complete the Java code based on the template below.
Enhanced Discovering Computers 2017 (Shelly Cashman Series) (MindTap Course List)
1st Edition
ISBN:9781305657458
Author:Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. Campbell
Publisher:Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. Campbell
Chapter4: Programs And Apps: Productivity, Graphics, Security, And Other Tools
Section: Chapter Questions
Problem 2IR
Related questions
Question
complete the Java code based on the template below. The images attached is the project description and sample!
Quiz Tree code template:
import java.io.PrintStream;
import java.util.Scanner;
public class QuizTree {
QuizTreeNode root;
public QuizTree(Scanner inputFile) {
root = read(inputFile, root);
}
private QuizTreeNode read(Scanner input, QuizTreeNode node) {
if (!input.hasNext()) return node;
String line = input.nextLine();
/**
* 1. set node to a new node
* 2. check if the line is the leaf => return the node
* 3. otherwise, set node left = read (input, node left)
* set node right = read(input, node right)
* return node
*/
return node;
}
public void takeQuiz(Scanner console) {
takeQuiz(console, root);
}
private void takeQuiz(Scanner console, QuizTreeNode node) {
/**
* if the node is a leaf (both children are null) => display the value and return
* Split the node value into two values (e.g. blue and green)
* prompt the user using nodes' value
* get the response from the user and determine is that left or right
* if the response equals left call takeQuiz(console, node.left)
* else if the response equals right call takeQuiz (console, node.right)
* else prompt the user 'Invalid response; try again.'
*/
}
public void export() {
export(root);
}
private void export(QuizTreeNode node) {
/**
* display the bst data to the console using System.out.println()
* if node is null return
* if node is a leaf print "END:" + leaf value
* else print leaf value
* call export(node left)
* call export(node right)
*/
}
public void addQuestion(String toReplace, String leftChoice, String rightChoice, String leftResult, String rightResult) {
/**
* 1. use the search function to search for the item
* 2. Concat the left choice and right choice into a single new value
* 3. update the node value (toReplace) with the new value
* 4. Add a left node with information from the leftResult
* 5. Add a right node with the information from the rightResult
*/
}
public QuizTreeNode search(String value) {
return search(value, root);
}
private QuizTreeNode search(String value, QuizTreeNode node) {
/**
* if (node is null ) return null;
* if (node value is the same as the value)
* node.left = call itself (value, node.left)
* node.right = call itself (value, node.right);
*/
return node;
}
static class QuizTreeNode {
public String value;
QuizTreeNode left;
QuizTreeNode right;
public QuizTreeNode(String value) {
if (value.startsWith("END:")) {
value = value.substring("END:".length());
}
this.value = value;
}
public boolean isLeaf() { return left == null && right == null; }
@Override
public String toString() {
return "QuizTreeNode{" +
"value='" + value + '\'' +
", left=" + left +
", right=" + right +
'}';
}
}
}
import java.util.Scanner;
public class QuizTree {
QuizTreeNode root;
public QuizTree(Scanner inputFile) {
root = read(inputFile, root);
}
private QuizTreeNode read(Scanner input, QuizTreeNode node) {
if (!input.hasNext()) return node;
String line = input.nextLine();
/**
* 1. set node to a new node
* 2. check if the line is the leaf => return the node
* 3. otherwise, set node left = read (input, node left)
* set node right = read(input, node right)
* return node
*/
return node;
}
public void takeQuiz(Scanner console) {
takeQuiz(console, root);
}
private void takeQuiz(Scanner console, QuizTreeNode node) {
/**
* if the node is a leaf (both children are null) => display the value and return
* Split the node value into two values (e.g. blue and green)
* prompt the user using nodes' value
* get the response from the user and determine is that left or right
* if the response equals left call takeQuiz(console, node.left)
* else if the response equals right call takeQuiz (console, node.right)
* else prompt the user 'Invalid response; try again.'
