create python algorithm of an operating system scheduler that is able to perform well in a variety of metrics, such as turnaround time, response time, burst time and switching time. You need to create a scheduler that is able to perform well in a variety of situations, and also needs to take into account I/O Interrupts. How you handle the processing is up to you.
create python algorithm of an operating system scheduler that is able to perform well in a variety of metrics, such as turnaround time, response time, burst time and switching time. You need to create a scheduler that is able to perform well in a variety of situations, and also needs to take into account I/O Interrupts. How you handle the processing is up to you.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
create python algorithm of an operating system scheduler that is able to perform well in a variety
of metrics, such as turnaround time, response time, burst time and switching time. You need to create a
scheduler that is able to perform well in a variety of situations, and also needs to take into account I/O Interrupts.
How you handle the processing is up to you.

Transcribed Image Text:3.1 Input
The input is a number n, indicating the number of processes in the file, followed by n processes, each on a new
line. Each process line is in the format:
Process Name, Process Runtime, Process Arrival Time, 10 Frequency
3.2 Output
Your program will need to save its output to a text file with the name schedulername_out_n.txt where n is
the number of processes processed (should match the input file). Inside the text file you should have 1 line
representing the order of the processes as they were scheduled, separated by a space. If IO occurs for a given
process, then it needs to be indicated by a followed by the process name without a space. In this case the
fequency of the IO is dependant on the "IO Frequency" parameter. It is also important to note that in this case
NO other process can be scheduled concurrently with an IO request. For more clarity see the examples below.
3.3 Example Input
7
C,8,0,1
E,5,3,0
F,30,3,10
G,5,3,3
B,3,10,2
A,14,13,2
D,27,14,4
3.4 Example Output for FCFS Scheduler
CICCIC CICCIC CIC CICCICCEEEEEFFFFFFFFFFFFFFFFFFFFF!FFFFFFFF
FFFGGG!GG G B B B B A A A A A A A A A A A A A A A A A A A ADDDDDDDDD !D
DDDDDDDDDDDDDDDDDDDDDDD
Here you can see that in the case of "Process C" every-time it is scheduled for 1 time unit it needs to be
scheduled for 1 time unit of IO except for the last time it is processed. Hence "C" only has 7 IO requests.
Likewise "F" should be scheduled for IO after every 10 normal time units except for the last time, therefore,
resulting in 2 total IO requests.
![import sys
import json
with open('config.json', 'r') as config_file:
config= json.load(config_file)
# Define the process data clas
class Process:
def _init__(self, name, duration, arrival_time, io_frequency) :
self.name = name
self.duration = duration
self.arrival time = arrival time
self.io_frequency = io_frequency
def main ():
# Check if the correct number of arguments is provided
import sys
if len (sys.argv) != 2:
return 1
# Extract the input file name from the command line arguments
input_file_name = f"Process_List/ {config['dataset']}/{ sys.argv[1]}"
# Define the number of processes
num_processes = 0
# Initialize an empty list for process data
data_set = []
# Open the file for reading
try:
with open (input_file_name, "r") as file:
# Read the number of processes from the file
num_processes = int (file.readline ().strip ())
# Read process data from the file and populate the data_set list
for in range (num_processes) :
line
file.readline ().strip ()
name, duration, arrival_time, io_frequency = line.split(',')
process Process (name, int (duration), int (arrival_time), int (io_frequency))
data_set.append (process)
except FileNot FoundError:
print("Error opening the file.")
return 1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2d6d90ff-3488-4045-9f18-920efa89fd99%2Fc8270d13-4f42-42c0-b6d2-a64b3948cb3a%2Ffng7vyl_processed.jpeg&w=3840&q=75)
Transcribed Image Text:import sys
import json
with open('config.json', 'r') as config_file:
config= json.load(config_file)
# Define the process data clas
class Process:
def _init__(self, name, duration, arrival_time, io_frequency) :
self.name = name
self.duration = duration
self.arrival time = arrival time
self.io_frequency = io_frequency
def main ():
# Check if the correct number of arguments is provided
import sys
if len (sys.argv) != 2:
return 1
# Extract the input file name from the command line arguments
input_file_name = f"Process_List/ {config['dataset']}/{ sys.argv[1]}"
# Define the number of processes
num_processes = 0
# Initialize an empty list for process data
data_set = []
# Open the file for reading
try:
with open (input_file_name, "r") as file:
# Read the number of processes from the file
num_processes = int (file.readline ().strip ())
# Read process data from the file and populate the data_set list
for in range (num_processes) :
line
file.readline ().strip ()
name, duration, arrival_time, io_frequency = line.split(',')
process Process (name, int (duration), int (arrival_time), int (io_frequency))
data_set.append (process)
except FileNot FoundError:
print("Error opening the file.")
return 1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps

Recommended textbooks for you
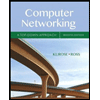
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
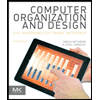
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
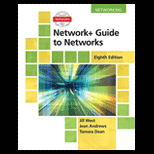
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
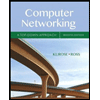
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
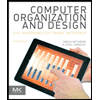
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
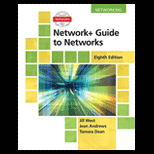
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
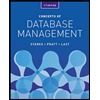
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
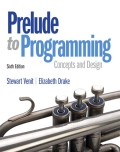
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
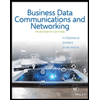
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY