Purpose You will have an opportunity in this exercise to rewrite a method to be immutable and add contracts to all methods. Instructions Consider Bloch's non-generic Stack implementation; public class Stack { private Object[] elements; private int size = 0; private static final int DEFAULT_INITIAL_CAPACITY = 16; public Stack() { this.elements = new Object[DEFAULT_INITIAL_CAPACITY]; } public void push (Object e) { ensureCapacity(); elements[size++] = e; } public Object pop () { if (size == 0) throw new IllegalStateException("Stack.pop"); Object result = elements[--size]; elements[size] = null; // Eliminate obsolete reference return result; } private void ensureCapacity() { if (elements.length == size) { Object oldElements[] = elements; elements = new Object[2*size + 1]; System.arraycopy(oldElements, 0, elements, 0, size); } } } Rewrite Stack to be immutable. Keep the representation variables elements and size. Do the right thing with push(). Do the right thing with pop(). Get rid of anything that doesn't make sense in an immutable data type. Deliverable Elect one member of your group to submit your group's answers to the discussion forum. Note your group name/number in the subject of your post. Everyone in your group is expected to actively contribute and is responsible for the solution provided. Individual grades for this course include a component based on evaluations submitted by your peers for this activity. Please refer to the Course Schedule for due dates.
Purpose You will have an opportunity in this exercise to rewrite a method to be immutable and add contracts to all methods.
Instructions
Consider Bloch's non-generic Stack implementation;
public class Stack { private Object[] elements; private int size = 0; private static final int DEFAULT_INITIAL_CAPACITY = 16; public Stack() { this.elements = new Object[DEFAULT_INITIAL_CAPACITY]; } public void push (Object e) { ensureCapacity(); elements[size++] = e; } public Object pop () { if (size == 0) throw new IllegalStateException("Stack.pop"); Object result = elements[--size]; elements[size] = null; // Eliminate obsolete reference return result; } private void ensureCapacity() { if (elements.length == size) { Object oldElements[] = elements; elements = new Object[2*size + 1]; System.arraycopy(oldElements, 0, elements, 0, size); } } }
- Rewrite Stack to be immutable. Keep the representation variables elements and size.
- Do the right thing with push().
- Do the right thing with pop().
- Get rid of anything that doesn't make sense in an immutable data type.
Deliverable Elect one member of your group to submit your group's answers to the discussion forum. Note your group name/number in the subject of your post. Everyone in your group is expected to actively contribute and is responsible for the solution provided. Individual grades for this course include a component based on evaluations submitted by your peers for this activity. Please refer to the Course Schedule for due dates.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

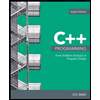
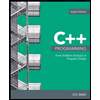