write a C program to manage 10 hotel rooms using C structures to develop a hotel management system. Every room should have the following details: room number, status (1 for reserved and 0 for vacant), customer details (username, last name, email address, address, and telephone number), check-in date, and check-out date. Your code should have a menu for these services: User input Functions Transaction type?: B or b input all customer data Check in and check out date Book a room, user can book a room. Room should be vacant otherwise a message says “Room already booked” Transaction type?: V or v room number?: room number View customer details, prints the all-customer details in specified room. Transaction type?: E or e room number?: room number Edit customer details, user can only edit information in booked room Transaction type?: C or c room number?: room number Check-out is only allowed if a room is booked. This function should return the status of the room to vacant. Additionally, it should print the user's bill for that room, calculated based on the number of days stayed (using a day rate of $100) between the check-in and check-out dates Transaction type?: R or r Print report. a user can print the information Transaction type?: E or e Exit program You should use c structure to implement your hotel management system. All the services mentioned above should be implemented as C functions. Use the following template to write your code. #include #include // Structure to store customer details // Structure to represent a hotel room // Function to book a room void bookRoom(struct Room hotel[], int roomNumber) { } // Function to view customer details void viewCustomerDetails(struct Room hotel[], int roomNumber) { } // Function to edit customer details void editCustomerDetails(struct Room hotel[], int roomNumber) { } // Function to check out void checkOut(struct Room hotel[], int roomNumber) { } // Function to print a report void printReport(struct Room hotel[]) { } int main() { return 0; }
write a C
management system.
Every room should have the following details: room number, status
(1 for reserved and 0 for vacant), customer details (username, last name, email address,
address, and telephone number), check-in date, and check-out date.
Your code should have a menu for these services:
User input Functions
Transaction
type?: B or b
input all customer
data
Check in and check
out date
Book a room, user can book a room. Room should be vacant otherwise
a message says “Room already booked”
Transaction
type?: V or v
room
number?: room
number
View customer details, prints the all-customer details in specified
room.
Transaction
type?: E or e
room number?:
room number
Edit customer details, user can only edit information in booked room
Transaction
type?: C or c
room number?:
room number
Check-out is only allowed if a room is booked. This function should
return the status of the room to vacant. Additionally, it should print the
user's bill for that room, calculated based on the number of days stayed
(using a day rate of $100) between the check-in and check-out dates
Transaction
type?: R or r
Print report. a user can print the information
Transaction
type?: E or e
Exit program
You should use c structure to implement your hotel management system.
All the services mentioned above should be implemented as C functions.
Use the following template to write your code.
#include <stdio.h>
#include <string.h>
// Structure to store customer details
// Structure to represent a hotel room
// Function to book a room
void bookRoom(struct Room hotel[], int roomNumber) {
}
// Function to view customer details
void viewCustomerDetails(struct Room hotel[], int roomNumber)
{
}
// Function to edit customer details
void editCustomerDetails(struct Room hotel[], int roomNumber)
{
}
// Function to check out
void checkOut(struct Room hotel[], int roomNumber) {
}
// Function to print a report
void printReport(struct Room hotel[]) {
}
int main() {
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

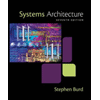
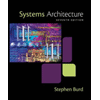