GIVEN FILES //delete_product.php prepare($query); $statement->bindValue(':product_id', $product_id);
SQL
SQL stands for Structured Query Language, is a form of communication that uses queries structured in a specific format to store, manage & retrieve data from a relational database.
Queries
A query is a type of computer programming language that is used to retrieve data from a database. Databases are useful in a variety of ways. They enable the retrieval of records or parts of records, as well as the performance of various calculations prior to displaying the results. A search query is one type of query that many people perform several times per day. A search query is executed every time you use a search engine to find something. When you press the Enter key, the keywords are sent to the search engine, where they are processed by an algorithm that retrieves related results from the search index. Your query's results are displayed on a search engine results page, or SER.
GIVEN FILES
//delete_product.php
<?php
require_once('
// Get IDs
$product_id = filter_input(INPUT_POST, 'product_id', FILTER_VALIDATE_INT);
$category_id = filter_input(INPUT_POST, 'category_id', FILTER_VALIDATE_INT);
// Delete the product from the database
if ($product_id != FALSE && $category_id != FALSE) {
$query = 'DELETE FROM products
WHERE productID = :product_id';
$statement = $db->prepare($query);
$statement->bindValue(':product_id', $product_id);
$success = $statement->execute();
$statement->closeCursor();
}
// Display the Product List page
include('index.php');
//delete_category.php
<?php
// Get ID
$category_id = filter_input(INPUT_POST, 'category_id', FILTER_VALIDATE_INT);
// Validate inputs
if ($category_id == NULL || $category_id == FALSE) {
$error = "Invalid category ID.";
include('error.php');
} else {
require_once('database.php');
// Add the product to the database
$query = 'DELETE FROM categories
WHERE categoryID = :category_id';
$statement = $db->prepare($query);
$statement->bindValue(':category_id', $category_id);
$statement->execute();
$statement->closeCursor();
// Display the Category List page
include('category_list.php');
}
?>
//display_results.php
<?php
// get the data from the form
$investment = filter_input(INPUT_POST, 'investment',
FILTER_VALIDATE_FLOAT);
$interest_rate = filter_input(INPUT_POST, 'interest_rate',
FILTER_VALIDATE_FLOAT);
$years = filter_input(INPUT_POST, 'years',
FILTER_VALIDATE_INT);
// set default error message of empty string
$error_message = '';
// validate investment
if ($investment === FALSE ) {
$error_message .= 'Investment must be a valid number.<br>';
} else if ( $investment <= 0 ) {
$error_message .= 'Investment must be greater than zero.<br>';
}
// validate interest rate
if ( $interest_rate === FALSE ) {
$error_message .= 'Interest rate must be a valid number.<br>';
} else if ( $interest_rate <= 0 ) {
$error_message .= 'Interest rate must be greater than zero.<br>';
} else if ( $interest_rate > 15 ) {
$error_message .= 'Interest rate must be less than or equal to 15.<br>';
}
// validate years
if ( $years === FALSE ) {
$error_message .= 'Years must be a valid whole number.<br>';
} else if ( $years <= 0 ) {
$error_message .= 'Years must be greater than zero.<br>';
} else if ( $years > 30 ) {
$error_message .= 'Years must be less than 31.<br>';
}
// if an error message exists, go to the index page
if ($error_message != '') {
include('index.php');
exit();
}
// calculate the future value
$future_value = $investment;
for ($i = 1; $i <= $years; $i++) {
$future_value += $future_value * $interest_rate *.01;
}
// apply currency and percent formatting
$investment_f = '$'.number_format($investment, 2);
$yearly_rate_f = $interest_rate.'%';
$future_value_f = '$'.number_format($future_value, 2);
?>
<!DOCTYPE html>
<html>
<head>
<title>Future Value Calculator</title>
<link rel="stylesheet" href="main.css"/>
</head>
<body>
<main>
<h1>Future Value Calculator</h1>
<label>Investment Amount:</label>
<span><?php echo htmlspecialchars($investment_f); ?></span><br />
<label>Yearly Interest Rate:</label>
<span><?php echo htmlspecialchars($yearly_rate_f); ?></span><br />
<label>Number of Years:</label>
<span><?php echo htmlspecialchars($years); ?></span><br />
<label>Future Value:</label>
<span><?php echo htmlspecialchars($future_value_f); ?></span><br />
<p>This calculation was done on <?php echo date('m/d/Y'); ?>.</p>
</main>
</body>
</html>


Step by step
Solved in 2 steps

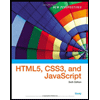
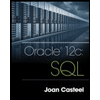
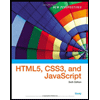
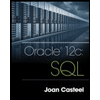