objective of the project: Implement a class address. An address has a house number street optional apartment number city state postal code. All member variables should be private and the member functions should be public. Implement two constructors: one with an apartment number one without an appartment number.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
objective of the project:
- Implement a class address.
- An address has a
- house number
- street
- optional apartment number
- city
- state
- postal code.
- All member variables should be private and the member functions should be public.
- Implement two constructors:
- one with an apartment number
- one without an appartment number.
- Implement a print function that prints the address with the street on one line and the city, state, and postal code on the next line.
- Implement a member function comesBefore that tests whether one address comes before another when the addresses are compared by postal code. Returns false if both zipcodes are equal.
- Use the provided main.cpp to start with.
- The code creates three instances of the Address class (three objects) to test your class.
- Each object will utilize a different constructor. You will need to add the class definition and implementation.
- The comesBefore function assumes one address comes before another based on zip code alone. The test will also return false when the zipcodes are equal.
---------------------------------
In the Main.cpp
- The code should create instances of the address class to test your class.
- Each object will utilize a different constructor. You will need to add the class definition and implementation.
- The comesBefore function assumes one address comes before another based on zip code alone. The test will also return false when the zipcodes are equal.
code provided:
#include <iostream> #include <iomanip> #include <string> using namespace std; #include "address.h" int main() { address defaultAddress; address myPlace(71, "North Broadway", 19, "North white plains", "NY", 10603); address yourPlace(86, "clover Rd", " North White Plains", "NY", 10603); cout << "Comparing address:\n"; myPlace.print(); cout << "\nWith address:\n"; yourPlace.print(); if (myPlace.comesBefore(yourPlace)) cout << "\nThe first address comes before the second\n"; else cout << "\nThe first address may come after the second\n"; myPlace.setZipcode(12603); if (myPlace.comesBefore(yourPlace)) cout << "\nThe first address comes before the second\n"; else cout << "\nThe first address may come after the second\n"; //The following makes sure proper OOP are followed, will not compile otherwise const address train(16, "Washington ave N", " North White Plains", "NY", 10601); train.print(); if (train.comesBefore(yourPlace)) cout << "\nThe first address comes before the second\n"; else cout << "\nThe first address may come after the second\n"; return 0; }
----------------------------
OUTPUT SHOULD LOOK LIKE THIS:
Comparing address:
71 North Broadway, #19
NORTH WHITE PLAINS, NY 10603
With address:
86 Clover Rd
North White Plains, NY 10603
The first address comes before the second
The first address may come after the second
16 Washington ave N
White Plains, NY 10601
\The first address comes before the second
----------------
Main goal is for to create a :
- Class header: address.h
- Class Implementation: address.cpp

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

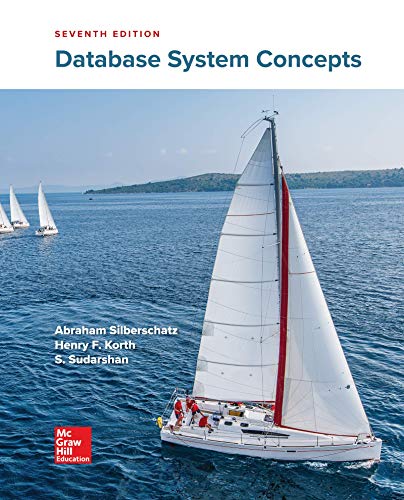
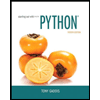
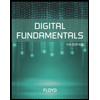
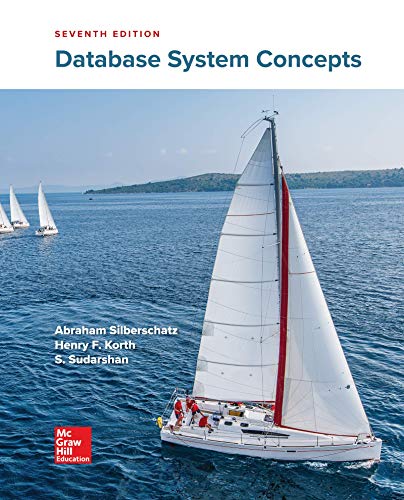
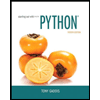
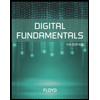
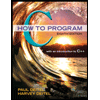
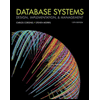
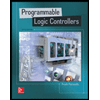