Using the attached diceType, create a class rollType that holds information for a roll of several dice simultaneously. rollType should contain the number of dice being rolled and a pointer to a dynamic array of diceType. (2) Create a client program to test your implementation that creates a dice roll whose size is based on the user's input. Step 1: Define remaining member functions Step 2: Complete test program Notes: DO NOT modify anything in diceType.h or diceTypeImp.cpp. **I just need your best guess at filling in the stub functions for this assignment and an updated test file. It doesn't have to be all that correct. I've attached the two diceType files as images. Only the rollDice ones need altering.** //diceRollType header file #ifndef DICE_ROLL_TYPE_H #define DICE_ROLL_TYPE_H #include "diceType.h" class diceRollType { public: void getResults() const; void rollDice() const; diceRollType(int = 1); ~diceRollType(); diceRollType(const diceRollType &otherDice); private: diceType *diceList; int numDice; }; //diceRollTypeImp file #include #include #include "diceRollType.h" using namespace std; void diceRollType::getResults() const { } void diceRollType::rollDice() const { } diceRollType::diceRollType(int n){ numDice = n; diceList = new diceType[numDice]; } // Copy Constructor diceRollType::diceRollType(const diceRollType &otherDice) { } diceRollType::~diceRollType(){ } //diceRollType test file #include #include "diceRollType.h" using namespace std; void PrintRoll(diceRollType dice); int main(){ int numDice; cout << "How many 6-sided dice do you want? "; cin >> numDice; diceRollType dice(numDice); dice.rollDice(); PrintRoll(dice); dice.getResults(); return 0; } void PrintRoll(diceRollType dice){ dice.getResults();
Using the attached diceType, create a class rollType that holds information for a roll of several dice simultaneously. rollType should contain the number of dice being rolled and a pointer to a dynamic array of diceType. (2) Create a client
- Step 1: Define remaining member functions
- Step 2: Complete test program
Notes:
- DO NOT modify anything in diceType.h or diceTypeImp.cpp.
**I just need your best guess at filling in the stub functions for this assignment and an updated test file. It doesn't have to be all that correct. I've attached the two diceType files as images. Only the rollDice ones need altering.**
//diceRollType header file
#ifndef DICE_ROLL_TYPE_H
#define DICE_ROLL_TYPE_H
#include "diceType.h"
class diceRollType {
public:
void getResults() const;
void rollDice() const;
diceRollType(int = 1);
~diceRollType();
diceRollType(const diceRollType &otherDice);
private:
diceType *diceList;
int numDice;
};
//diceRollTypeImp file
#include <iostream>
#include <iomanip>
#include "diceRollType.h"
using namespace std;
void diceRollType::getResults() const {
}
void diceRollType::rollDice() const {
}
diceRollType::diceRollType(int n){
numDice = n;
diceList = new diceType[numDice];
}
// Copy Constructor
diceRollType::diceRollType(const diceRollType &otherDice) {
}
diceRollType::~diceRollType(){
}
//diceRollType test file
#include <iostream>
#include "diceRollType.h"
using namespace std;
void PrintRoll(diceRollType dice);
int main(){
int numDice;
cout << "How many 6-sided dice do you want? ";
cin >> numDice;
diceRollType dice(numDice);
dice.rollDice();
PrintRoll(dice);
dice.getResults();
return 0;
}
void PrintRoll(diceRollType dice){
dice.getResults();
}



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

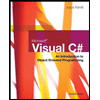
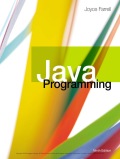
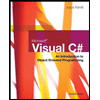
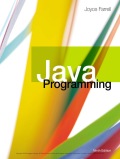