For this assignment, you'll build an application that calculates the car loan amortization (month by month breakdown for the life of the loan). Prompt the user to enter in the amount of the car loan, the APR, and the car payment per month including interest. The final output of your program should break down the monthly output for the user with the final line showing the total amount paid, total interest paid, and the number of months it took to pay off the car.
For this assignment, you'll build an application that calculates the car loan amortization (month by month breakdown for the life of the loan). Prompt the user to enter in the amount of the car loan, the APR, and the car payment per month including interest. The final output of your program should break down the monthly output for the user with the final line showing the total amount paid, total interest paid, and the number of months it took to pay off the car.
Chapter7: Characters, Strings, And The Stringbuilder
Section: Chapter Questions
Problem 1CP
Related questions
Question
I'm really confused about how to do this assignment but it involves looping. I have to do it through #C

Transcribed Image Text:Assignment 4
For this assignment, you'll build an application that calculates the car loan amortization (month by
month breakdown for the life of the loan). Prompt the user to enter in the amount of the car loan, the
APR, and the car payment per month including interest. The final output of your program should break
down the monthly output for the user with the final line showing the total amount paid, total interest
paid, and the number of months it took to pay off the car.
Sample
If the user enters a car loan amount of 20,000 with APR of 4.5 and monthly payment of 350, the
output should look something like this. Note that formatting won't be graded very harshly here for
spacing, but it's important to make sure you have the number values on each line as shown below and
that you are formatting money values as currency (ahem....ToString("C")).
Calculations from month to month will use what's known as simple interest to determine the interest
charge each month. Here's a breakdown of how it's calculated and your task will be translating this all to
C#:
1. Determine the monthly interest rate by dividing the APR entered by the user by 12. Then divide
by 100 since this is a percent
4.5/12/100 = 0.00375
2. Calculate the interest for the current month using the current balance. Make sure you round
this to 2 decimal places to prevent rounding errors from month to month (use Math. Round for
this since you actually want to round the number value instead of just format it for display):
21000 * 0.00375= 78.75
3. Calculate the principle paid for the current month by subtracting the interest from step 2 from
the payment
350-78.75 = 271.25
4. Calculate the remaining balance by adding interest to the previous balance and subtracting the
payment
2
21000 + 78.75 - 350= 20728.75
5. Repeat this until the balance gets down to 0
Most likely, there will be a special case in the last month where the total payment left
doesn't match the balance + new interest. In that case, you'll need to adjust the amount
paid as shown in this example
6. Keep track of the total amount paid and total interest to print on the final line.
Enter the total loan amount: 21000
What is your interest rate: 4.5
What is the monthly payment: 350
Month Interest - Principle -
1
2
$78.75
$77.73
Balance
$271.25 $20,728.75
$272.27 $20,456.48

Transcribed Image Text:51
52
53
54
55
56
57
58
59
60
61
$22.92
$21.70
$327.08
$328.30
$329.53
$330.77
$332.01
$333.25
$334.50
$335.76
$337.02
$338.28
$339.55
$340.82
$342.10
64
$343.38
65
$344.67
66
$345.96
67
$347.26
68
$348.56
69
$34.50
Total Paid: $23,834.63. Total Interest: $2,834.63. Months: 69
62
63
$20.47
$19.23
$17.99
$5,786.22
$5,457.92
$5,128.39
$4,797.62
$4,465.61
$4,132.36
$3,797.86
$3,462.10
$3,125.08
$2,786.80
$2,447.25
$2,106.43
$1,764.33
$1,420.95
$1,076.28
$16.75
$15.50
$14.24
$12.98
$11.72
$10.45
$9.18
$7.90
$6.62
$5.33
$4.04
$2.74
$1.44
$0.13
$730.32
$383.06
$34.50
$0.00
Takeaways
Once you have this program running, try plugging in different numbers to see how making larger or
smaller payments changes the total amount of interest paid over the course of a loan.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
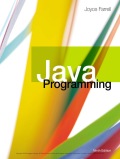
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
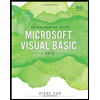
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
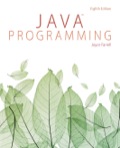
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
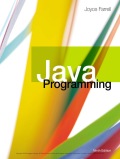
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
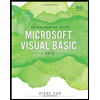
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
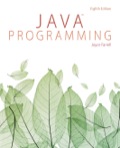
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
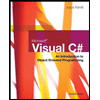
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,