The following code is used to derive Fibonacci algorithm with this sequence: (0, 1, 1, 2, 3, 5, 8, 13, 21, …) where each number is “add” of two previous ones. int fib(int n){ if (n==0) return 0; else if (n == 1) return 1; else return fib(n−1) + fib(n−2); } Explain why the following RISC-V assembly code works, you need to explain all the details using the comments as hints? # IMPORTANT! Stack pointer must remain a multiple of 16!!!! addi x10,x10,8 # fib(8), you can change it fib: beq x10, x0, done # If n==0, return 0 addi x5, x0, 1 beq x10, x5, done # If n==1, return 1 addi x2, x2, -16 # Allocate 2 words of stack space sd x1, 0(x2) # Save the return address sd x10, 8(x2) # Save the current n addi x10, x10, -1 # x10 = n-1 jal x1, fib # fib(n-1) ld x5, 8(x2) # Load old n from the stack sd x10, 8(x2) # Push fib(n-1) onto the stack addi x10, x5, -2 # x10 = n-2 jal x1, fib # Call fib(n-2) ld x5, 8(x2) # x5 = fib(n-1) add x10, x10, x5 # x10 = fib(n-1)+fib(n-2) # Clean up: ld x1, 0(x2) # Load saved return address addi x2, x2, 16 # Pop two words from the stack done: jalr x0, 0(x1)
The following code is used to derive Fibonacci algorithm with this sequence: (0, 1, 1, 2, 3, 5, 8, 13, 21, …) where each number is “add” of two previous ones. int fib(int n){ if (n==0) return 0; else if (n == 1) return 1; else return fib(n−1) + fib(n−2); } Explain why the following RISC-V assembly code works, you need to explain all the details using the comments as hints? # IMPORTANT! Stack pointer must remain a multiple of 16!!!! addi x10,x10,8 # fib(8), you can change it fib: beq x10, x0, done # If n==0, return 0 addi x5, x0, 1 beq x10, x5, done # If n==1, return 1 addi x2, x2, -16 # Allocate 2 words of stack space sd x1, 0(x2) # Save the return address sd x10, 8(x2) # Save the current n addi x10, x10, -1 # x10 = n-1 jal x1, fib # fib(n-1) ld x5, 8(x2) # Load old n from the stack sd x10, 8(x2) # Push fib(n-1) onto the stack addi x10, x5, -2 # x10 = n-2 jal x1, fib # Call fib(n-2) ld x5, 8(x2) # x5 = fib(n-1) add x10, x10, x5 # x10 = fib(n-1)+fib(n-2) # Clean up: ld x1, 0(x2) # Load saved return address addi x2, x2, 16 # Pop two words from the stack done: jalr x0, 0(x1)
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter4: Selection Structures
Section: Chapter Questions
Problem 14PP
Related questions
Question
The following code is used to derive Fibonacci
int fib(int n){
if (n==0)
return 0;
else if (n == 1)
return 1;
else
return fib(n−1) + fib(n−2);
}
Explain why the following RISC-V assembly code works, you need to explain all the details using the comments as hints?
# IMPORTANT! Stack pointer must remain a multiple of 16!!!!
- addi x10,x10,8 # fib(8), you can change it
- fib:
- beq x10, x0, done # If n==0, return 0
- addi x5, x0, 1
- beq x10, x5, done # If n==1, return 1
- addi x2, x2, -16 # Allocate 2 words of stack space
- sd x1, 0(x2) # Save the return address
- sd x10, 8(x2) # Save the current n
- addi x10, x10, -1 # x10 = n-1
- jal x1, fib # fib(n-1)
- ld x5, 8(x2) # Load old n from the stack
- sd x10, 8(x2) # Push fib(n-1) onto the stack
- addi x10, x5, -2 # x10 = n-2
- jal x1, fib # Call fib(n-2)
- ld x5, 8(x2) # x5 = fib(n-1)
- add x10, x10, x5 # x10 = fib(n-1)+fib(n-2)
- # Clean up:
- ld x1, 0(x2) # Load saved return address
- addi x2, x2, 16 # Pop two words from the stack
- done:
- jalr x0, 0(x1)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
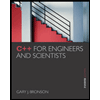
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
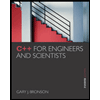
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr