The Main class, ITrip interface, and SimpleTrip class have been provided for you. The remaining classes you will have to complete on your own. The Builder is responsible for building your trip by creating several ComplexTrip and SimpleTrip objects and connecting them. Your trip should start at Home and then visit North Adams and Pittsfield. While in North Adams you will visit MASSMoCA, with a stop at the Lewitt Exhibit and the Cafe, and then you will check out MCLA. While in Pittsfield you will visit the Science Museum and then catch a Baseball Game. When your program is working correctly, your program should produce the following output: Visiting Home Visiting North Adams Visiting MASSMoCA Visiting Lewitt Exhibit Visiting Cafe Visiting MCLA Visiting Pittsfield Visiting Science Museum Visiting Baseball Game
The Main class, ITrip interface, and SimpleTrip class have been provided for you. The remaining classes you will have to complete on your own. The Builder is responsible for building your trip by creating several ComplexTrip and SimpleTrip objects and connecting them.
Your trip should start at Home and then visit North Adams and Pittsfield. While in North Adams you will visit MASSMoCA, with a stop at the Lewitt Exhibit and the Cafe, and then you will check out MCLA. While in Pittsfield you will visit the Science Museum and then catch a Baseball Game.
When your
Visiting Home Visiting North Adams Visiting MASSMoCA Visiting Lewitt Exhibit Visiting Cafe Visiting MCLA Visiting Pittsfield Visiting Science Museum Visiting Baseball Game
import java.util.*;
import java.io.*;
public class Main
{
public static void main (String[] args) throws Exception
{
ITrip e = (Builder.getInstance()).createTrip();
e.travel();
}
}
public interface ITrip
{
public void travel();
}
public class SimpleTrip implements ITrip
{
private String m_location;
public SimpleTrip(String location)
{
m_location = location;
}
@Override
public void travel()
{
System.out.printf("Visiting %s%n", m_location);
}
}
public class Builder {
public ITrip createTrip() {
ComplexTrip t1 = new ComplexTrip("Home");
ComplexTrip t2 = new ComplexTrip("North Adams");
ComplexTrip t3 = new ComplexTrip("Pittsfield");
t1.add(t2).add(t3);
ComplexTrip t4 = new ComplexTrip("MassMoCA");
ComplexTrip t5 = new ComplexTrip("MCLA");
t2.add(t4).add(t5);
ITrip t6 = new ComplexTrip("Science Museum");
ITrip t7 = new ComplexTrip("Baseball Game");
t3.add(t6).add(t7);
ITrip t8 = new ComplexTrip("Lewitt Exhibit");
ITrip t9 = new ComplexTrip("Cafe");
t4.add(t8).add(t9);
return null;
}
public static ITrip getInstance() {
// TODO Auto-generated method stub
return null;
}
import java.util.ArrayList;
import java.util.List;
public class ComplexTrip {
private List<ITrip> m_trips;
public ComplexTrip(String location) {
}
public void travel() {
super.travel();
for(ITrip t: m_trips) {
t.travel();
}
}
public ComplexTrip add(ITrip trip) {
return (null);
}
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

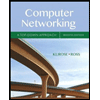
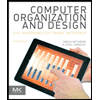
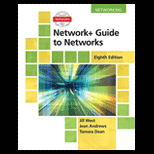
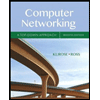
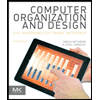
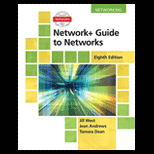
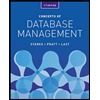
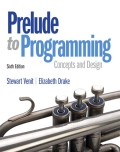
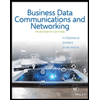