Change value of isMomHappy to true and run the code. Provide your source code and the output var isMomHappy = false; //promise var willIGetNewPhone = new Promise( function (resolve, reject) { if(isMomHappy){ var phone = { brand: 'samsung', color:'black' }; resolve(phone); } else { var reason = new Error('mom is not happy'); reject(reason); } } ); //call our promise var askMom = function() { willIGetNewPhone .then(function (fulfilled){ //yay, you got a new phone console.log(fulfilled); //output: {brand: 'sumsung', color: 'black' } }) .catch(function (error) { //oops, mom didnt buy it console.log(error.message); //output: 'mom is not happy' }); }; askMom();
Change value of isMomHappy to true and run the code. Provide your source code and the output var isMomHappy = false; //promise var willIGetNewPhone = new Promise( function (resolve, reject) { if(isMomHappy){ var phone = { brand: 'samsung', color:'black' }; resolve(phone); } else { var reason = new Error('mom is not happy'); reject(reason); } } ); //call our promise var askMom = function() { willIGetNewPhone .then(function (fulfilled){ //yay, you got a new phone console.log(fulfilled); //output: {brand: 'sumsung', color: 'black' } }) .catch(function (error) { //oops, mom didnt buy it console.log(error.message); //output: 'mom is not happy' }); }; askMom();
Programming with Microsoft Visual Basic 2017
8th Edition
ISBN:9781337102124
Author:Diane Zak
Publisher:Diane Zak
Chapter10: Classes And Objects
Section: Chapter Questions
Problem 17RQ
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
Change value of isMomHappy to true and run the code. Provide your source code and the output
var isMomHappy = false;
//promise
var willIGetNewPhone = new Promise(
function (resolve, reject) {
if(isMomHappy){
var phone = {
brand: 'samsung',
color:'black'
};
resolve(phone);
} else {
var reason = new Error('mom is not happy');
reject(reason);
}
}
);
//call our promise
var askMom = function() {
willIGetNewPhone
.then(function (fulfilled){
//yay, you got a new phone
console.log(fulfilled);
//output: {brand: 'sumsung', color: 'black' }
})
.catch(function (error) {
//oops, mom didnt buy it
console.log(error.message);
//output: 'mom is not happy'
});
};
askMom();

Transcribed Image Text:10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
function (resolve, reject) (
if (isMomHappy) {
var phone
{
};
}
} else {
brand: "Samsung",
color: black"
}
);
// call our promise
};
resolve (phone); // fulfilled
var askMom - function () {
willIGetNewPhone
})
var reason = new Error('mom is not happy');
reject(reason); // reject
.then(function (fulfilled) (
// yay, you got a new phone
console.log(fulfilled);
});
askMon();
.catch(function (error) {
// output: { brand: "Samsung", color: "black" }
// oops, mom didn't buy it
console.log(error.message);
| // output: 'mom is not happy'
This code returns:
mom is not happy and the person will not get the promised phone.
Change value of isMom Happy to true and run the code. Provide your source code and the output
2.5.2. Async/await
Most recent additions to the JavaScript language are async functions and the await keyword. These
22

Transcribed Image Text:})
.catch(failureCallback);
When a promise is created, it is neither in a success nor in a failure state. It is said to be pending. When
a promise returns, it is said to be resolved. A successfully resolved promise is said to be fulfilled. It
returns a value, which can be accessed by chaining a .then() block onto the end of the promise chain.
The callback function inside the .then() block will contain the promise's return value.
An unsuccessful resolved promise is said to be rejected. It returns a reason, an error message stating
why the promise was rejected. This reason can be accessed by chaining a .catch() block onto the end
of the promise chain.
Create a promises.js file and add the following code there:
chapter08>
promises.js>...
1 var isMomHappy = false;
2
3
5
9
10
11
12
===26=92====**========
13
14
15
16
17
18
19
20
21
23
24
25
27
28
30
31
33
// Promise
var willIGetNewPhone new Promise(
function (resolve, reject) {
if (isMomHappy) {
var phone
{
32 };
34
}
}
resolve(phone); // fulfilled
} else {
var reason = new Error('mom is not happy');
reject(reason); // reject
);
// call our promise
var askMom - function () {
williGetNewPhone
}}
})
brand: "Samsung",
color: 'black'
.then(function (fulfilled) {
});
askMon();
.catch(function (error) {
// yay, you got a new phone
console.log(fulfilled);
// output: { brand: "Samsung", color: "black" }
// oops, mon didn't buy it
console.log(error.message);
// output: 'mom is not happy'
22
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
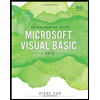
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
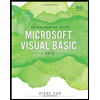
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning