In this c++ program please explain everyline of the code and explain the output. Thank you Source Code: #include using namespace std; class Node { public: string data; Node* next; Node(string data){ this->data=data; this->next=NULL; } }; class Linkedlist{ Node *head=NULL; public: Linkedlist(){ Node *head=NULL; } //addNode() will add a new node to the list void addNode(string data) { //Create a new node Node* newNode=new Node(data); //Checks if the list is empty if(head == NULL) { //If list is empty, head will point to new node head = newNode; } else if(head != NULL) { Node* temp = head; while (temp->next != NULL) { temp = temp->next; } // Insert at the last. temp->next = newNode; } } // deleteAtIndex() will delete a node at specific index void deleteAtIndex(int index){ if(head == NULL){ cout<<"List is empty\n"; } else{ //if index is starting position if(index==1){ head=head->next; } else{ Node *temp=head; for(int i=2;inext!=NULL) { temp = temp->next; } } temp->next = temp->next->next; } } } void printList() { Node *n=head; if(n==NULL) cout<<"List is empty\n"; else{ cout<<"\nYou entered the following names:\n"; while (n != NULL) { cout << n->data <next; } } } }; int main() { Linkedlist l; while(1){ cout<<"\n1 - Enter name in the list.\n"; cout<<"1 - Delete name from the list.\n"; cout<<"3 - Display all names.\n"; cout<<"4 - Exit.\n"; cout<<"\nEnter your choice: "; int ch; cin>>ch; if(ch==1){ string name; cout<<"\nEnter Name: "; getline(cin >> ws, name); l.addNode(name); } else if(ch==2){ int index; cout<<"\nEnter the name position to be deleted: "; cin>>index; l.deleteAtIndex(index); } else if(ch==3){ l.printList(); } else if(ch==4){ break; } } }
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
In this c++ program please explain everyline of the code and explain the output. Thank you
Source Code:
#include <iostream>
using namespace std;
class Node {
public:
string data;
Node* next;
Node(string data){
this->data=data;
this->next=NULL;
}
};
class Linkedlist{
Node *head=NULL;
public:
Linkedlist(){
Node *head=NULL;
}
//addNode() will add a new node to the list
void addNode(string data) {
//Create a new node
Node* newNode=new Node(data);
//Checks if the list is empty
if(head == NULL) {
//If list is empty, head will point to new node
head = newNode;
}
else if(head != NULL) {
Node* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
// Insert at the last.
temp->next = newNode;
}
}
// deleteAtIndex() will delete a node at specific index
void deleteAtIndex(int index){
if(head == NULL){
cout<<"List is empty\n";
}
else{
//if index is starting position
if(index==1){
head=head->next;
}
else{
Node *temp=head;
for(int i=2;i<index;i++) {
if(temp->next!=NULL) {
temp = temp->next;
}
}
temp->next = temp->next->next;
}
}
}
void printList()
{
Node *n=head;
if(n==NULL)
cout<<"List is empty\n";
else{
cout<<"\nYou entered the following names:\n";
while (n != NULL) {
cout << n->data <<endl;
n = n->next;
}
}
}
};
int main() {
Linkedlist l;
while(1){
cout<<"\n1 - Enter name in the list.\n";
cout<<"1 - Delete name from the list.\n";
cout<<"3 - Display all names.\n";
cout<<"4 - Exit.\n";
cout<<"\nEnter your choice: ";
int ch;
cin>>ch;
if(ch==1){
string name;
cout<<"\nEnter Name: ";
getline(cin >> ws, name);
l.addNode(name);
}
else if(ch==2){
int index;
cout<<"\nEnter the name position to be deleted: ";
cin>>index;
l.deleteAtIndex(index);
}
else if(ch==3){
l.printList();
}
else if(ch==4){
break;
}
}
}

Step by step
Solved in 3 steps with 2 images

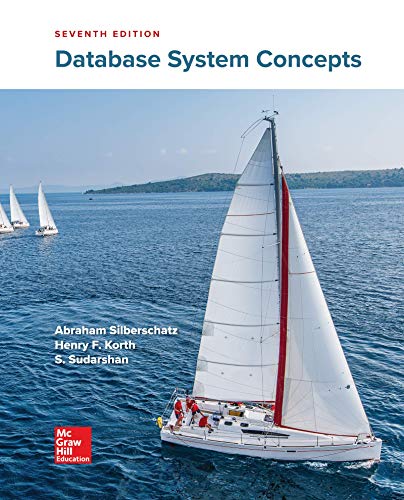
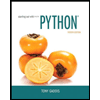
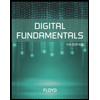
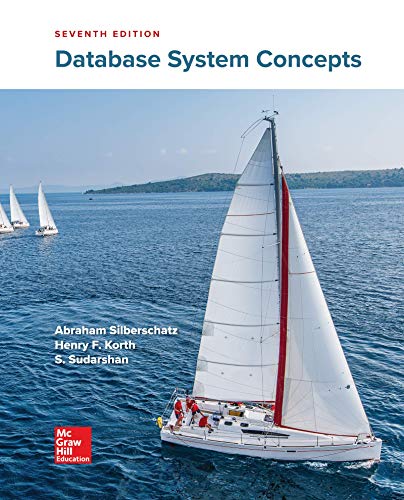
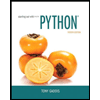
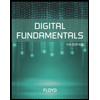
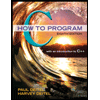
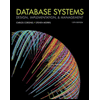
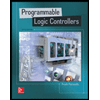