The Loop Operation Let's spend a few moments writing a plan as a pseudocode. sum <- 0 number - 0 Grab the current character -> ch If ch is a digit then ● digit <-ascii-to-decimal(ch) number<- number * 10 number<- number + digit Else sum <- sum + number number <- 0 Here are some notes on implementing this plan in C++. Use str.at () to grab the current character; store it in a variable Use isdigit() to see if the character is between '0' and '9' inclusive There is no ascii-to-decimal function. Instead, subtract the character '0' from ch and store the result in the variable digit. Note that the ch has an underlying ASCII code and the codes are sequential. If ch is '0' then subtracting '0' will store the binary number in digit. If the character is '1', then subtracting '0' will leave the binary number 1. Multiply the current value of number by 10, and then add the digit. For instance, if number has the value 2 and digit has the value 5, then number * 10 -> 20 and adding 5 leaves number with 25, which is correct. When you encounter something that isn't a digit, then add the current value of number to sum. Then, set number back to 0 for the next iteration.
The Loop Operation Let's spend a few moments writing a plan as a pseudocode. sum <- 0 number - 0 Grab the current character -> ch If ch is a digit then ● digit <-ascii-to-decimal(ch) number<- number * 10 number<- number + digit Else sum <- sum + number number <- 0 Here are some notes on implementing this plan in C++. Use str.at () to grab the current character; store it in a variable Use isdigit() to see if the character is between '0' and '9' inclusive There is no ascii-to-decimal function. Instead, subtract the character '0' from ch and store the result in the variable digit. Note that the ch has an underlying ASCII code and the codes are sequential. If ch is '0' then subtracting '0' will store the binary number in digit. If the character is '1', then subtracting '0' will leave the binary number 1. Multiply the current value of number by 10, and then add the digit. For instance, if number has the value 2 and digit has the value 5, then number * 10 -> 20 and adding 5 leaves number with 25, which is correct. When you encounter something that isn't a digit, then add the current value of number to sum. Then, set number back to 0 for the next iteration.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter5: Repetition Statements
Section: Chapter Questions
Problem 9PP
Related questions
Question
Need some help turning this c++ pseudocode into working code

Transcribed Image Text:The Loop Operation
Let's spend a few moments writing a plan as a pseudocode.
sum <- 0
number - 0
Grab the current character -> ch
If ch is a digit then
digit ‹- ascii-to-decimal (ch)
number<- number * 10
number<- number + digit
●
Else
sum <- sum + number
number <- 0
Here are some notes on implementing this plan in C++.
Use str.at() to grab the current character; store it in a variable
Use isdigit() to see if the character is between '0' and '9' inclusive
There is no ascii-to-decimal function. Instead, subtract the character '0'
from ch and store the result in the variable digit. Note that the ch has an
underlying ASCII code and the codes are sequential. If ch is '0' then
subtracting '0' will store the binary number in digit. If the character is
'1', then subtracting '0' will leave the binary number 1.
Multiply the current value of number by 10, and then add the digit. For
instance, if number has the value 2 and digit has the value 5, then number
* 10 -> 20 and adding 5 leaves number with 25, which is correct.
When you encounter something that isn't a digit, then add the current value
of number to sum. Then, set number back to 0 for the next iteration.

Transcribed Image Text:string sumNums (const string& str)
{
}
string result;
int sum{0};
int num{0};
for(size_t i{0}, len{str.size()}; i < len; ++i)
{
}
Grab the current character -> ch
If ch is a digit then
digit <- ascii-to-decimal(ch)
number <- number * 10
number <- number + digit
Else
sum <- sum + number
number - 0
return result;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
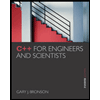
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
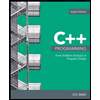
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
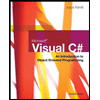
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
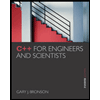
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
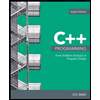
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
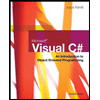
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage