PLEASE REFER TO THE IMAGES FOR INSTRUCTIONS PLEASE USE STARTER CODE - CODE IN PYTHON3 ### starter code import random def spider_web(web_map, starting_place, destination): pass def spider_web_rec(web_map, starting_place, destination, visited): pass
PLEASE REFER TO THE IMAGES FOR INSTRUCTIONS
PLEASE USE STARTER CODE - CODE IN PYTHON3
### starter code
import random
def spider_web(web_map, starting_place, destination):
pass
def spider_web_rec(web_map, starting_place, destination, visited):
pass
def make_spider_web(num_nodes, seed=0):
if seed:
random.seed(seed)
web_map = {}
for i in range(1, num_nodes + 1):
web_map[f'Node {i}'] = []
for i in range(1, num_nodes + 1):
sample = random.sample(list(range(i, num_nodes + 1)), random.randint(1, num_nodes - i + 1))
print('sample', i, sample)
for x in sample:
if i != x:
web_map[f'Node {i}'].append(f'Node {x}')
web_map[f'Node {x}'].append(f'Node {i}')
return web_map
if __name__ == '__main__':
num_nodes, seed = [int(x) for x in input('Input num_nodes, seed: ').split(',')]
the_web = make_spider_web(num_nodes, seed)
print(spider_web(the_web, 'Node 1', f'Node {num_nodes}'))
Allowed Built-ins/Methods/etc
- Declaring and assigning variables, ints, floats, bools, strings, lists, dicts.
- Using +, -, *, /, //, %, **; +=, -=, *=, /=, //=, %=, **= where appropriate
- Comparisons ==,›, b, in
- Logical and, or, not • if/elif/else, nested if statements
- Casting int(x), str(x),float(x), (technically bool(x))
- For loops, both Pori and for each type.
- While loops
sentinel values, boolean flags to terminate while loops
- Lists, list(), indexing, i.e. try or myJist[3]
2d-lists if you want them/need them my_2d[i][j]
Append, remove o list slicing
- If you have read this section, then you know the secret word is: createous.
- String operations, concatenation +, +=, strip(), join(), upper(), lower(), isupper(), islower(), rjust(), ljust()
string slicing
- Print, with string formatting, with end= or sep=:
'{}'format(var),' %d% some_int, f-strings
Really the point is that we don't care how you format strings in Python
Ord, chr, but you won't need them this time.
- Input, again with string formatting in the prompt, casting the returned value.
- Dictionaries
- creation using dict(), or {}, copying using dict(other_dict)
- .get(value, not_found_value) method
- accessing, inserting elements, removing elements.
- Using the functions provided to you in the starter code.
- Using import with libraries and specific functions as allowed by the project/homework.
- Recursion
Forbidden Built-ins/Methods/etc
This is not a complete listing, but it includes:
- break, continue
- methods outside those permitted within allowed types
- for instance str.endswith
- list.index, list.count, etc.
- Keywords you definitely don't need: await, as, assert, async, class, except, finally, global, lambda, nonlocal, raise, try, yield
- The is keyword is forbidden, not because it's necessarily bad, but because it doesn't behave as you might expect (it's not the same as ==).
- built in functions: any, all, breakpoint, callable, classmethod, compile, exec, delattr, divmod, enumerate, filter, map, max, min, isinstance, issubclass, iter, locals, oct, next, memoryview, property, repr, reversed, round, set, setattr, sorted, staticmethod, sum, super, type, vars, zip
- If you have read this section, then you know the secret word is: argumentative.
- exit() or quit()
- If something is not on the allowed list, not on this list, then it is probably forbidden.
- The forbidden list can always be overridden by a particular problem, so if a problem allows something on this list, then it is allowed for that problem.

![Spider Webbing
Create a recursive function in a file called spider_web.py:
This is going to be a guided problem more than most of the others on this homework. This will teach you some basic
pathfinding in a network (graph) for the project. You can use this problem to build your project so l would recommend
working on this problem until you understand it.
Imagine there is a spider web:
Our goal is to find a path (not the best path, but just any path) from A to Z You see that the green path is not the
shortest but it does let us navigate from start to finish.
How can we do this?
First, we need to know A and Z, our starting and ending points. We'll pass these into our function.
I'm going to use a dictionary to represent this graph. Each node (vertex, circle) will have a name, in this case "A" and 'Z"
were the names of the nodes, but in the generated maps l'm going to use "Node 1", "Node 2", "Node 3", etc.
Here is an example web_map
web_map = {
"Node 1: ['Node 3, 'Node 2],
"Node 2: ['Node 1', 'Node 41.
"Node 3: [Node l],
Node 4: ['Node 2]
}
Nodel is connected to 2 and 3 for instance, and then also note that Node 3 is connected back to Node 1. Similariy, Node
2 is connected back to Node 1.
Then there's a connection between Node 2 and Node 4 that also goes both ways. All connections in our web will be
bi-directional.
So, in order to find the path from the start to the finish we should check if there's a path recursively through any of the
nodes connected to wherever we start.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5d4869a0-2403-4e1b-8150-f6736e06bf2f%2F7c1e1154-5238-4417-b21a-781b699d00ca%2Fujbvpuc_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

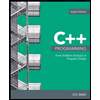
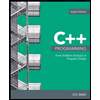