Computer Science Create UML Class Diagrams for the 2 following java classes which will be used in a connect X game. GameScreen.java This will be the class that contains the main() method and controls the game's flow. It will alternate the game between players, say whose turn it is, get the column they would like, and place their marker. If the player chooses a location that is not available (either because the column is full or because it is outside the bounds of the 6 x 9 board), then the program will print a message saying the space is unavailable and ask them to enter a new column. Once a placement has been made, it will check to see if either player has won. If so, it will print off a congratulatory message, and ask if they would like to play again and prompt them for a response of "Y" or "N". If they choose to play again, it should present them with a blank board and start the game over. If a player has not won, it will check to see if the game has ended in a tie (no spaces left on the board). If the game has ended in a tie, it will print a message saying so and ask if the player would like to play again. If the game has not ended in a win or a tie, print the current board to the screen and prompt the next player for their next move. When asking a player for their move, you can assume the user will enter an integer value for the Column. You cannot assume that they will enter a value in range (they could enter 15 or 21). Player X should go first in every game. This class has the least amount of specific requirements. You may design your class and add any private helper methods that you believe may help you. All input and output to the screen must happen in only this class. BoardPosition.java This class will be used to keep track of an individual cell for a board. BoardPosition will have variables to represent the Row position and the Column position. There will only be one constructor for the class, which will take in an int for the Row position and an int for the Column position. After the constructor has been called, there will be no other way to change any fields through any setter methods. Other methods that will be necessary: public int getRow(){ //returns the row } public int getColumn(){ //returns the column } You must also override the equals() method inherited from the Object class. Two BoardPositions are equal if they have the same row and column. You should override the toString() method to create a string in the following format ",." Example "3,5". You won't call these in your code, but it is a best practice to override them and provide a meaningful implementation. No other methods are necessary, and no other methods should be provided.
Computer Science
Create UML Class Diagrams for the 2 following java classes which will be used in a connect X game.
GameScreen.java
This will be the class that contains the main() method and controls the game's flow. It will
alternate the game between players, say whose turn it is, get the column they would like, and place
their marker. If the player chooses a location that is not available (either because the column is full or
because it is outside the bounds of the 6 x 9 board), then the
space is unavailable and ask them to enter a new column. Once a placement has been made, it will
check to see if either player has won. If so, it will print off a congratulatory message, and ask if they
would like to play again and prompt them for a response of "Y" or "N". If they choose to play again, it
should present them with a blank board and start the game over. If a player has not won, it will check to
see if the game has ended in a tie (no spaces left on the board). If the game has ended in a tie, it will
print a message saying so and ask if the player would like to play again. If the game has not ended in a
win or a tie, print the current board to the screen and prompt the next player for their next move.
When asking a player for their move, you can assume the user will enter an integer value for the
Column. You cannot assume that they will enter a value in range (they could enter 15 or 21). Player X
should go first in every game.
This class has the least amount of specific requirements. You may design your class and add any
private helper methods that you believe may help you. All input and output to the screen must happen
in only this class.
BoardPosition.java
This class will be used to keep track of an individual cell for a board. BoardPosition will
have variables to represent the Row position and the Column position.
There will only be one constructor for the class, which will take in an int for the Row position
and an int for the Column position. After the constructor has been called, there will be no other way
to change any fields through any setter methods.
Other methods that will be necessary:
public int getRow(){
//returns the row
}
public int getColumn(){
//returns the column
}
You must also override the equals() method inherited from the Object class. Two
BoardPositions are equal if they have the same row and column. You should override the
toString() method to create a string in the following format "<row>,<column>." Example "3,5". You
won't call these in your code, but it is a best practice to override them and provide a meaningful
implementation.
No other methods are necessary, and no other methods should be provided.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

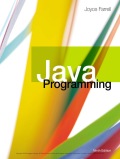
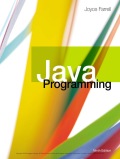