Implementing the Customer Class We've partially defined the Customer class for you in "customer.h" and provided the member variables for you for a Customer name representing the name of the Customer, product_count_ representing the number of products in the Customer's shopping cart, and next_customer representing the next Customer in line, if there is one. You are tasked with defining the constructors, accessor functions, and recursive functions. 1. Create a non-default constructor which accepts 3 parameters: a const reference to a std::string for the customer's name, an int parameter for the product count, and a shared pointer to the next customer in line. 2. Create accessor functions GetName, GetProductCount, and GetNextCustomer, which each should return the corresponding member variable. 3. Define the recursive member function TotalCustomers InLine, which accepts no input, and returns an int representing the total number of customers waiting in line starting at the current customer and including all customers lined up behind them. 4. Define the recursive member function Total Products InLine, which accepts no input, and returns an int representing the total number of products held by customers waiting in line starting at the current customer and including all customers lined up behind them. 5. Define the recursive member function FirstAlphabeticalCustomer InLine, which accepts no input, and returns a std::string which is the name of the customer in line whose name comes first alphabetically, compared to all other customers lined up behind them. Hint: for this question, you can use < or > to strings. In main.cc, you will be asked to invoke each recursive function for a line of customers we've provided for you, starting with Adele at the front of the line. You are tasked with asking the customer at the front of the line how many customers are waiting in total, how many products are waiting to be purchased in the line, and the name of the customer in line that comes first alphabetically. After running main, your output should be: Total customers waiting: 5 Total products to be purchased: 34 First customer name alphabetically: Adele
main.cc file
#include <iostream>
#include <memory>
#include "customer.h"
int main() {
// Creates a line of customers with Adele at the front.
// LinkedList diagram:
// Adele -> Kehlani -> Giveon -> Drake -> Ruel
std::shared_ptr<Customer> ruel =
std::make_shared<Customer>("Ruel", 5, nullptr);
std::shared_ptr<Customer> drake =
std::make_shared<Customer>("Drake", 8, ruel);
std::shared_ptr<Customer> giveon =
std::make_shared<Customer>("Giveon", 2, drake);
std::shared_ptr<Customer> kehlani =
std::make_shared<Customer>("Kehlani", 15, giveon);
std::shared_ptr<Customer> adele =
std::make_shared<Customer>("Adele", 4, kehlani);
std::cout << "Total customers waiting: ";
// =================== YOUR CODE HERE ===================
// 1. Print out the total number of customers waiting
// in line by invoking TotalCustomersInLine.
// ======================================================
std::cout << std::endl;
std::cout << "Total products to be purchased: ";
// =================== YOUR CODE HERE ===================
// 2. Print out the total number of products held by
// customers in line by invoking TotalProductsInLine.
// ======================================================
std::cout << std::endl;
std::cout << "First customer name alphabetically: ";
// =================== YOUR CODE HERE ===================
// 3. Print out the name of the customer in line whose
// name comes first alphabetically by invoking
// FirstAlphabeticalCustomerInLine.
// ======================================================
std::cout << std::endl;
return 0;
}
customer.cc file
#include "customer.h"
// ========================= YOUR CODE HERE =========================
// This implementation file (customer.cc) is where you should implement
// the member functions declared in the header (customer.h), only
// if you didn't implement them inline within customer.h.
//
// Remember to specify the name of the class with :: in this format:
// <return type> MyClassName::MyFunction() {
// ...
// }
// to tell the compiler that each function belongs to the Customer class.
// ===================================================================
customer.h file
#include <memory>
#include <string>
class Customer {
public:
// ====================== YOUR CODE HERE ======================
// 1. Define a constructor using member initializer list syntax
// according to the README.
// 2. Define the accessor functions (i.e. the getter functions)
// `GetName`, `GetProductCount`, and `GetNextCustomer`.
// You do not need to create mutator functions (setters).
// 3. Define the recursive functions specified in the README.
// ============================================================
private:
std::string name_;
int product_count_;
std::shared_ptr<Customer> next_customer_;
};
Sample Output:



Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 4 images

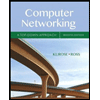
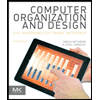
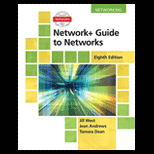
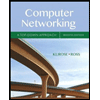
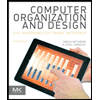
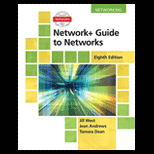
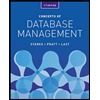
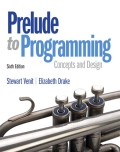
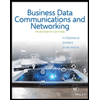