For each function, describe what it actually does when called with a string argument. If it does not correctly check for lowercase letters, give an example argument that produces incorrect results, and describe why the result is incorrect. # 1 def any_lowercase1(s): for c in s: if c.islower(): return True else: return False # 2 def any_lowercase2(s): for c in s: if 'c'.islower(): return 'True' else: return 'False' # 3 def any_lowercase3(s): for c in s: flag = c.islower() return flag # 4 def any_lowercase4(s): flag = False for c in s: flag = flag or c.islower() return flag # 5 def any_lowercase5(s): for c in s: if not c.islower(): return False return True The code and its output must be explained technically whenever asked. The explanation can be provided before or after the code, or in the form of code comments within the code. For any descriptive type question, Your answer must be at least 150 words.
For each function, describe what it actually does when called with a string argument. If it does not correctly check for lowercase letters, give an example argument that produces incorrect results, and describe why the result is incorrect.
# 1
def any_lowercase1(s):
for c in s:
if c.islower():
return True
else:
return False
# 2
def any_lowercase2(s):
for c in s:
if 'c'.islower():
return 'True'
else:
return 'False'
# 3
def any_lowercase3(s):
for c in s:
flag = c.islower()
return flag
# 4
def any_lowercase4(s):
flag = False
for c in s:
flag = flag or c.islower()
return flag
# 5
def any_lowercase5(s):
for c in s:
if not c.islower():
return False
return True
The code and its output must be explained technically whenever asked. The explanation can be provided before or after the code, or in the form of code comments within the code. For any descriptive type question, Your answer must be at least 150 words.

#1
"any_lowercase1" checks if the input string 's' contains any lowercase letters. It iterates through the string, and for each character 'c', it checks if it is lowercase using the islower() method. If c is lowercase, the function returns True, otherwise, it returns False.
However, this implementation is flawed because it only checks the first character of the string. If the first character is not lowercase, the function returns False without checking the rest of the string.
Therefore, an incorrect result can be produced if the input string contains lowercase letters after the first character. For example, calling any_lowercase1("HELLOworld") would return False instead of True.
code:
def any_lowercase1(s):
# iterate through the string
for c in s:
# check if the character is lowercase
if c.islower():
# if a lowercase character is found, return True
return True
else:
# if not, return False (this line of code is misplaced)
return False
Trending now
This is a popular solution!
Step by step
Solved in 5 steps

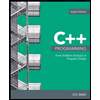
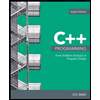