Write a code that will print the deleted number class LinkedList { static Node head; static class Node { int data; Node next; Node(int d) { data = d; next = null; } } void duplicateremove() { Node ptr1 = null, ptr2 = null, dup = null; ptr1 = head; while (ptr1 != null && ptr1.next != null) { ptr2 = ptr1; while (ptr2.next != null) { if (ptr1.data == ptr2.next.data) { ptr2.next = ptr2.next.next; System.gc(); } else { ptr2 = ptr2.next; } } ptr1 = ptr1.next; } } void printList(Node node) { while (node != null) { System.out.print(node.data + " "); node = node.next; } } public static void main(String[] args) { LinkedList list = new LinkedList(); list.head = new Node(3); list.head.next = new Node(3); list.head.next.next = new Node(5); list.head.next.next.next = new Node(6); System.out.println( "before removing duplicates : \n "); list.printList(head); list.duplicateremove(); System.out.println(""); System.out.println( "after removing duplicates : \n "); list.printList(head); } }
Write a code that will print the deleted number
class LinkedList {
static Node head;
static class Node {
int data;
Node next;
Node(int d)
{
data = d;
next = null;
}
}
void duplicateremove()
{
Node ptr1 = null, ptr2 = null, dup = null;
ptr1 = head;
while (ptr1 != null && ptr1.next != null) {
ptr2 = ptr1;
while (ptr2.next != null) {
if (ptr1.data == ptr2.next.data) {
ptr2.next = ptr2.next.next;
System.gc();
}
else {
ptr2 = ptr2.next;
}
}
ptr1 = ptr1.next;
}
}
void printList(Node node)
{
while (node != null) {
System.out.print(node.data + " ");
node = node.next;
}
}
public static void main(String[] args)
{
LinkedList list = new LinkedList();
list.head = new Node(3);
list.head.next = new Node(3);
list.head.next.next = new Node(5);
list.head.next.next.next = new Node(6);
System.out.println(
"before removing duplicates : \n ");
list.printList(head);
list.duplicateremove();
System.out.println("");
System.out.println(
"after removing duplicates : \n ");
list.printList(head);
}
}

Step by step
Solved in 3 steps with 1 images

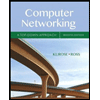
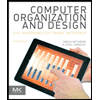
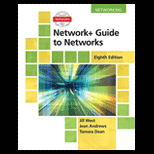
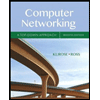
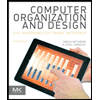
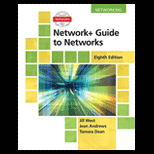
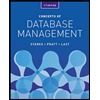
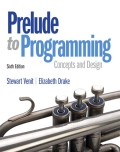
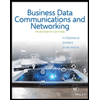