventory[i].name, price, inventory[i].on_hand);
}
}
// comparison function for qsort, sorts by price
int compare_parts(const void *p, const void *q)
{
const struct part *part1 = p;
const struct part *part2 = q;
if (part1->price < part2->price)
return -1;
else if (part1->price == part2->price)
return 0;
else
return 1;
}
int read_line(char str[], int n)
{
int ch, i = 0;
while (isspace(ch = getchar()))
;
while (ch != '\n' && ch != EOF) {
if (i < n)
str[i++] = ch;
ch = getchar();
}
str[i] = '\0';
return i;
}

Transcribed Image Text:Modify the inventory.c program by adding a price member to the part structure. The insert
function should ask the user for the price of a new item. The search and print functions should
display the price.
The print function calls gsort to sort the inventory array before it prints the parts. The
qsort function uses a comparison function. The prototype of the comparison function is
int compare parts (const void *p, const void q);
that sorts parts by using price member.
Expected output:
Enter operation code: i
Enter part number: 528
Enter part name: Disk drive
Enter price: 20
Enter quantity on hand: 10
Enter operation code: i
Enter part number: 914
Enter part name: Printer cable
Enter price: 5
Enter quantity on hand: 20
Enter operation code: i
Enter part number: 25
Enter part name: Printer
Enter price: 150
Enter quantity on hand: 4
Enter
Enter operation code: p
Part Number Part Name
Printer cable
Disk drive
Printer
914
528
25
Enter operation code: s
Enter part number: 528
Part name: Disk drive
Price: $20.00
Quantity on hand: 10
Enter operation code: q
Price
$ 5.00
$ 20.00
$ 150.00
Quantity on Hand
20
10
4
cats.iku.edu.tr
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 9 images

Recommended textbooks for you
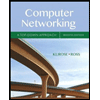
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
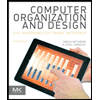
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
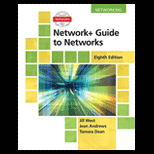
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
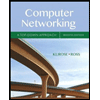
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
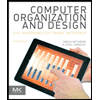
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
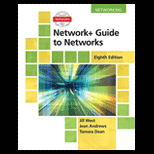
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
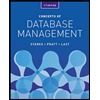
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
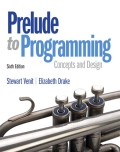
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
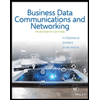
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY