// TODO: Define a data structure // of type mq_attr to specify a // queue that can hold up to 10 // messages with the maximum message // size being 4096 bytes // The buffer used to store the message copied // copied from the shared memory char buff[MQ_MSGSIZE]; // The total number of bytes written int totalBytesWritten = 0; // The number of bytes written int bytesWritten = 0; // The return value int msqBytesRecv = -1; // Open the file for writing int fd = open(RECV_FILE_NAME, O_CREAT | O_WRONLY | O_TRUNC, 0777); // Make sure the file was opened if(fd < 0) { perror("open"); exit(1); } // TODO: Create the message queue // whose name is defined by the macro // MSQ_NAME macro above. // The queue should be configured with // parameters defined by the data // message queue data structure defined above. // The queue should have permissions of 0600. while(msqBytesRecv != 0) { // TODO: Receive the message. // Write the data from the message // into the file (represented by the // descriptor fd). Please note: // the mq_receive() function // you will use will return // the size of the message // received (in bytes). // If the size of the message // is 0, it is the sender telling // us that it has no more data to send. // Please see the sender's code for the // corresponding logic } // TODO: Deallocate the message queue } int main(int argc, char** argv) { recvFile(); return 0; } -------------------------------------------------------------------------- sender.cpp #include #include #include #include #include #include #include #include #include #include #define MSQ_NAME "/cpsc351queue" int main(int argc, char** argv) { // The file size int fileSize = -1; // The buffer used to store the message copied // copied from the shared memory char buff[4096]; // The variable to hold the message queue ID int msqid = -1; // The total number of bytes written int totalBytesRead = 0; // The number of bytes int bytesRead = 0; // Whether we are done reading bool finishedReading = false; // TODO: Define a data structure // of type mq_attr to specify a // queue that can hold up to 10 // messages with the maximum message // size being 4096 bytes // Sanity checks -- make sure the user has provided a file if(argc < 2) { fprintf(stderr, "USAGE: %s \n", argv[0]); exit(1); } // Open the file for reading int fd = open(argv[1], O_RDONLY); // Make sure the file was opened if(fd < 0) { perror("open"); exit(1); } // TODO: Gain access to the message queue // whose name is defined by the macro // MSQ_NAME macro above. We assume that // the receiver has allocated the message queue. //TODO: Loop below attempts to read the // file 4096 bytes at a time. // Modify the loop as necessary to send // each chunk of data read as message // through the message queue. You can use // 1 for the priority of the message. // Keep writing until all data has been written while((totalBytesRead < fileSize) && !finishedReading) { // Read from file to the shared memory bytesRead = read(fd, buff, 4096); // Something went wrong if(bytesRead < 0) { perror("read"); exit(1); } // We are at the end of file else if(bytesRead == 0) { // We are at the end of file finishedReading = true; } totalBytesRead += bytesRead; } // TODO: Send a message with size of 0 // to the receiver to tell it that the // transmission is done fprintf(stderr, "Sent a total of %d bytes\n", totalBytesRead); // TODO: Close the file
COMPLETE TO DO's (do not copy other responses to this question if you do ill downvote)
recv.cpp
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <mqueue.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <sys/mman.h>
#include <unistd.h>
#include <signal.h>
// The name of the shared memory segment
#define MSQ_NAME "/cpsc351queue"
// The name of the file where to save the received file
#define RECV_FILE_NAME "file__recv"
#define MQ_MSGSIZE 4096
/**
* Receive the file from the message queue
*/
void recvFile()
{
// TODO: Define a data structure
// of type mq_attr to specify a
// queue that can hold up to 10
// messages with the maximum message
// size being 4096 bytes
// The buffer used to store the message copied
// copied from the shared memory
char buff[MQ_MSGSIZE];
// The total number of bytes written
int totalBytesWritten = 0;
// The number of bytes written
int bytesWritten = 0;
// The return value
int msqBytesRecv = -1;
// Open the file for writing
int fd = open(RECV_FILE_NAME, O_CREAT | O_WRONLY | O_TRUNC, 0777);
// Make sure the file was opened
if(fd < 0)
{
perror("open");
exit(1);
}
// TODO: Create the message queue
// whose name is defined by the macro
// MSQ_NAME macro above.
// The queue should be configured with
// parameters defined by the data
// message queue data structure defined above.
// The queue should have permissions of 0600.
while(msqBytesRecv != 0)
{
// TODO: Receive the message.
// Write the data from the message
// into the file (represented by the
// descriptor fd). Please note:
// the mq_receive() function
// you will use will return
// the size of the message
// received (in bytes).
// If the size of the message
// is 0, it is the sender telling
// us that it has no more data to send.
// Please see the sender's code for the
// corresponding logic
}
// TODO: Deallocate the message queue
}
int main(int argc, char** argv)
{
recvFile();
return 0;
}
--------------------------------------------------------------------------
sender.cpp
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <sys/mman.h>
#include <mqueue.h>
#include <unistd.h>
#include <signal.h>
#define MSQ_NAME "/cpsc351queue"
int main(int argc, char** argv)
{
// The file size
int fileSize = -1;
// The buffer used to store the message copied
// copied from the shared memory
char buff[4096];
// The variable to hold the message queue ID
int msqid = -1;
// The total number of bytes written
int totalBytesRead = 0;
// The number of bytes
int bytesRead = 0;
// Whether we are done reading
bool finishedReading = false;
// TODO: Define a data structure
// of type mq_attr to specify a
// queue that can hold up to 10
// messages with the maximum message
// size being 4096 bytes
// Sanity checks -- make sure the user has provided a file
if(argc < 2)
{
fprintf(stderr, "USAGE: %s <FILE NAME>\n", argv[0]);
exit(1);
}
// Open the file for reading
int fd = open(argv[1], O_RDONLY);
// Make sure the file was opened
if(fd < 0)
{
perror("open");
exit(1);
}
// TODO: Gain access to the message queue
// whose name is defined by the macro
// MSQ_NAME macro above. We assume that
// the receiver has allocated the message queue.
//TODO: Loop below attempts to read the
// file 4096 bytes at a time.
// Modify the loop as necessary to send
// each chunk of data read as message
// through the message queue. You can use
// 1 for the priority of the message.
// Keep writing until all data has been written
while((totalBytesRead < fileSize) && !finishedReading)
{
// Read from file to the shared memory
bytesRead = read(fd, buff, 4096);
// Something went wrong
if(bytesRead < 0)
{
perror("read");
exit(1);
}
// We are at the end of file
else if(bytesRead == 0)
{
// We are at the end of file
finishedReading = true;
}
totalBytesRead += bytesRead;
}
// TODO: Send a message with size of 0
// to the receiver to tell it that the
// transmission is done
fprintf(stderr, "Sent a total of %d bytes\n", totalBytesRead);
// TODO: Close the file
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

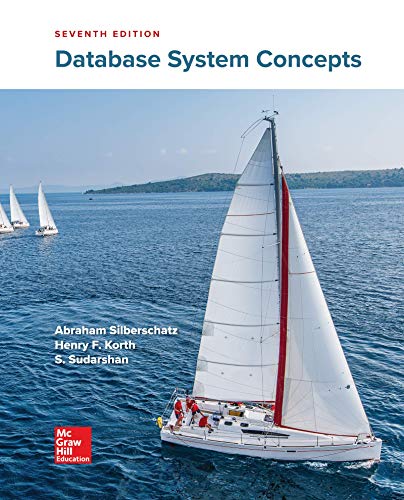
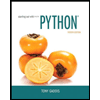
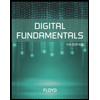
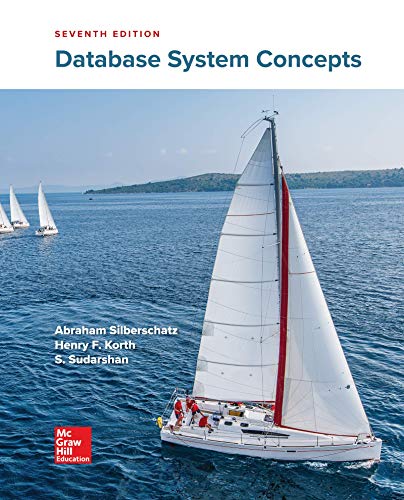
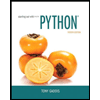
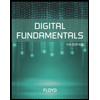
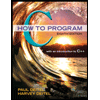
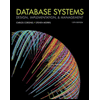
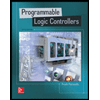