write a python code Create the Testing class that does the following: a-Use the constructor to create an instance of Cylinder class cy with radius of 5 and height of 10. use the following code:
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
write a python code
Create the Testing class that does the following:
a-Use the constructor to create an instance of Cylinder class cy with radius of 5 and height of 10.
use the following code:
class Circular_Shape_3D:
radius = 0.0 #double value
#Constructor
def __init(self,radius):
self.radius = radius
#Get function
def getRadius(self):
return self.radius
#Set function
def setRadius(self, radius):
self.radius = radius
#Print function
def Print(self):
print("Circular_Shape_3D")
print("Radius:", self.radius)
class Cylinder(Circular_Shape_3D):
#Radius value is already present in super class Circular_Shape_3D
height = 0.0 #double value
#Constructor
def __init(self,radius,height):
super().__init__(radius)
self.height = height
#Get function
def getHeight(self):
return self.height
#Set function
def setHeight(self, height):
self.height = height
#Function compute the area of cylinder
def area(self):
r = self.getRadius()
a = 2 * 3.1416 * r * self.height + 2 * 3.1416 * r * r
return a
#Function compute the volume of cylinder
def volume(self):
r = self.getRadius()
v = 3.1416 * r * r * self.height
return v
def Print(self):
super().Print()
print("Height:", self.height)
print("Area:", self.area())
print("Volume:", self.volume())

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

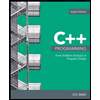
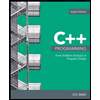