What your Python program should do In the main part of your code outside of the functions You need to create this code. Ask the user to type in an order in this format (without the square brackets): [table number]&[the name of the dish]$[c, g, s, or p] The number is the table number. This is followed by an ampersand ‘&’. Next is the name of the dish. This is followed by a dollar sign ‘$’. At the end is c, g, s, or p, in lowercase. This denotes which chef will receive the order. ‘c’ means that the order will go to the cold bar (salads, appetizers). ‘g’ means that it will go to the grill. ‘s’ means that it will go to the saute station. ‘p’ means that it will go to the pastry chef. For example: 3&creme brulee$p This means table number 3, the dish is called ‘creme brulee’, and it is going to the pastry chef. The user will type all of this on one line with no spaces except for in the dish name. Store this order into a list. Let the user keep typing in orders. Store all orders in the same list. The user stops entering orders by typing in ‘x’ without quotes. Once you have all of the orders, access each order in the list and send it to a function called profrich() that takes in the order as a parameter. Send each order to a function called profrich(order) You need to create the code for this function. The function definition and the parameter is given to you in the starter code. Do not change the name of this function. The function separates out the various components of the order: table number, dish name, and which chef it is going to. You need to send these three components to another function that will return a string. Send these three components to a function called printout(table, dish, chef) You need to create the code for this function. The function definition and the parameter is given to you in the starter code. Do not change the name of this function. The output of this function is a string with the order details that is nicely formatted so the chef can easily read it. First, add “T“, the table number, and a space to the string. Next, process the dish name. Add the dish name to the string. If the length of the dish name is 10 characters or fewer, add the text “easy” to the string to let the chef know it’s an easy dish to cook. If the length of the dish name is more than 10 characters, add the text “RUSH” to the string to let the chef know they’re going to have to cook quickly to get the dish out on time. Finally, look at the last letter in the order. If it is ‘c’, add ‘cold’ to the string. If it is ‘g’, add ‘grill’ to the string. If it is ‘s’, add ‘saute’ to the string. If it is ‘p’, add ‘pastry’ to the string. Include spaces in the string like in normal writing. Using our example from above, the order “3&creme brulee$p” would result in a string: T3 creme brulee RUSH pastry Return this string back to profrich(). Back inside profrich() You need to continue creating the code for this function. Store the string returned from printout(). Print it for the user. profrich() does not need to return anything. Back inside the main part of your program This kicks the program back down to the main part (outside of the functions) where your code should automatically pull the next order from the list and send it to profrich(). You need to create this functionality. Once you’ve processed all the orders, your program can end and show a funny message to the wait staff (e.g., your TA).
What your Python program should do
In the main part of your code outside of the functions
You need to create this code.
Ask the user to type in an order in this format (without the square brackets):
[table number]&[the name of the dish]$[c, g, s, or p]
The number is the table number. This is followed by an ampersand ‘&’.
Next is the name of the dish.
This is followed by a dollar sign ‘$’.
At the end is c, g, s, or p, in lowercase. This denotes which chef will receive the order. ‘c’ means that the order will go to the cold bar (salads, appetizers). ‘g’ means that it will go to the grill. ‘s’ means that it will go to the saute station. ‘p’ means that it will go to the pastry chef.
For example:
3&creme brulee$p
This means table number 3, the dish is called ‘creme brulee’, and it is going to the pastry chef.
The user will type all of this on one line with no spaces except for in the dish name.
Store this order into a list.
Let the user keep typing in orders. Store all orders in the same list. The user stops entering orders by typing in ‘x’ without quotes.
Once you have all of the orders, access each order in the list and send it to a function called profrich() that takes in the order as a parameter.
Send each order to a function called profrich(order)
You need to create the code for this function. The function definition and the parameter is given to you in the starter code. Do not change the name of this function.
The function separates out the various components of the order: table number, dish name, and which chef it is going to.
You need to send these three components to another function that will return a string.
Send these three components to a function called printout(table, dish, chef)
You need to create the code for this function. The function definition and the parameter is given to you in the starter code. Do not change the name of this function.
The output of this function is a string with the order details that is nicely formatted so the chef can easily read it.
First, add “T“, the table number, and a space to the string.
Next, process the dish name. Add the dish name to the string.
- If the length of the dish name is 10 characters or fewer, add the text “easy” to the string to let the chef know it’s an easy dish to cook.
- If the length of the dish name is more than 10 characters, add the text “RUSH” to the string to let the chef know they’re going to have to cook quickly to get the dish out on time.
Finally, look at the last letter in the order. If it is ‘c’, add ‘cold’ to the string. If it is ‘g’, add ‘grill’ to the string. If it is ‘s’, add ‘saute’ to the string. If it is ‘p’, add ‘pastry’ to the string.
Include spaces in the string like in normal writing.
Using our example from above, the order “3&creme brulee$p” would result in a string:
T3 creme brulee RUSH pastry
Return this string back to profrich().
Back inside profrich()
You need to continue creating the code for this function.
Store the string returned from printout(). Print it for the user.
profrich() does not need to return anything.
Back inside the main part of your program
This kicks the program back down to the main part (outside of the functions) where your code should automatically pull the next order from the list and send it to profrich(). You need to create this functionality.
Once you’ve processed all the orders, your program can end and show a funny message to the wait staff (e.g., your TA).
note by me = I sennt an output of what it should look like

![main.py x +
23 ▼
24
25 ▼
26
if chef == 'S':
output output + "saute"
if chef == 'p':
output = output + "pastry"
return output
27
28
29 while order != 'X':
30
31
32
33 for order in orders:
34
35
36
37
38
39
40
41
42
43
Line 28: Col 5
orders.append(order)
order = input ('Order: ')
orde = order.split('&')
table orde[0]
ordd = orde[1].split('$¹)
ordered ordd[0]
chefordd [1]
order = [table, ordered, chef]
profrich(order)
> Console x +
HELLO WELCOME TO MARIA'S KITCHEN WAIT STAFF. Enter orders one at a time or x to quit
Order: 1&steak$g
Order: x
Traceback (most recent call last):
File "main.py", line 33, in <module>
orde = order.split('&')
AttributeError: 'list' object has no attribute 'split'
KeyboardInterrupt
⠀
History C](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F309a0b45-81e4-45d0-8860-fe87ea651e15%2F6aaf92aa-4a7a-424e-9272-38f8b908524d%2Fvqbwpk4_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

its still saying list object has no attritube split

the output is also missing a few things. this is what he output should look like
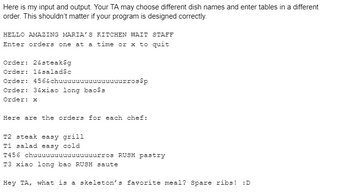
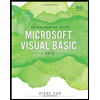
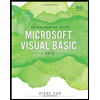