Description: There are 5 philosophers sitting around a table and try to eat from the center of the table. 5 chopsticks also lay on the table. Let us call the 5 philosophers in clockwise P1, P2, ...P5. There also 5 chopsticks clockwise S1, S2, ..., S5 on the table. There are only one chopstick between every two philosophers. Every right hand chopstick will have the same index as the philosopher. For example, on the right hand side of P1, the chopstick is called S1. And every left hand side chopstick number is 1+number of the philosopher. A philosopher spend random time to think, then he feel hungry and try to eat. The middle dish can provide enough food for everyone at the same time. But a philosopher only can start to eat when he picked up two chopsticks from left hand side and right hand side to form a pair of chopsticks. If a philosopher take one chopsticks, he will try to fight with neighbours to get another one, and never back off to put down the one in his hand. Once the philosopher is eating, you can not interrupt him.
Description: There are 5 philosophers sitting around a table and try to eat from the center of the table. 5 chopsticks also lay on the table. Let us call the 5 philosophers in clockwise P1, P2, ...P5. There also 5 chopsticks clockwise S1, S2, ..., S5 on the table. There are only one chopstick between every two philosophers. Every right hand chopstick will have the same index as the philosopher. For example, on the right hand side of P1, the chopstick is called S1. And every left hand side chopstick number is 1+number of the philosopher. A philosopher spend random time to think, then he feel hungry and try to eat. The middle dish can provide enough food for everyone at the same time. But a philosopher only can start to eat when he picked up two chopsticks from left hand side and right hand side to form a pair of chopsticks. If a philosopher take one chopsticks, he will try to fight with neighbours to get another one, and never back off to put down the one in his hand. Once the philosopher is eating, you can not interrupt him.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Description: There are 5 philosophers sitting around a table and try to eat from the center of the table. 5 chopsticks also lay on the table. Let us call the 5 philosophers in clockwise P1, P2, ...P5. There also 5 chopsticks clockwise S1, S2, ..., S5 on the table. There are only one chopstick between every two philosophers. Every right hand chopstick will have the same index as the philosopher. For example, on the right hand side of P1, the chopstick is called S1. And every left hand side chopstick number is 1+number of the philosopher.
- A philosopher spend random time to think, then he feel hungry and try to eat.
- The middle dish can provide enough food for everyone at the same time.
- But a philosopher only can start to eat when he picked up two chopsticks from left hand side and right hand side to form a pair of chopsticks.
- If a philosopher take one chopsticks, he will try to fight with neighbours to get another one, and never back off to put down the one in his hand.
- Once the philosopher is eating, you can not interrupt him.
Here comes the deadlock problem: when all the 5 philosophers start to pick up the right hand side chopsticks at the same time, they get stuck.
We use a mutex, every time whenever one philosor start to pick chopsticks, he lock the mutex, and he is the only guy can pick chopsticks. After he get two chopsticks, he unlock the mutex.
Your first task is modifying the two lines between the beginning line and end line. You can insert and edit multiple lines between special comment lines in anyways, however, as the comment indicated, do not modify the special begin and comment lines themselves!
#include <stdio.h>
#include <stdlib.h>
#include <malloc.h>
#include <time.h>
#include <unistd.h>
#include <pthread.h>
#include <semaphore.h>
#define N 5
// here we use 5 different semaphores, each chopstick use one
// semaphore. Initally, the value for each is 1. After using one
// resource (chopstick), the value -1. 0 means currently not available
sem_t chopsticks[N];
// We do not use mutex, we set up a new semaphore
// called m
// #1#BEGIN# DO NOT MODIFIE THIS COMMENT LINE!
// #1#END# DO NOT MODIFIE THIS COMMENT LINE!
//The id of 5 philosophers
int philosophers[N] = {0, 1, 2, 3, 4};
void delay (int len) {
inti = rand() % len;
intx;
while (i > 0) {
x = rand() % len;
while (x > 0) {
x--;
}
i--;
}
}
void *philosopher (void* arg) {
inti = *(int *)arg;
intright = i;// right hand chopstick indexed as the same id of the philospher
intleft = (i + 1) % N;// left hand chopstick index as the id of the philospher + 1
while (1) {
printf("Philosopher %d is thinking\n", i);
delay(60000);
printf("Philosopher %d is hungry \n", i);
// wait for the semaphore m first, make sure no more than 4 philoshpers
// pick their first chopstick at the same time.
// #2#BEGIN# DO NOT MODIFIE THIS COMMENT LINE!
// #2#END# DO NOT MODIFIE THIS COMMENT LINE!
// wait for first (right) chopstick to use
// after using it, the value will decrease 1
// THAT IS WHY use need to use &, because the semaphore need to be modified
sem_wait(&chopsticks[right]);
printf("Philosopher %d picked up Chopstick %d, can not eat with one chopstick.\n", i, right);
// wati to use the left chopstick
sem_wait(&chopsticks[left]);
printf("Philosopher %d picked up Chopstick %d, now is eating with two chopsticks.\n", i, left);
delay(60000);
// release the first chopstick, increase the semaphore 1 by sem_post
sem_post(&chopsticks[right]);
printf("Philosopher %d put down Chopstick %d\n", i, right);
// when any right chopstick been released,
// increase semaphore m to release one capacity
// #3#BEGIN# DO NOT MODIFIE THIS COMMENT LINE!
// #3#END# DO NOT MODIFIE THIS COMMENT LINE!
sem_post(&chopsticks[left]);
printf("Philosopher %d put down Chopstick %d\n", i, left);
}
}
int main (int argc, char **argv) {
srand(time(NULL));
pthread_tphilo[N];
inti;
// change your the following id into your banner id
// #8#BEGIN# DO NOT MODIFIE THIS COMMENT LINE!
// #8#END# DO NOT MODIFIE THIS COMMENT LINE!
printf("Banner id: %d\n", banner_id);
// initalize the chopstick semphore
for (i=0; i<N; i++) {
// #4#BEGIN# DO NOT MODIFIE THIS COMMENT LINE!
// #4#END# DO NOT MODIFIE THIS COMMENT LINE!
}
// initalize the new semaphore m
// think what should be the inital value, and why?
// #5#BEGIN# DO NOT MODIFIE THIS COMMENT LINE!
// #5#END# DO NOT MODIFIE THIS COMMENT LINE!
// create threads
// #6#BEGIN# DO NOT MODIFIE THIS COMMENT LINE!
// #6#END# DO NOT MODIFIE THIS COMMENT LINE!
// put threads into the system
for (i=0; i<N; i++) {
pthread_join(philo[i], NULL);
}
// destroy semaphores
for (i=0; i<N; i++) {
sem_destroy(&chopsticks[i]);
}
// also do not forgor to destroy the m semaphore
// #7#BEGIN# DO NOT MODIFIE THIS COMMENT LINE!
// #7#END# DO NOT MODIFIE THIS COMMENT LINE!
return0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Recommended textbooks for you
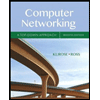
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
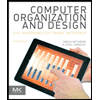
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
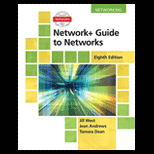
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
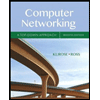
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
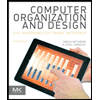
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
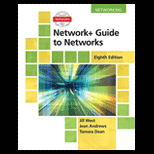
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
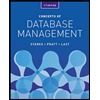
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
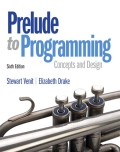
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
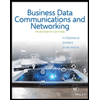
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY