lab_2_learning_python
.py
keyboard_arrow_up
School
University of Texas, Rio Grande Valley *
*We aren’t endorsed by this school
Course
2350
Subject
Civil Engineering
Date
Apr 3, 2024
Type
py
Pages
2
Uploaded by suckmyassbuddy on coursehero.com
"""
**CIVE 2350: Numerical Methods for Civil Engineers** **Lab B: Learning Python**
"""
import math
# %% Question `sort_last`
"""
# Given a list of non-empty tuples, return a list sorted in increasing
# order by the last element in each tuple.
# e.g. [(1, 7), (1, 3), (3, 4, 5), (2, 2)] yields
# [(2, 2), (1, 3), (3, 4, 5), (1, 7)]
Hint: use `sorted` with a custom key
"""
def sort_last(tup):
tup.sort(key = lambda x: x[1])
return tup
tup = [(1, 7), (1, 3), (3, 4, 5), (2, 2)]
print(sort_last(tup))
# %%
"""
Write a program which can compute the factorial of a given number using a recursive function..
Example:
Input: 8
Output: 40320
"""
def get_factorial(x):
if x == 1:
return 1
else:
return x * get_factorial(x-1)
get_factorial(8)
# %%
"""
Write a function that takes a list and returns a new list that contains a sorted list of unique elements in the first.
Example:
Input: [1, 5, 2, 3, 4, 5, 2, 3, 4]
Output: [1, 2, 3, 4, 5]
"""
def get_uniques(x):
return sorted(set(x))
get_uniques([1, 5, 2, 3, 4, 5, 2, 3, 4])
# %%
"""
Return the average of a list of numbers
Example:
Input: [5, 3, 4, 5, 2]
Output: 3.8
"""
def get_average(x):
return sum(x)/len(x)
x = [5,3,4,5,2]
get_average(x)
# %%
"""
Write a program to calculate the area of a circle. Import the "math" library to get pi
"""
def get_area_circle(radius):
import math
return math.pi*(radius**2)
get_area_circle(4)
#%%
"""
Define a class called "Vehicle".
Write the special __init__ method for the class to take the following
propoerties:
- "color"
- "max_speed_in_miles"
Add a method "get_speed_in_kilometers" to the class, that returns the speed in km by converting the value in "max_speed_in_miles"
"""
class Vehicle():
def __init__(self, color, max_speed_in_miles):
self.color = color
self.max_speed_in_miles = max_speed_in_miles
def get_speed_in_kilometers(self):
return self.max_speed_in_miles*1.609344
v1 = Vehicle(color = 'red', max_speed_in_miles = 60)
v1.get_speed_in_kilometers()
#%%
"""
Write a class for a "Bus" as a subclass (inheritance) from the class
"Vehicle".
"""
class Bus(Vehicle):
pass
b1 = Bus("white", 80)
# %%
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
The domain and range of the
* :function which has the graph
%23
is x 2 1 & y > 1 O
is x 2 2 & y > 2 O
is x 1 O
is x 2 2 & y > 1 O
is x 2 2 & y 1 O
is x > 1 & y > 1 O
arrow_forward
Write a program in MATLAB to print the Multiplication table by using for statement?
Your answer
arrow_forward
Which command in MATLAB performs
?matrix operation on elements
a' O
a^3
O
a. *b
O
a_4 O
arrow_forward
Slove the following equation using grammars
rule:
X + 2y + Z = 3
2X + y - Z = 0
X - y + Z = 0
arrow_forward
SOLVE THE PROBLEM a,b,c parts GIVEN IN IMAGE IN TYPED FORM NOT WRITTEN FORM PLEASE.
arrow_forward
04:1 Write a computer program using FORTRAN 90 to to evaluate sin(x) using the
following series by using DO loop. Hint: use format statement to print your result
Sin (x)- y²+²+ (+1)*(+1)*(+1)
arrow_forward
P2.4.6
List the given, required, graph and box the final answer
Note: 4.5 is just a reference since as stated at the problem of 4.6
arrow_forward
Numerical analysis-doing by matlab
arrow_forward
Draw Arrow and node diagram
arrow_forward
Please show computations, graph and solution thank you
arrow_forward
bläi 5 The phrase used in Matlab program to
find the sum of elements above the
*: main diagonal in matrix A is
arrow_forward
PLease answer it in Matlab
arrow_forward
Programming the MCS Method in MATLAB or any
programming language which you are familiar according to
the procedure proposed by this course.
arrow_forward
21. Domain of a function is:
a) the maximal set of numbers for which a function is defined
b) the maximal set of numbers which a function can take values
c) it is set of natural numbers for which a function is defined
d) none of the mentioned
arrow_forward
Do not roundoff the values during computation.
arrow_forward
SOLVE SHOW FORMULA AND PLS WRITE LEGIBLE
arrow_forward
this is for matlab. could you please assist me?
arrow_forward
When determining the symbolic length of x in a structure from the equation PL/EA, I am not sure how the 2nd line in the equation algabraically turns into what is in the 3rd line (3/2)x=L/2 (a screenshot is provided).
I haven't had engineering classes for 7 years, so I am a little fuzzy on some of the basics, and am just looking to see how the algebra actually gives x.
Would you be able to explain how the equation breaks down without the abbreviated answer that is given?
arrow_forward
Q1: Difference between Finite element analysis and finite element methods ?
arrow_forward
Answer number 2 only
x=1, y=5
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
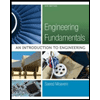
Engineering Fundamentals: An Introduction to Engi...
Civil Engineering
ISBN:9781305084766
Author:Saeed Moaveni
Publisher:Cengage Learning
Related Questions
- The domain and range of the * :function which has the graph %23 is x 2 1 & y > 1 O is x 2 2 & y > 2 O is x 1 O is x 2 2 & y > 1 O is x 2 2 & y 1 O is x > 1 & y > 1 Oarrow_forwardWrite a program in MATLAB to print the Multiplication table by using for statement? Your answerarrow_forwardWhich command in MATLAB performs ?matrix operation on elements a' O a^3 O a. *b O a_4 Oarrow_forward
- Slove the following equation using grammars rule: X + 2y + Z = 3 2X + y - Z = 0 X - y + Z = 0arrow_forwardSOLVE THE PROBLEM a,b,c parts GIVEN IN IMAGE IN TYPED FORM NOT WRITTEN FORM PLEASE.arrow_forward04:1 Write a computer program using FORTRAN 90 to to evaluate sin(x) using the following series by using DO loop. Hint: use format statement to print your result Sin (x)- y²+²+ (+1)*(+1)*(+1)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Engineering Fundamentals: An Introduction to Engi...Civil EngineeringISBN:9781305084766Author:Saeed MoaveniPublisher:Cengage Learning
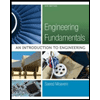
Engineering Fundamentals: An Introduction to Engi...
Civil Engineering
ISBN:9781305084766
Author:Saeed Moaveni
Publisher:Cengage Learning