Hi, help me with this problem, please. Also, please follow the skeleton code provided to find the output. Here's the skeleton code: package graph; import java.util.*; public class Graph2 { public int n; //number of vertice public int[][] A; //the adjacency matrix public Graph2 () { n = 0; A = null; } public Graph2 (int _n, int[][] _A) { this.n = _n; this.A = _A; } public int prim (int r) { } /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub int n = 9; int A[][] = { {0, 4, 0, 0, 0, 0, 0, 8, 0}, {4, 0, 8, 0, 0, 0, 0, 11, 0}, {0, 8, 0, 7, 0, 4, 0, 0, 2}, {0, 0, 7, 0, 9, 14, 0, 0, 0}, {0, 0, 0, 9, 0, 10, 0, 0, 0}, {0, 0, 4, 14, 10, 0, 2, 0, 0}, {0, 0, 0, 0, 0, 2, 0, 1, 6}, {8, 11, 0, 0, 0, 0, 1, 0, 7}, {0, 0, 2, 0, 0, 0, 6, 7, 0} }; Graph2 g = new Graph2(n, A); System.out.println(g.prim(0)); } } Here is the prim function: package graph; public class PrimNode implements Comparable { public int id; public int key; public PrimNode(int id, int key) { this.id = id; this.key = key; } public int compareTo(PrimNode o) { return (this.key - o.key); } }
Hi, help me with this problem, please. Also, please follow the skeleton code provided to find the output. Here's the skeleton code: package graph; import java.util.*; public class Graph2 { public int n; //number of vertice public int[][] A; //the adjacency matrix public Graph2 () { n = 0; A = null; } public Graph2 (int _n, int[][] _A) { this.n = _n; this.A = _A; } public int prim (int r) { } /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub int n = 9; int A[][] = { {0, 4, 0, 0, 0, 0, 0, 8, 0}, {4, 0, 8, 0, 0, 0, 0, 11, 0}, {0, 8, 0, 7, 0, 4, 0, 0, 2}, {0, 0, 7, 0, 9, 14, 0, 0, 0}, {0, 0, 0, 9, 0, 10, 0, 0, 0}, {0, 0, 4, 14, 10, 0, 2, 0, 0}, {0, 0, 0, 0, 0, 2, 0, 1, 6}, {8, 11, 0, 0, 0, 0, 1, 0, 7}, {0, 0, 2, 0, 0, 0, 6, 7, 0} }; Graph2 g = new Graph2(n, A); System.out.println(g.prim(0)); } } Here is the prim function: package graph; public class PrimNode implements Comparable { public int id; public int key; public PrimNode(int id, int key) { this.id = id; this.key = key; } public int compareTo(PrimNode o) { return (this.key - o.key); } }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
Hi, help me with this problem, please. Also, please follow the skeleton code provided to find the output.
Here's the skeleton code:
package graph; import java.util.*; public class Graph2 { public int n; //number of vertice public int[][] A; //the adjacency matrix public Graph2 () { n = 0; A = null; } public Graph2 (int _n, int[][] _A) { this.n = _n; this.A = _A; } public int prim (int r) { } /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub int n = 9; int A[][] = { {0, 4, 0, 0, 0, 0, 0, 8, 0}, {4, 0, 8, 0, 0, 0, 0, 11, 0}, {0, 8, 0, 7, 0, 4, 0, 0, 2}, {0, 0, 7, 0, 9, 14, 0, 0, 0}, {0, 0, 0, 9, 0, 10, 0, 0, 0}, {0, 0, 4, 14, 10, 0, 2, 0, 0}, {0, 0, 0, 0, 0, 2, 0, 1, 6}, {8, 11, 0, 0, 0, 0, 1, 0, 7}, {0, 0, 2, 0, 0, 0, 6, 7, 0} }; Graph2 g = new Graph2(n, A); System.out.println(g.prim(0)); } }Here is the prim function:
package graph; public class PrimNode implements Comparable<PrimNode> { public int id; public int key; public PrimNode(int id, int key) { this.id = id; this.key = key; } public int compareTo(PrimNode o) { return (this.key - o.key); } }![Instructions. You are provided one skeleton program named Graph2.java.
The source files are available on Canvas in a folder named HW9. Please modify
the skeleton code to solve the following tasks.
• Task 1
Lecture 18.
Implement the prim(int r) function as discussed in
- Note: You should return an integer that indicates the total weight
of the minimum spanning tree.
Hint 1: We use an adjacent matrix to represent the graph. If
Ali][i] = 0, it means there is no edge between the i-th and j-th node.
If A(i][j] > 0, then it means the weight of the edge between the i-th
and j-th node.
- Hint 2:
Java PriorityQueue API. Also find some tutorials on Java Pri-
orityQueue to understand it better.
To learn how to use Priority Queue in Java, google
Hint 3: In order to associate each node with a weight and place it
into the queue, you may need to create another class. Check ListN-
ode.java and LinkedList.java in HW3 to get an example of auxiliary
class.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0b1fac41-8ac9-4abd-b414-8218fcd8a359%2F2c45ac8f-2458-4c6a-ac85-db46b0c81a15%2F387ud1k_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Instructions. You are provided one skeleton program named Graph2.java.
The source files are available on Canvas in a folder named HW9. Please modify
the skeleton code to solve the following tasks.
• Task 1
Lecture 18.
Implement the prim(int r) function as discussed in
- Note: You should return an integer that indicates the total weight
of the minimum spanning tree.
Hint 1: We use an adjacent matrix to represent the graph. If
Ali][i] = 0, it means there is no edge between the i-th and j-th node.
If A(i][j] > 0, then it means the weight of the edge between the i-th
and j-th node.
- Hint 2:
Java PriorityQueue API. Also find some tutorials on Java Pri-
orityQueue to understand it better.
To learn how to use Priority Queue in Java, google
Hint 3: In order to associate each node with a weight and place it
into the queue, you may need to create another class. Check ListN-
ode.java and LinkedList.java in HW3 to get an example of auxiliary
class.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
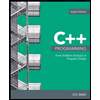
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
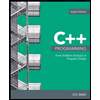
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning