Help, I making a elevator simulator, I need help in writing code in the Elevator class and it's polymorphism class. I am not sure what to do next. Any help is appreciated. There are 4 types of passengers in the system: Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements. VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators. Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators. Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. There are 4 types of elevators in the system: StandardElevator: This is the most common type of elevator and has a request percentage of 70%. Standard elevators have a maximum capacity of 10 passengers. ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a maximum capacity of 8 passengers and are faster than standard elevators. FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a maximum capacity of 5 passengers and are designed to transport heavy items. GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a maximum capacity of 6 passengers and are designed to transport fragile item This is all I could think of import java.util.Random; public abstract class Passenger { public static int passengerCounter = 0; private String passengerID; protected int startFloor; protected int endFloor; Passenger() { this.passengerID = "" + passengerCounter; passengerCounter++; } public abstract boolean requestElevator(SimulatorSettings settings); } class StandardPassenger extends Passenger { public StandardPassenger() { super(); } @Override public boolean requestElevator(SimulatorSettings settings) { Random rand = new Random(); this.startFloor = rand.nextInt(settings.getNofloors()); this.endFloor = rand.nextInt(settings.getNofloors()); while (this.startFloor == this.endFloor) { this.endFloor = rand.nextInt(settings.getNofloors()); } return true; } } class VIPPassenger extends Passenger { public VIPPassenger() { super(); } @Override public boolean requestElevator(SimulatorSettings settings) { // Request an express elevator int expressElevator = settings.getNofloors() / 2; this.startFloor = expressElevator; this.endFloor = expressElevator; return true; } } class FreightPassenger extends Passenger { public FreightPassenger() { super(); } @Override public boolean requestElevator(SimulatorSettings settings) { Random rand = new Random(); this.startFloor = rand.nextInt(settings.getNofloors()); this.endFloor = rand.nextInt(settings.getNofloors()); while (this.startFloor == this.endFloor) { this.endFloor = rand.nextInt(settings.getNofloors()); } return true; } } class GlassPassenger extends Passenger { public GlassPassenger() { super(); } @Override public boolean requestElevator(SimulatorSettings settings) { Random rand = new Random(); this.startFloor = rand.nextInt(settings.getNofloors()); this.endFloor = rand.nextInt(settings.getNofloors()); while (this.startFloor == this.endFloor) { this.endFloor = rand.nextInt(settings.getNofloors()); } return true; } } public abstract class Elevator{ } public class StandardElevator extends Elevator{ } public class ExpressElevator extends Elevator{ } public class FreightElevator extends Elevator{ } public class GlassElevator extends Elevator{ }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Help, I making a elevator simulator, I need help in writing code in the Elevator class and it's polymorphism class. I am not sure what to do next. Any help is appreciated.
There are 4 types of passengers in the system:
Standard: This is the most common type of passenger and has a request percentage of 70%.
Standard passengers have no special requirements.
VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority
and are more likely to be picked up by express elevators.
Freight: This type of passenger has a request percentage of 15%. Freight passengers have large
items that need to be transported and are more likely to be picked up by freight elevators.
Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items
that need to be transported and are more likely to be picked up by glass elevators.
There are 4 types of elevators in the system:
StandardElevator: This is the most common type of elevator and has a request percentage of 70%.
Standard elevators have a maximum capacity of 10 passengers.
ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a
maximum capacity of 8 passengers and are faster than standard elevators.
FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a
maximum capacity of 5 passengers and are designed to transport heavy items.
GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a
maximum capacity of 6 passengers and are designed to transport fragile item
This is all I could think of
import java.util.Random;
public abstract class Passenger {
public static int passengerCounter = 0;
private String passengerID;
protected int startFloor;
protected int endFloor;
Passenger() {
this.passengerID = "" + passengerCounter;
passengerCounter++;
}
public abstract boolean requestElevator(SimulatorSettings settings);
}
class StandardPassenger extends Passenger {
public StandardPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
Random rand = new Random();
this.startFloor = rand.nextInt(settings.getNofloors());
this.endFloor = rand.nextInt(settings.getNofloors());
while (this.startFloor == this.endFloor) {
this.endFloor = rand.nextInt(settings.getNofloors());
}
return true;
}
}
class VIPPassenger extends Passenger {
public VIPPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
// Request an express elevator
int expressElevator = settings.getNofloors() / 2;
this.startFloor = expressElevator;
this.endFloor = expressElevator;
return true;
}
}
class FreightPassenger extends Passenger {
public FreightPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
Random rand = new Random();
this.startFloor = rand.nextInt(settings.getNofloors());
this.endFloor = rand.nextInt(settings.getNofloors());
while (this.startFloor == this.endFloor) {
this.endFloor = rand.nextInt(settings.getNofloors());
}
return true;
}
}
class GlassPassenger extends Passenger {
public GlassPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
Random rand = new Random();
this.startFloor = rand.nextInt(settings.getNofloors());
this.endFloor = rand.nextInt(settings.getNofloors());
while (this.startFloor == this.endFloor) {
this.endFloor = rand.nextInt(settings.getNofloors());
}
return true;
}
}
public abstract class Elevator{
}
public class StandardElevator extends Elevator{
}
public class ExpressElevator extends Elevator{
}
public class FreightElevator extends Elevator{
}
public class GlassElevator extends Elevator{
}

Step by step
Solved in 4 steps with 3 images

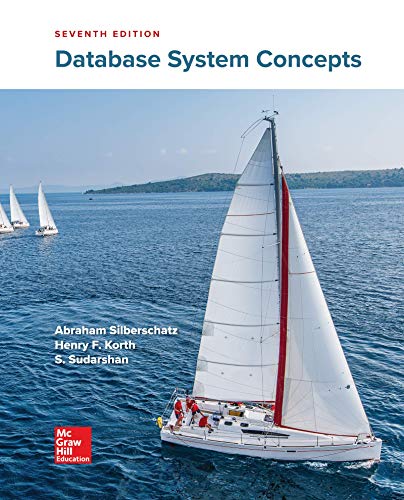
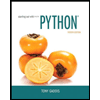
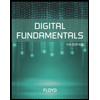
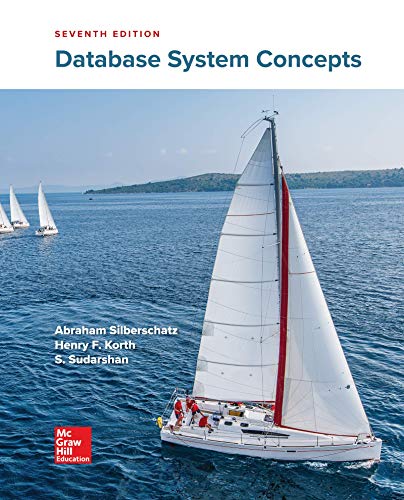
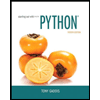
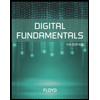
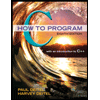
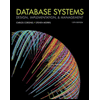
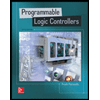