Consider the insertion sort algorithm, which sorts an array `A` of length `N` in ascending order. Below is a partial implementation of the insertion sort algorithm in Python: python function insertion_sort(A[O...N-1]) if N > 1: for i in range(1, N): index = i for j in range(i - 1, -1, -1): if A[index] < A[j]: A[index], A[j] = A[j], A[index] index = j else: break Copy code a. Complete the implementation of the 'insertion_sort function in Python, ensuring that it correctly sorts the input array `A` in ascending order. b. Explain the purpose of each loop and conditional statement within the insertion sort algorithm. c. Test your implementation with different input arrays and verify that it produces the expected output. d. Analyze the time complexity of your 'insertion_sort' implementation. Provide a step-by-step explanation of how you determined the time cplexity and discuss its implications.
Consider the insertion sort algorithm, which sorts an array `A` of length `N` in ascending order. Below is a partial implementation of the insertion sort algorithm in Python: python function insertion_sort(A[O...N-1]) if N > 1: for i in range(1, N): index = i for j in range(i - 1, -1, -1): if A[index] < A[j]: A[index], A[j] = A[j], A[index] index = j else: break Copy code a. Complete the implementation of the 'insertion_sort function in Python, ensuring that it correctly sorts the input array `A` in ascending order. b. Explain the purpose of each loop and conditional statement within the insertion sort algorithm. c. Test your implementation with different input arrays and verify that it produces the expected output. d. Analyze the time complexity of your 'insertion_sort' implementation. Provide a step-by-step explanation of how you determined the time cplexity and discuss its implications.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Question
This is not a test question. This is a pratice test.
![Consider the insertion sort algorithm, which sorts an array `A` of length `N` in ascending order. Below
is a partial implementation of the insertion sort algorithm in Python:
python
function insertion_sort(A[O...N-1])
if N > 1:
for i in range(1, N):
index = i
for j in range(i - 1, -1, -1):
if A[index] < A[j]:
A[index], A[j] = A[j], A[index]
index = j
else:
break
Copy code
a. Complete the implementation of the 'insertion_sort function in Python, ensuring that it correctly
sorts the input array `A` in ascending order.
b. Explain the purpose of each loop and conditional statement within the insertion sort algorithm.
c. Test your implementation with different input arrays and verify that it produces the expected output.
d. Analyze the time complexity of your 'insertion_sort' implementation. Provide a step-by-step
explanation of how you determined the time cplexity and discuss its implications.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0e02c446-9367-41d9-9f9b-9aac6cb957ab%2F85e687fe-c133-41b1-af67-9ffd0479a1c3%2Fg4y3c1_processed.png&w=3840&q=75)
Transcribed Image Text:Consider the insertion sort algorithm, which sorts an array `A` of length `N` in ascending order. Below
is a partial implementation of the insertion sort algorithm in Python:
python
function insertion_sort(A[O...N-1])
if N > 1:
for i in range(1, N):
index = i
for j in range(i - 1, -1, -1):
if A[index] < A[j]:
A[index], A[j] = A[j], A[index]
index = j
else:
break
Copy code
a. Complete the implementation of the 'insertion_sort function in Python, ensuring that it correctly
sorts the input array `A` in ascending order.
b. Explain the purpose of each loop and conditional statement within the insertion sort algorithm.
c. Test your implementation with different input arrays and verify that it produces the expected output.
d. Analyze the time complexity of your 'insertion_sort' implementation. Provide a step-by-step
explanation of how you determined the time cplexity and discuss its implications.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
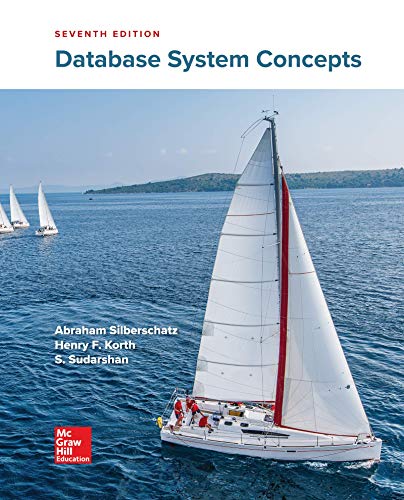
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
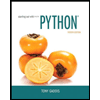
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
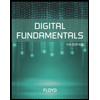
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
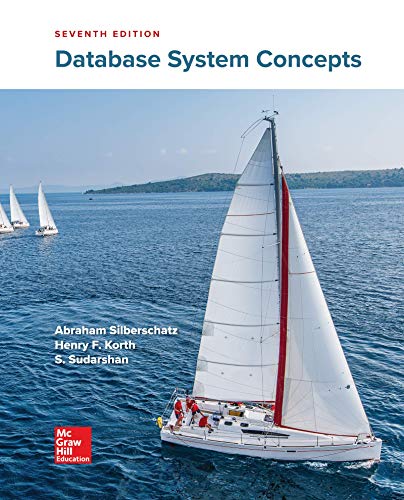
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
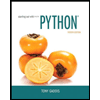
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
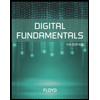
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
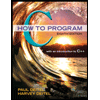
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
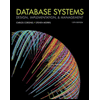
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
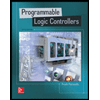
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education