Please help with the following: Change the code from JAVA to C# public class HealthProfile { private String firstName; private String lastName; private char gender; private int day; private int month; private int year; private double height; private double weight; // Constructor public HealthProfile(String firstName, String lastName, char gender, int day, int month, int year, double height, double weight) { super(); this.firstName = firstName; this.lastName = lastName; this.gender = gender; this.day = day; this.month = month; this.year = year; this.height = height; this.weight = weight; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public char getGender() { return gender; } public void setGender(char gender) { this.gender = gender; } public int getDay() { return day; } public void setDay(int day) { this.day = day; } public int getMonth() { return month; } public void setMonth(int month) { this.month = month; } public int getYear() { return year; } public void setYear(int year) { this.year = year; } public double getHeight() { return height; } public void setHeight(double height) { this.height = height; } public double getWeight() { return weight; } public void setWeight(double weight) { this.weight = weight; } public int calculateAge() { Calendar dateOfBirth = Calendar.getInstance(); dateOfBirth.set(year, month, day); Calendar now = Calendar.getInstance(); return now.get(Calendar.YEAR) - dateOfBirth.get(Calendar.YEAR); } // This method will calculate maximumHeartRate public int maximumHeartRate() { return 220 - calculateAge(); } public double[] targetHeartRateRange() { double[] range = new double[2]; // Calculate Stating range(50 % of maximumHeartRate) range[0] = 0.5 * maximumHeartRate(); // Calculate End range(85 % of maximumHeartRate) range[1] = 0.85 * maximumHeartRate(); return range; } public double calculateBMI() { return (weight * 703)/(height * height); } public String getBMIValue() { double bmi=calculateBMI(); if(bmi < 18.5) { return "Underweight"; } else if (bmi>18.5 && bmi<24.9) { return "Normal"; } else if (bmi>25 && bmi<29.9) { return "Normal"; } else if (bmi>=30) { return "Obese"; } return "DafultValue"; //you can give any default value of your choice here if no condition meets the given criteria } @Override public String toString() { return "HealthProfile [firstName=" + firstName + ", lastName=" + lastName + ", gender=" + gender + ", Date Of Birth=" + day + "-" + month + "-" + year + ", height=" + height + ", weight=" + weight + "]"; } } package healthcare; import java.util.Scanner; public class TestHealthCare { private static Scanner sc; public static void main(String[] args) { sc = new Scanner(System.in); System.out.println("Please enter following details of the Patient"); System.out.println("First Name"); String firstName=sc.nextLine(); System.out.println("Last Name"); String lastName=sc.nextLine(); System.out.println("Gender ...... M or F ?"); char gender=sc.next().charAt(0); System.out.println("Date of Birth"); System.out.println("Day"); int day=sc.nextInt(); System.out.println("Month"); int month=sc.nextInt(); System.out.println("Year"); int year=sc.nextInt(); System.out.println("Height in inches"); double height =sc.nextDouble(); System.out.println("weight (in pounds)"); double weight =sc.nextDouble(); //Create a New Object of HealthProfile class //Calling constructor HealthProfile obj=new HealthProfile(firstName, lastName, gender, day, month, year, height, weight); //Call calculateAge int age=obj.calculateAge(); System.out.println("Patient age is "+age + " Years"); //Call maximumHeartRate int maxHeartRate=obj.maximumHeartRate(); System.out.println("Patient Maximum Heart Rate is "+maxHeartRate + " beats per minute"); //Call targetHeartRateRange double targetHeartRateRange []=obj.targetHeartRateRange(); System.out.println("Target Heart Range is "+targetHeartRateRange [0] + " - " +targetHeartRateRange [1]); double bmi=obj.calculateBMI(); System.out.println("Patient BMI is "+bmi); System.out.println("Patient BMI Value is "+obj.getBMIValue()); } }
Please help with the following: Change the code from JAVA to C#
public class HealthProfile {
private String firstName;
private String lastName;
private char gender;
private int day;
private int month;
private int year;
private double height;
private double weight;
// Constructor
public HealthProfile(String firstName, String lastName, char gender, int day, int month, int year, double height,
double weight) {
super();
this.firstName = firstName;
this.lastName = lastName;
this.gender = gender;
this.day = day;
this.month = month;
this.year = year;
this.height = height;
this.weight = weight;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public char getGender() {
return gender;
}
public void setGender(char gender) {
this.gender = gender;
}
public int getDay() {
return day;
}
public void setDay(int day) {
this.day = day;
}
public int getMonth() {
return month;
}
public void setMonth(int month) {
this.month = month;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
public int calculateAge() {
Calendar dateOfBirth = Calendar.getInstance();
dateOfBirth.set(year, month, day);
Calendar now = Calendar.getInstance();
return now.get(Calendar.YEAR) - dateOfBirth.get(Calendar.YEAR);
}
// This method will calculate maximumHeartRate
public int maximumHeartRate() {
return 220 - calculateAge();
}
public double[] targetHeartRateRange() {
double[] range = new double[2];
// Calculate Stating range(50 % of maximumHeartRate)
range[0] = 0.5 * maximumHeartRate();
// Calculate End range(85 % of maximumHeartRate)
range[1] = 0.85 * maximumHeartRate();
return range;
}
public double calculateBMI() {
return (weight * 703)/(height * height);
}
public String getBMIValue()
{
double bmi=calculateBMI();
if(bmi < 18.5)
{
return "Underweight";
}
else if (bmi>18.5 && bmi<24.9)
{
return "Normal";
}
else if (bmi>25 && bmi<29.9)
{
return "Normal";
}
else if (bmi>=30)
{
return "Obese";
}
return "DafultValue"; //you can give any default value of your choice here if no condition meets the given criteria
}
@Override
public String toString() {
return "HealthProfile [firstName=" + firstName + ", lastName=" + lastName + ", gender=" + gender + ", Date Of Birth="
+ day + "-" + month + "-" + year + ", height=" + height + ", weight=" + weight + "]";
}
}
package healthcare;
import java.util.Scanner;
public class TestHealthCare {
private static Scanner sc;
public static void main(String[] args) {
sc = new Scanner(System.in);
System.out.println("Please enter following details of the Patient");
System.out.println("First Name");
String firstName=sc.nextLine();
System.out.println("Last Name");
String lastName=sc.nextLine();
System.out.println("Gender ...... M or F ?");
char gender=sc.next().charAt(0);
System.out.println("Date of Birth");
System.out.println("Day");
int day=sc.nextInt();
System.out.println("Month");
int month=sc.nextInt();
System.out.println("Year");
int year=sc.nextInt();
System.out.println("Height in inches");
double height =sc.nextDouble();
System.out.println("weight (in pounds)");
double weight =sc.nextDouble();
//Create a New Object of HealthProfile class
//Calling constructor
HealthProfile obj=new HealthProfile(firstName, lastName, gender, day, month, year, height, weight);
//Call calculateAge
int age=obj.calculateAge();
System.out.println("Patient age is "+age + " Years");
//Call maximumHeartRate
int maxHeartRate=obj.maximumHeartRate();
System.out.println("Patient Maximum Heart Rate is "+maxHeartRate + " beats per minute");
//Call targetHeartRateRange
double targetHeartRateRange []=obj.targetHeartRateRange();
System.out.println("Target Heart Range is "+targetHeartRateRange [0] + " - " +targetHeartRateRange [1]);
double bmi=obj.calculateBMI();
System.out.println("Patient BMI is "+bmi);
System.out.println("Patient BMI Value is "+obj.getBMIValue());
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

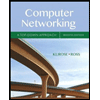
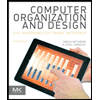
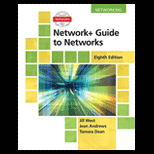
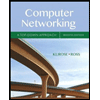
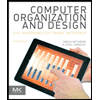
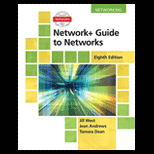
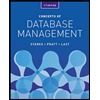
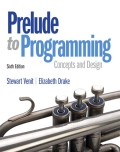
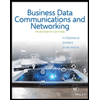