#include <stdio.h>#include <stdlib.h> int cent50 = 0;int cent20 = 0;int cent10 = 0;int cent05 = 0; //Function definitionvoid calculateChange(int change) {if(change > 0) {if(change >= 50) {change -= 50;cent50++;} else if(change >= 20) {change -= 20;cent20++;} else if(change >= 10) {change -= 10;cent10++;} else if(change >= 05) {change -= 05;cent05++;}calculateChange(change);}} //Define the functionvoid printChange() { if(cent50)printf("\n50 Cents : %d coins", cent50); if(cent20)printf("\n20 Cents : %d coins", cent20); if(cent10)printf("\n10 Cents : %d coins", cent10); if(cent05)printf("\n05 Cents : %d coins", cent05); cent50 = 0;cent20 = 0;cent10 = 0;cent05 = 0; } //Function's definitionint TakeChange() { int change;printf("\nEnter the amount : ");scanf("%d", &change);return change; }//main functionint main() {//call the functionint change = TakeChange(); //use while-loop to repeatedly ask for input to the userwhile(change != -1){if((change % 5)==0){if(change >= 5 && change <=95 ){calculateChange(change); //Calculate coins}else {printf("\nPlease Enter the value between 5 and 95:");}}else{printf("\nPlease Enter the valid value:");} //Print coinsprintChange(); printf("\nEnter the amount to continue or Enter -1 to exit: ");scanf("%d", &change); }return 0;} With the C program above how to fill the table below set of test data in tabular form with expected results and desk check results from the algorithm. Each test data must be justified – reason for selecting that data. Test id Test description/justification – what is the test for and why this particular test. Actual data for this test Expected output Actual desk check; result when desk check is carried out Test outcome – Pass/Fail 1 2 3 4
#include <stdio.h>
#include <stdlib.h>
int cent50 = 0;
int cent20 = 0;
int cent10 = 0;
int cent05 = 0;
//Function definition
void calculateChange(int change) {
if(change > 0) {
if(change >= 50) {
change -= 50;
cent50++;
}
else if(change >= 20) {
change -= 20;
cent20++;
}
else if(change >= 10) {
change -= 10;
cent10++;
}
else if(change >= 05) {
change -= 05;
cent05++;
}
calculateChange(change);
}
}
//Define the function
void printChange() {
if(cent50)
printf("\n50 Cents : %d coins", cent50);
if(cent20)
printf("\n20 Cents : %d coins", cent20);
if(cent10)
printf("\n10 Cents : %d coins", cent10);
if(cent05)
printf("\n05 Cents : %d coins", cent05);
cent50 = 0;
cent20 = 0;
cent10 = 0;
cent05 = 0;
}
//Function's definition
int TakeChange() {
int change;
printf("\nEnter the amount : ");
scanf("%d", &change);
return change;
}
//main function
int main() {
//call the function
int change = TakeChange();
//use while-loop to repeatedly ask for input to the user
while(change != -1)
{
if((change % 5)==0)
{
if(change >= 5 && change <=95 ){
calculateChange(change); //Calculate coins
}
else {
printf("\nPlease Enter the value between 5 and 95:");
}
}
else
{
printf("\nPlease Enter the valid value:");
}
//Print coins
printChange();
printf("\nEnter the amount to continue or Enter -1 to exit: ");
scanf("%d", &change);
}
return 0;
}
With the C program above how to fill the table below set of test data in tabular form with expected results and desk check results from the
Test id |
Test description/justification – what is the test for and why this particular test. |
Actual data for this test |
Expected output |
Actual desk check; result when desk check is carried out |
Test outcome – Pass/Fail |
1 |
|
|
|
|
|
2 |
|
|
|
|
|
3 |
|
|
|
|
|
4 |
|
|
|
|
|

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

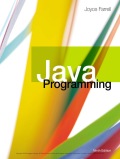
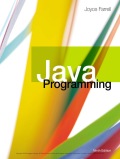