*/
}
public void export() {
export(root);
}
private void export(QuizTreeNode node) {
/**
* display the bst data to the console using System.out.println()
* if node is null return
* if node is a leaf print "END:" + leaf value
* else print leaf value
* call export(node left)
* call export(node right)
*/
}
public void addQuestion(String toReplace, String leftChoice, String rightChoice, String leftResult, String rightResult) {
/**
* 1. use the search function to search for the item
* 2. Concat the left choice and right choice into a single new value
* 3. update the node value (toReplace) with the new value
* 4. Add a left node with information from the leftResult
* 5. Add a right node with the information from the rightResult
*/
}
public QuizTreeNode search(String value) {
return search(value, root);
}
private QuizTreeNode search(String value, QuizTreeNode node) {
/**
* if (node is null ) return null;
* if (node value is the same as the value)
* node.left = call itself (value, node.left)
* node.right = call itself (value, node.right);
*/
return node;
}
static class QuizTreeNode {
public String value;
QuizTreeNode left;
QuizTreeNode right;
public QuizTreeNode(String value) {
if (value.startsWith("END:")) {
value = value.substring("END:".length());
}
this.value = value;
}
public boolean isLeaf() { return left == null && right == null; }
@Override
public String toString() {
return "QuizTreeNode{" +
"value='" + value + '\'' +
", left=" + left +
", right=" + right +
'}';
}
}
}
Quiz Client Code:
import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintStream;
import java.util.Scanner;
public class QuizClient {
public static void main(String[] args) throws FileNotFoundException {
Scanner console = new Scanner(System.in);
System.out.print("Enter quiz file to read: ");
String inFileName = console.nextLine();
File inFile = new File(inFileName);
while (!inFile.exists()) {
System.out.println(" File does not exist. Please try again.");
System.out.print("Enter quiz file to read: ");
inFileName = console.nextLine();
inFile = new File(inFileName);
}
QuizTree quiz = new QuizTree(new Scanner(inFile));
System.out.println("Quiz created!");
System.out.println();
String option = "";
while (!option.equalsIgnoreCase("quit")) {
option = menu(console);
System.out.println();
if (option.equalsIgnoreCase("take")) {
quiz.takeQuiz(console);
System.out.println();
} else if (option.equalsIgnoreCase("add")) {
addQ(console, quiz);
} else if (option.equalsIgnoreCase("export")) {
quiz.export();
System.out.println("Quiz exported!");
System.out.println();
} else if (!option.equalsIgnoreCase("quit")) {
System.out.println(" Invalid choice. Please try again.");
}
}
}
private static String menu(Scanner console) {
System.out.println("What would you like to do? Choose an option in brackets.");
System.out.println(" [take] quiz");
System.out.println(" [add] question");
System.out.println(" [export] quiz");
System.out.println(" [quit] program");
return console.nextLine();
}
private static void addQ(Scanner console, QuizTree quiz) {
System.out.print("Enter result to replace: ");
String toReplace = console.nextLine();
System.out.print("Enter left choice: ");
String leftChoice = console.nextLine();
System.out.print("Enter right choice: ");
String rightChoice = console.nextLine();
System.out.print("Enter left result: ");
String leftResult = console.nextLine();
System.out.print("Enter right result: ");
String rightResult = console.nextLine();
quiz.addQuestion(toReplace, leftChoice, rightChoice, leftResult, rightResult);
}
}
import java.io.FileNotFoundException;
import java.io.PrintStream;
import java.util.Scanner;
public class QuizClient {
public static void main(String[] args) throws FileNotFoundException {
Scanner console = new Scanner(System.in);
System.out.print("Enter quiz file to read: ");
String inFileName = console.nextLine();
File inFile = new File(inFileName);
while (!inFile.exists()) {
System.out.println(" File does not exist. Please try again.");
System.out.print("Enter quiz file to read: ");
inFileName = console.nextLine();
inFile = new File(inFileName);
}
QuizTree quiz = new QuizTree(new Scanner(inFile));
System.out.println("Quiz created!");
System.out.println();
String option = "";
while (!option.equalsIgnoreCase("quit")) {
option = menu(console);
System.out.println();
if (option.equalsIgnoreCase("take")) {
quiz.takeQuiz(console);
System.out.println();
} else if (option.equalsIgnoreCase("add")) {
addQ(console, quiz);
} else if (option.equalsIgnoreCase("export")) {
quiz.export();
System.out.println("Quiz exported!");
System.out.println();
} else if (!option.equalsIgnoreCase("quit")) {
System.out.println(" Invalid choice. Please try again.");
}
}
}
private static String menu(Scanner console) {
System.out.println("What would you like to do? Choose an option in brackets.");
System.out.println(" [take] quiz");
System.out.println(" [add] question");
System.out.println(" [export] quiz");
System.out.println(" [quit] program");
return console.nextLine();
}
private static void addQ(Scanner console, QuizTree quiz) {
System.out.print("Enter result to replace: ");
String toReplace = console.nextLine();
System.out.print("Enter left choice: ");
String leftChoice = console.nextLine();
System.out.print("Enter right choice: ");
String rightChoice = console.nextLine();
System.out.print("Enter left result: ");
String leftResult = console.nextLine();
System.out.print("Enter right result: ");
String rightResult = console.nextLine();
quiz.addQuestion(toReplace, leftChoice, rightChoice, leftResult, rightResult);
}
}

Transcribed Image Text:o You may also not create a single base class or interface that two separate classes
extend or implement. All nodes in the tree must be instances of the same class.
Extension: Modifying Quizzes
To earn an E on this assignment, you must also implement the following additional methods in your
QuizTree:
public void export (PrintStream outputFile)
Print the current quiz to the provided output file. See above for the expected file format.
public void addQuestion(String toReplace, String leftChoice, String rightChoice, String
leftResult, String rightResult)
• Replace the node for the result toReplace with a new node representing a choice be-
tween leftChoice and rightChoice leading to left Result and rightResult respectively.
o If toReplace is not a possible result in the quiz, including if it is a choice rather
than a result, throw
IllegalArgumentException.
o You do not need to support replacing choice nodes; only results.
Tree Representation of Modified Quiz
Notice that the result Froot Loops is no longer available in the quiz. In its place is now a new choice
node choosing between gold and silver, which produce the results Cheerios and Frosted Mini-
Wheats respectively.
Sample Executions - Taking Quiz
Here are a few sample executions of taking the sample quiz above. User input is bold.
Do you prefer red or blue? **red**
Do you prefer yellow or green? **green**
Your result is: Raisin Bran
Do you prefer red or blue? **blue**
Do you prefer purple or orange? **purple**
Your result is: Frosted Flakes
Do you prefer red or blue? **blue**
Do you prefer purple or orange? **orange**
Do you prefer black or white? **black**
Your result is: Rice Krispies
Do you prefer red or blue? **green**
Invalid response; try again.
Do you prefer red or blue? **white**
Invalid response; try again.
Do you prefer red or blue? **neither!!!**
Invalid response; try again.
Do you prefer red or blue? **Red**
Do you prefer yellow or green? **YELLOW**
Your result is: Froot Loops
Notice in the last example that when the user types an invalid option, they should be informed their
response was not valid and prompted again. Notice also that options should be case-insensitive.
(For example, the program accepted Red instead of red).
Sample Output - Modifying Quiz
Suppose the sample quiz from above were stored in a QuizTree called cereals, and the following call
were made:
cereals.addQuestion("Froot Loops", "gold", "silver", "Cheerios", "Frosted Mini-Wheats");
This would result in the following quiz:
red/blue

Transcribed Image Text:Background
The website BuzzFeed, now a media and news outlet, came to popularity on social media in
part due to its quizzes. On these simple, interactive websites, users are presented with a series
of choices or questions to respond to, after which they are given some sort of result-- a score,
categorization, or recommendation, among other options. This format has been emulated on many
other entertainment and social media platforms. (Of note, BuzzFeed did not invent this format, but
is likely responsible for popularizing the types of quizzes currently prevalent on social media.)
In this assignment, you will implement a version of a BuzzFeed-style quiz that we have named
"BrettFeed"
System Structure
In our quizzes, users will be asked repeatedly to choose which of two options they prefer until they
are presented with a final result. We will represent a quiz using a binary tree, where leaf nodes
represent possible results, and non-leaf nodes (branches) represent choices the user will make.
When a user takes a quiz, they will be presented with the choice from the root node of the tree.
Based on their response, the system will traverse to either the left or right child of the root. If the
node found is a leaf, the user will be shown their result. Otherwise, the process will repeat from the
new node until a leaf is reached. See below for a full sample quiz and execution.
Quiz File Format
In addition to representing quizzes as a binary tree in our program, we will also read quizzes from
and store quizzes to text files in a standard file format. In a quiz file, each node will be represented
by a single line in the file containing the text for that node.
• "Choice" nodes (i.e. nodes that represent a choice between two options) will be written
with the two choices separated by a single slash (/) character. When taking the quiz,
choosing the option before the slash will move to the left child of the node, whereas
choosing the option after the slash will move the right child of the node.
o For example, red/blue represents a choice between red and blue, where red is
the "left" choice and blue is the "right" choice.
o You may assume that no choice will contain a slash.
• "Result" nodes will be written as the result option prefixed with the text END:.
o For example, END: froot loops represents a result node for the result "froot
loops"
o You may assume that no result will contain the exact text END:.
Required Class
You will implement a class called QuizTree to represent a quiz as a binary tree. To earn an S on this
assignment you must implement the following methods in your QuizTree class:
public QuizTree (Scanner inputFile)
• Constructs a new quiz based on the provided input. See above for the expected file format.
• You may assume the provided input is in the correct format.
public void takeQuiz (Scanner console)
• Allows the user to take the current quiz using the provided Scanner. This method should
prompt the user to choose between the options at each node and traverse the tree until
a leaf node is reached. When a leaf is reached, the user's result should be printed. See
below for example output.
Quiz TreeNode class
As part of writing your QuizTree class, you should also create a public static inner
class called Quiz TreeNode to represent the nodes of the tree. The contents of this class are up to
you, but must meet the following requirements:
• The fields of the QuizTreeNode class must be public.
• The QuizTreeNode class must not contain any constructors or methods that are not used by
the QuizTree class.
• The QuizTreeNode class must not contain any logic necessary to take a quiz-- it should
purely represent a node in the tree.
• You must have a single QuizTreeNode class that can represent both choices and results-- you
should not create separate classes for the different types of nodes.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
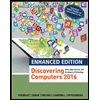
Enhanced Discovering Computers 2017 (Shelly Cashm…
Computer Science
ISBN:
9781305657458
Author:
Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. Campbell
Publisher:
Cengage Learning
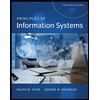
Principles of Information Systems (MindTap Course…
Computer Science
ISBN:
9781305971776
Author:
Ralph Stair, George Reynolds
Publisher:
Cengage Learning
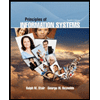
Principles of Information Systems (MindTap Course…
Computer Science
ISBN:
9781285867168
Author:
Ralph Stair, George Reynolds
Publisher:
Cengage Learning
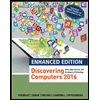
Enhanced Discovering Computers 2017 (Shelly Cashm…
Computer Science
ISBN:
9781305657458
Author:
Misty E. Vermaat, Susan L. Sebok, Steven M. Freund, Mark Frydenberg, Jennifer T. Campbell
Publisher:
Cengage Learning
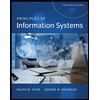
Principles of Information Systems (MindTap Course…
Computer Science
ISBN:
9781305971776
Author:
Ralph Stair, George Reynolds
Publisher:
Cengage Learning
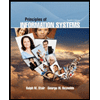
Principles of Information Systems (MindTap Course…
Computer Science
ISBN:
9781285867168
Author:
Ralph Stair, George Reynolds
Publisher:
Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L