You must write a script that prints a table of inches and their equivalent centimeter values. You convert inches to centimeters using the formula
You must write a script that prints a table of inches and their equivalent centimeter values. You convert inches to centimeters using the formula
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:Specification
You must write a script that prints a table of inches and their cquivalent centimcter values.
You convert inches to centimeters using the formula
cm = inches * 2.54
Centimeter values must be integers.
At the top of the table should appear the labels "Inches" and "Centimeters",
Under these labels should be a line of dashes.
The centimeter values should align with the "Centimeters" label.
In other words, it will work in exactly the same way as
But it will do this in a different way.
The script will create the table using 3 functions which you must write.
• print_conversion_table
• print_table_header
• print_table_line
These functions will be called using test code which you will find below.
print_conversion_table
This function must have the following header
def print_conversion_table(min, max):
This function will will print the conversion table.
It will call print_table_header to print the labels at the top of the table.
It will use a for loop to call print_line to print each line of the table.
This function will be called by the test code you will find below.
print_table_header
This function must have the following header
def print_table_header():
This should print the labels "Inches" and "Centimeters".
It should then print a line of dashes.
print_table_line
This function must have the following header
def print_table_line(inches):
This function will print one line of the conversion table.
It will convert the inches value into centimeters.
Then it will print the inches and centimeters,
The centimeter values should align with the "Centimeter" label.
See the example below.
Script for this assignment
Open an a text editor and create the file
You can use the editor built into IDLE or a program like Sublime.
Test Code
Your hw6.py file must contain the following test code at the bottom of the file
min = int(input ("Minimum inches: "))
max = int(input ("Maximum inches: "))
print()
print_conversion_table(min, max)
This code is needed to call the functions that you will create for this assignment.

Transcribed Image Text:Suggestions
Write this program in a step-by-step fashion using the technique of incremental development.
In other words, write a bit of code, test it, make whatever changes you need to get it working, and go on to the
next step.
1. Copy only the function headers for the three functions listed above into your script file.
Under each header, write pass.
pass is a special Python statement that does nothing.
You use it in situations like this.
Make sure each pass statement is indented.
Run the script.
Fix any errors you find.
2. Remove the pass statement from print_conversion_table.
Print the parameters min and max.
Copy the test code above to the bottom of your script.
Run the script.
Fix any errors you find.
3. Replace the print statement in print_conversion_table with a call to print_table_header.
Remove the pass statement from print_table_header.
Add a print statement for the labels at the top of the table.
You will need to put a Tab character between the two words so everything will line up.
You do this with an escape sequence.
Add another print statement for the line of dashes.
Run the script.
After the input prompts you should see
Inches
Centimeters
Fix any errors you find.
4. In print_conversion_table add a for loop that loops from min to max.
The name of the loop variable should be inches.
Inside the loop print the value of inches.
Run the script.
Fix any errors you find.
5. In print_conversion_table replace the print statement inside the for loop with a call to
print_table_line.
Inside print_table_line remove the pass statement.
Replace it with a statement that prints the value of the parameter inches.
Run the script.
The output should be the same as above but now it comes from print_table_line.
Fix any errors you find.
6. Remove the print statement from print_table_line.
In it's place you need to write an assignment statement that sets the value of the local variable cm.
Use the same formula you used in homework 5 to get the value for cm.
Print the values of both inches and cm.
Run the script.
Fix any errors you find.
7. Change the print statement in print_table_line so it prints a single string with a Tab character
between inches and cm.
You will have to use the conversion function str to change both variables to a string.
The values should line up with the labels for each column.
Run the script.
Fix any errors you find.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
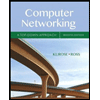
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
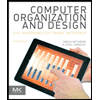
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
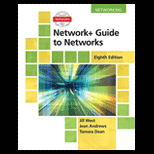
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
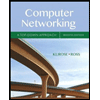
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
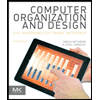
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
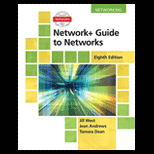
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
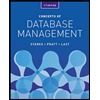
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
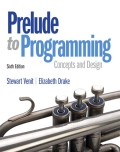
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
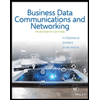
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY