Check this code for this assignment: It runs successfully on Netbeans IDE but there is no output. // Create a Scanner object to read user input Scanner input = new Scanner(System.in); // Prompt the user to enter the street number System.out.print("Enter street number: "); int streetNumber = input.nextInt(); input.nextLine(); // Prompt the user to enter the street name System.out.print("Enter street name: "); String streetName = input.nextLine(); // Prompt the user to enter the number of rooms in the house System.out.print("Enter number of rooms: "); int numRooms = input.nextInt(); input.nextLine(); // Create an array to store the room types String[] roomTypes = { "living", "dining", "bedroom1", "bedroom2", "kitchen", "bathroom" }; // Create an array to store the area of each room int[] roomAreas = new int[6]; // Prompt the user to enter the area of each room for (int i = 0; i < 6; i++) { System.out.print("Enter area of " + roomTypes[i] + " room: "); roomAreas[i] = input.nextInt(); input.nextLine(); } // Prompt the user to enter the price per sq. ft. System.out.print("Enter price per sq. ft.: "); double pricePerSqFt = input.nextDouble(); // Calculate the total area of the house int totalArea = 0; for (int i = 0; i < 6; i++) { totalArea += roomAreas[i]; } // Calculate the estimated property value double estimatedValue = totalArea * pricePerSqFt; // Display the results System.out.println("\n1. Street: " + streetName + " #" + streetNumber); System.out.print("2. Total Rooms: " + numRooms + " ("); for (int i = 0; i < 6; i++) { System.out.print(roomTypes[i]); if (i < 5) { System.out.print(", "); } } System.out.println(")"); System.out.println("3. Total Area: " + totalArea + " sq. ft."); System.out.println("4. Price per sq. ft: $" + pricePerSqFt); System.out.println("5. Estimated property value: $" + estimatedValue); } }
Check this code for this assignment: It runs successfully on Netbeans IDE but there is no output.
// Create a Scanner object to read user input
Scanner input = new Scanner(System.in);
// Prompt the user to enter the street number
System.out.print("Enter street number: ");
int streetNumber = input.nextInt();
input.nextLine();
// Prompt the user to enter the street name
System.out.print("Enter street name: ");
String streetName = input.nextLine();
// Prompt the user to enter the number of rooms in the house
System.out.print("Enter number of rooms: ");
int numRooms = input.nextInt();
input.nextLine();
// Create an array to store the room types
String[] roomTypes = { "living", "dining", "bedroom1", "bedroom2", "kitchen", "bathroom" };
// Create an array to store the area of each room
int[] roomAreas = new int[6];
// Prompt the user to enter the area of each room
for (int i = 0; i < 6; i++) {
System.out.print("Enter area of " + roomTypes[i] + " room: ");
roomAreas[i] = input.nextInt();
input.nextLine();
}
// Prompt the user to enter the price per sq. ft.
System.out.print("Enter price per sq. ft.: ");
double pricePerSqFt = input.nextDouble();
// Calculate the total area of the house
int totalArea = 0;
for (int i = 0; i < 6; i++) {
totalArea += roomAreas[i];
}
// Calculate the estimated property value
double estimatedValue = totalArea * pricePerSqFt;
// Display the results
System.out.println("\n1. Street: " + streetName + " #" + streetNumber);
System.out.print("2. Total Rooms: " + numRooms + " (");
for (int i = 0; i < 6; i++) {
System.out.print(roomTypes[i]);
if (i < 5) {
System.out.print(", ");
}
}
System.out.println(")");
System.out.println("3. Total Area: " + totalArea + " sq. ft.");
System.out.println("4. Price per sq. ft: $" + pricePerSqFt);
System.out.println("5. Estimated property value: $" + estimatedValue);
}
}
![Write a program to calculate an estimation of a real estate property value.
1. Declare variables to hold the data fields for:
■ Street number
■ Street name
▪ The number of rooms in the house
▪
Five string variables for types of rooms: (living, dining, bedroom1, bedroom2, kitchen,
bathroom, etc.)
▪ Five integer variables for the area of each room in sq. ft.
▪ Price per sq. ft., for example, $150.50 (store this value as double.)
2. Prompt the user for each of the above fields. Read console input and store entered values in
the corresponding variables.
IMPORTANT: Make sure that the street name can be entered with embedded spaces, for
example, "Washington St" and "Park Ave" are both valid street names.
3. Compute total area of the house (total sq. ft.) and multiply it by the Price per sq. ft. to
compute the estimated property value.
4. Display the results in the following format. IMPORTANT: Your program should keep the
line count in a separate variable and print the line numbers in your report using that variable
as shown:
1.
Street:
2.
Total Rooms:
Total Area:
3.
4.
Price per sq. ft: $
5. Estimated property value: $
Coding Requirements
Always have a block comment on top of the program describing assignment number, author,
and what it does.
(List entered rooms here)
sq. ft.
Label the end of your class and the end of the main method with appropriate "ending"
comments:
public class your class name goes here
{
}//main
public static void main(String[] args)
{
}//class your class name goes here
Maintain your coding standard:
o Align verically each opening and closing brace { }
o Indent program statements
• Use appropriate variable naming convention
Add comments where necessary.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1c50bd72-23cb-4139-953a-0cc18f92fc0e%2F843a892f-392f-4f24-85ec-d90384810a87%2F1dpkomd_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

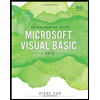
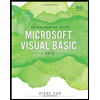