12.19 LAB: Natural merge sort The merge sort algorithm recursively divides the array in half until an array with one element is reached. A variant of merge sort, called natural merge sort, instead finds already-sorted runs of elements and merges the runs together. Ex: The unsorted array below has five sorted runs. 17 58 96 24 88 70 23 64 74 81 55 A B C D E Natural merge sort starts at index 0 and finds sorted runs A and B. Runs A and B are merged, using the same merging algorithm as merge sort, to make run F. 17 24 58 88 96 70 23 64 74 81 55 F с D E Next, the algorithm starts after the merged part F, again looking for two sequential, sorted runs. Runs C and D are found and merged to make run G. 17 24 58 88 96 23 64 70 74 81 55 F E The algorithm then starts after the merged portion G. Only one run exists, run E, until the end of the array is reached. So the algorithm starts back at index 0, finds runs F and G, and merges to make run H. 17 23 24 58 64 70 74 81 88 96 55 H E Again a single run is found after the just-merged part, so the search starts back at index 0. Runs H and E are found and merged. One last search for a sorted run occurs, finding a sorted run length equal to the array's length. So the array is sorted and the algorithm terminates. 17 23 24 55 58 64 70 74 81 88 96 Step 1: Implement the getSorted RunLength() method Implement the getSorted RunLength() method in Natural MergeSorter.java. Access Natural MergeSorter.java by clicking on the orange arrow next to Natural Merge.java at the top of the coding window. getSortedRunLength() has three parameters: • array: a reference to an array of integers, ⚫ arrayLength: an integer for the array's length, and startIndex: an integer for the run's starting index. The method returns the number of array elements sorted in ascending order, starting at startIndex and ending either at the end of the sorted run, or the end of the array, whichever comes first. The method returns 0 if startIndex is out of bounds. File NaturalMerge.java has several test cases for getSorted RunLength() that can be run by clicking the "Run program" button. One test case also exists for natural MergeSort(), but that can be ignored until step two is completed. The program's output does not affect grading. Submit for grading to ensure that the getSorted RunLength() unit tests pass before proceeding. Step 2: Implement the natural MergeSort() method Implement the natural MergeSort() method in Natural MergeSorter.java. naturalMergeSort() must: 1. Start at index i=0 2. Get the length of the first sorted run, starting at i • Return if the first run's length equals the array length • If the first run ends at the array's end, reassign i=0 and repeat step 2 3. Get the length of the second sorted run, starting immediately after the first 4. Merge the two runs with the provided merge() method 5. Reassign i with the first index after the second run, or 0 if the second run ends at the array's end 6. Go to step 2
12.19 LAB: Natural merge sort The merge sort algorithm recursively divides the array in half until an array with one element is reached. A variant of merge sort, called natural merge sort, instead finds already-sorted runs of elements and merges the runs together. Ex: The unsorted array below has five sorted runs. 17 58 96 24 88 70 23 64 74 81 55 A B C D E Natural merge sort starts at index 0 and finds sorted runs A and B. Runs A and B are merged, using the same merging algorithm as merge sort, to make run F. 17 24 58 88 96 70 23 64 74 81 55 F с D E Next, the algorithm starts after the merged part F, again looking for two sequential, sorted runs. Runs C and D are found and merged to make run G. 17 24 58 88 96 23 64 70 74 81 55 F E The algorithm then starts after the merged portion G. Only one run exists, run E, until the end of the array is reached. So the algorithm starts back at index 0, finds runs F and G, and merges to make run H. 17 23 24 58 64 70 74 81 88 96 55 H E Again a single run is found after the just-merged part, so the search starts back at index 0. Runs H and E are found and merged. One last search for a sorted run occurs, finding a sorted run length equal to the array's length. So the array is sorted and the algorithm terminates. 17 23 24 55 58 64 70 74 81 88 96 Step 1: Implement the getSorted RunLength() method Implement the getSorted RunLength() method in Natural MergeSorter.java. Access Natural MergeSorter.java by clicking on the orange arrow next to Natural Merge.java at the top of the coding window. getSortedRunLength() has three parameters: • array: a reference to an array of integers, ⚫ arrayLength: an integer for the array's length, and startIndex: an integer for the run's starting index. The method returns the number of array elements sorted in ascending order, starting at startIndex and ending either at the end of the sorted run, or the end of the array, whichever comes first. The method returns 0 if startIndex is out of bounds. File NaturalMerge.java has several test cases for getSorted RunLength() that can be run by clicking the "Run program" button. One test case also exists for natural MergeSort(), but that can be ignored until step two is completed. The program's output does not affect grading. Submit for grading to ensure that the getSorted RunLength() unit tests pass before proceeding. Step 2: Implement the natural MergeSort() method Implement the natural MergeSort() method in Natural MergeSorter.java. naturalMergeSort() must: 1. Start at index i=0 2. Get the length of the first sorted run, starting at i • Return if the first run's length equals the array length • If the first run ends at the array's end, reassign i=0 and repeat step 2 3. Get the length of the second sorted run, starting immediately after the first 4. Merge the two runs with the provided merge() method 5. Reassign i with the first index after the second run, or 0 if the second run ends at the array's end 6. Go to step 2
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write the full Java code for NaturalMergeSorter.java

Transcribed Image Text:12.19 LAB: Natural merge sort
The merge sort algorithm recursively divides the array in half until an array with one element is reached. A variant of merge
sort, called natural merge sort, instead finds already-sorted runs of elements and merges the runs together.
Ex: The unsorted array below has five sorted runs.
17
58 96 24 88 70 23 64 74
81
55
A
B
C
D
E
Natural merge sort starts at index 0 and finds sorted runs A and B. Runs A and B are merged, using the same merging
algorithm as merge sort, to make run F.
17 24 58
88 96 70 23
64 74 81
55
F
с
D
E
Next, the algorithm starts after the merged part F, again looking for two sequential, sorted runs. Runs C and D are found and
merged to make run G.
17 24 58 88
96 23 64
70 74 81 55
F
E
The algorithm then starts after the merged portion G. Only one run exists, run E, until the end of the array is reached. So the
algorithm starts back at index 0, finds runs F and G, and merges to make run H.
17 23 24 58
64 70 74 81 88
96
55
H
E
Again a single run is found after the just-merged part, so the search starts back at index 0. Runs H and E are found and
merged.
One last search for a sorted run occurs, finding a sorted run length equal to the array's length. So the array is sorted and the
algorithm terminates.
17 23 24 55 58 64 70 74 81 88 96
Step 1: Implement the getSorted RunLength() method
Implement the getSorted RunLength() method in Natural MergeSorter.java. Access Natural MergeSorter.java by clicking on
the orange arrow next to Natural Merge.java at the top of the coding window.
getSortedRunLength() has three parameters:
•
array: a reference to an array of integers,
⚫ arrayLength: an integer for the array's length, and
startIndex: an integer for the run's starting index.
The method returns the number of array elements sorted in ascending order, starting at startIndex and ending either at the
end of the sorted run, or the end of the array, whichever comes first. The method returns 0 if startIndex is out of bounds.
File NaturalMerge.java has several test cases for getSorted RunLength() that can be run by clicking the "Run program"
button. One test case also exists for natural MergeSort(), but that can be ignored until step two is completed.
The program's output does not affect grading.
Submit for grading to ensure that the getSorted RunLength() unit tests pass before proceeding.
Step 2: Implement the natural MergeSort() method
Implement the natural MergeSort() method in Natural MergeSorter.java. naturalMergeSort() must:
1. Start at index i=0
2. Get the length of the first sorted run, starting at i
• Return if the first run's length equals the array length
• If the first run ends at the array's end, reassign i=0 and repeat step 2
3. Get the length of the second sorted run, starting immediately after the first
4. Merge the two runs with the provided merge() method
5. Reassign i with the first index after the second run, or 0 if the second run ends at the array's end
6. Go to step 2
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Similar questions
Recommended textbooks for you
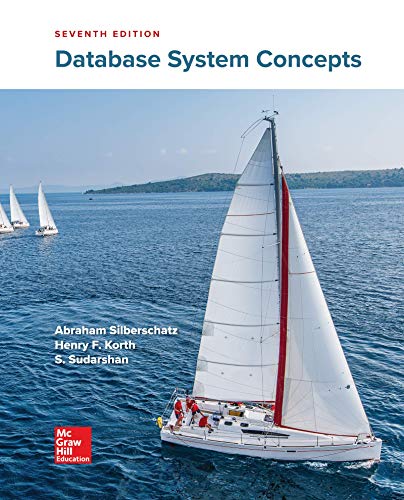
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
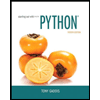
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
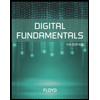
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
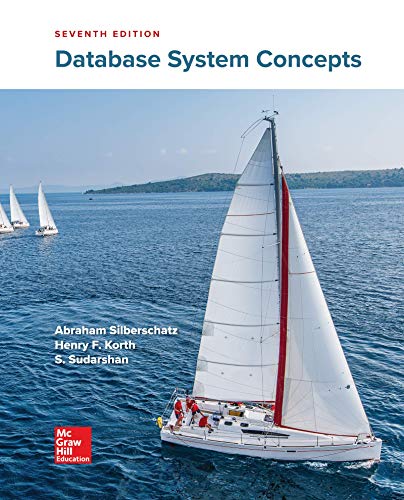
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
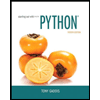
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
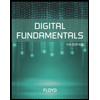
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
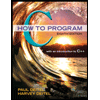
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
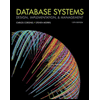
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
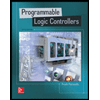
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education