n Java code: Write the class encapsulating the concept of money, assuming that money has the following attributes: dollars, cents In addition to the constructors, the accessors and mutators, write the following methods: public Money() public Money(int dollars, int cents) public Money add(Money m) public Money substract(Money m) public Money multiply(int m) public static Money[] multiply(Money[] moneys, int amt) public boolean equals(Money money) public String toString() private void normalize() // normalize dollars and cents field Add additional helper methods if necessary. Use the following test driver program to test your Money class: public class MoneyTester { public static void main(String[] args) { Money m1 = new Money(8, 75); // set dollars to 8 and cents to 75 Money m2 = new Money(5, 80); // set dollars to 5 and cents to 80 Money Money m3 = new Money(); // initialize dollars to 0 and cents to 0 System.out.println("\tJane Doe " + "CIS35A Spring 2021 Lab 4"); // use your name System.out.println("m1 = " + m1); System.out.println("m2 = " + m2); System.out.println("m3 = " + m3); System.out.println("m1 equals m2? " + m1.equals(m2)); System.out.println("m1 equals m3? " + m1.equals(m3)); Money m4 = m1.add(m2); System.out.println("m4 = m1 + m2 = " + m1.add(m2)); Money m5 = m4.multiply(3); System.out.println("m5 = m4 * 3 = " + m5); System.out.println("m1 + m2 + m3 + m4 = " + m1.add(m2).add(m3).add(m4)); System.out.println("m4 - m2 = " + m4.subtract(m2)); Money[] m6 = new Money[]{new Money(10, 50), new Money(20, 50), new Money(30, 50), new Money(40, 50)}; Money[] m7 = Money.multiply(m6, 2); System.out.print("m6 = ("); for(int i = 0; i < m6.length; i++) { if(i < m6.length -1) System.out.print(m6[i] + ", "); else System.out.print(m6[i] + ")"); } System.out.println(); System.out.print("m7 = m6 * 2 = ("); for(int i = 0; i < m7.length; i++) { if (i < m7.length -1) System.out.print(m7[i] + ", "); else System.out.print(m7[i] + ")"); } System.out.println(); } } Your output will look similar to this Jane Doe CIS35A Fall 2022 Lab 4 m1 = 8.75 m2 = 5.80 m3 = 0.0 m1 equals m2? false m1 equals m3? false m4 = m1 + m2 = 14.55 m5 = m4 * 3 = 43.65 m1 + m2 + m3 + m4 = 29.10 m4 - m2 = 8.75 m6 = (10.50, 20.50, 30.50, 40.50) m7 = m6 * 2 = (21.0, 41.0, 61.0, 81.0) Java source code (Money.java and MoneyTester.java). Output of the sample run
In Java code:
Write the class encapsulating the concept of money, assuming that money has the following attributes: dollars, cents
In addition to the constructors, the accessors and mutators, write the following methods:
public Money()
public Money(int dollars, int cents)
public Money add(Money m)
public Money substract(Money m)
public Money multiply(int m)
public static Money[] multiply(Money[] moneys, int amt)
public boolean equals(Money money)
public String toString()
private void normalize() // normalize dollars and cents field
Add additional helper methods if necessary.
Use the following test driver program to test your Money class:
public class MoneyTester
{
public static void main(String[] args)
{
Money m1 = new Money(8, 75); // set dollars to 8 and cents to 75
Money m2 = new Money(5, 80); // set dollars to 5 and cents to 80 Money
Money m3 = new Money(); // initialize dollars to 0 and cents to 0
System.out.println("\tJane Doe " + "CIS35A Spring 2021 Lab 4"); // use
your name
System.out.println("m1 = " + m1);
System.out.println("m2 = " + m2);
System.out.println("m3 = " + m3);
System.out.println("m1 equals m2? " + m1.equals(m2));
System.out.println("m1 equals m3? " + m1.equals(m3));
Money m4 = m1.add(m2);
System.out.println("m4 = m1 + m2 = " + m1.add(m2));
Money m5 = m4.multiply(3);
System.out.println("m5 = m4 * 3 = " + m5);
System.out.println("m1 + m2 + m3 + m4 = " +
m1.add(m2).add(m3).add(m4));
System.out.println("m4 - m2 = " + m4.subtract(m2));
Money[] m6 = new Money[]{new Money(10, 50), new Money(20, 50), new
Money(30, 50), new Money(40, 50)};
Money[] m7 = Money.multiply(m6, 2);
System.out.print("m6 = (");
for(int i = 0; i < m6.length; i++)
{
if(i < m6.length -1)
System.out.print(m6[i] + ", ");
else
System.out.print(m6[i] + ")");
}
System.out.println();
System.out.print("m7 = m6 * 2 = (");
for(int i = 0; i < m7.length; i++)
{
if (i < m7.length -1)
System.out.print(m7[i] + ", ");
else
System.out.print(m7[i] + ")");
}
System.out.println();
}
}
Your output will look similar to this
Jane Doe CIS35A Fall 2022 Lab 4
m1 = 8.75
m2 = 5.80
m3 = 0.0
m1 equals m2? false
m1 equals m3? false
m4 = m1 + m2 = 14.55
m5 = m4 * 3 = 43.65
m1 + m2 + m3 + m4 = 29.10
m4 - m2 = 8.75
m6 = (10.50, 20.50, 30.50, 40.50)
m7 = m6 * 2 = (21.0, 41.0, 61.0, 81.0)
- Java source code (Money.java and MoneyTester.java).
- Output of the sample run

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 3 images

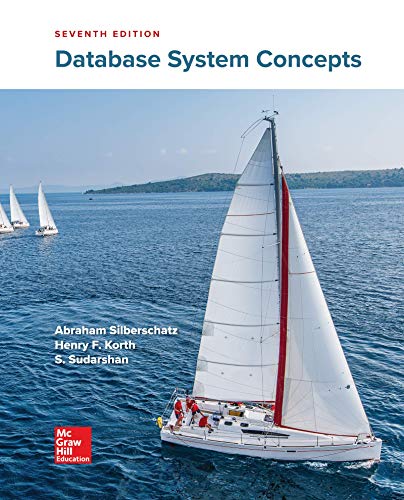
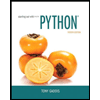
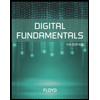
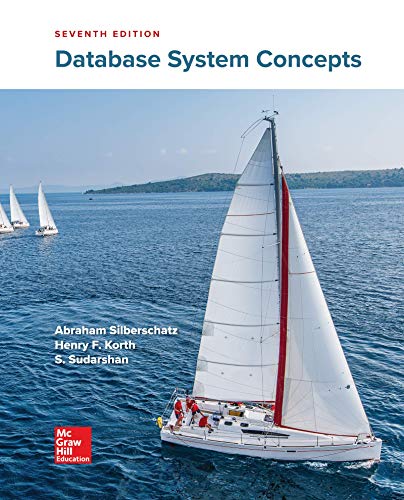
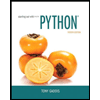
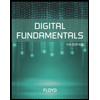
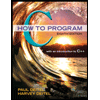
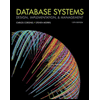
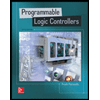