You are tasked with developing a comprehensive program to oversee the performance of four cashiers in a retail setting. The program should handle cash register discrepancies, calculate overall totals, and compute averages for each cashier as well as the entire group. The data for each cashier includes cash on hand and reported sales for multiple shifts over a reporting period. Here are the specific requirements for your Java program: 1. Data Storage: • Use a class to represent each cashier, storing data such as cash on hand, reported sales, and the number of shifts worked. • Ensure that cash on hand and reported sales are stored as 'double data types. • Use varying quantities of data for each cashier to simulate real-world scenarios. 2. Array Sizes: • Utilize the length property of arrays to determine the array sizes dynamically. For the first two employees, store the array sizes using a byte variable. For the other two employees, use the length property directly. 3. Computing Discrepancies: • Calculate the discrepancies (over, under, or on target) for each cashier's registers. • Keep track of the discrepancies in pennies and store them in an array of int data type. 4. Overall Totals and Averages: • Implement a loop to compute the total and average for each cashier. • Maintain a separate array for the total amount off and the average for each cashier. 5. Registers and Pointers: • Use pointers to navigate through the arrays, incrementing them appropriately for each shift and cashier. Initialize counters using integer data types. 6. Registers and Watches: • Utilize 'eax', 'ebx, ecx', and 'edx' registers for processing. • Add these registers to the Watch to monitor their values during debugging. 7. Division and Averages: • When computing averages, use the cdq' command before using the 'idiv' command for signed division. • Ensure that only the eax', 'ebx, ecx', and 'edx registers are used in your processing. 8. Overall Total and Average: • Calculate the overall total and average for all cashiers. • Divide by the number of transactions overall, not just the number of cashiers. 9. Java-Specific Considerations: • Use the appropriate Java syntax and constructs for loops, arrays, and classes. • Implement exception handling where necessary. 10. Testing: • Test your program with various sets of data to ensure accuracy and reliability. • Verify that the computed overall total and average align with the expected results. Your solution should demonstrate proficiency in Java programming, proper use of arrays, classes, and registers, as well as adherence to the specified requirements. Ensure that your program is well-organized and includes comments to explain your logic and approach.
You are tasked with developing a comprehensive program to oversee the performance of four cashiers in a retail setting. The program should handle cash register discrepancies, calculate overall totals, and compute averages for each cashier as well as the entire group. The data for each cashier includes cash on hand and reported sales for multiple shifts over a reporting period. Here are the specific requirements for your Java program: 1. Data Storage: • Use a class to represent each cashier, storing data such as cash on hand, reported sales, and the number of shifts worked. • Ensure that cash on hand and reported sales are stored as 'double data types. • Use varying quantities of data for each cashier to simulate real-world scenarios. 2. Array Sizes: • Utilize the length property of arrays to determine the array sizes dynamically. For the first two employees, store the array sizes using a byte variable. For the other two employees, use the length property directly. 3. Computing Discrepancies: • Calculate the discrepancies (over, under, or on target) for each cashier's registers. • Keep track of the discrepancies in pennies and store them in an array of int data type. 4. Overall Totals and Averages: • Implement a loop to compute the total and average for each cashier. • Maintain a separate array for the total amount off and the average for each cashier. 5. Registers and Pointers: • Use pointers to navigate through the arrays, incrementing them appropriately for each shift and cashier. Initialize counters using integer data types. 6. Registers and Watches: • Utilize 'eax', 'ebx, ecx', and 'edx' registers for processing. • Add these registers to the Watch to monitor their values during debugging. 7. Division and Averages: • When computing averages, use the cdq' command before using the 'idiv' command for signed division. • Ensure that only the eax', 'ebx, ecx', and 'edx registers are used in your processing. 8. Overall Total and Average: • Calculate the overall total and average for all cashiers. • Divide by the number of transactions overall, not just the number of cashiers. 9. Java-Specific Considerations: • Use the appropriate Java syntax and constructs for loops, arrays, and classes. • Implement exception handling where necessary. 10. Testing: • Test your program with various sets of data to ensure accuracy and reliability. • Verify that the computed overall total and average align with the expected results. Your solution should demonstrate proficiency in Java programming, proper use of arrays, classes, and registers, as well as adherence to the specified requirements. Ensure that your program is well-organized and includes comments to explain your logic and approach.
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 14RQ
Related questions
Question
Alert - don't use any AI platform to generate answer and don't try to copy others'work otherwise I'll reduce rating.

Transcribed Image Text:You are tasked with developing a comprehensive program to oversee the
performance of four cashiers in a retail setting. The program should handle cash
register discrepancies, calculate overall totals, and compute averages for each
cashier as well as the entire group. The data for each cashier includes cash on hand
and reported sales for multiple shifts over a reporting period.
Here are the specific requirements for your Java program:
1. Data Storage:
• Use a class to represent each cashier, storing data such as cash on hand,
reported sales, and the number of shifts worked.
• Ensure that cash on hand and reported sales are stored as 'double data types.
• Use varying quantities of data for each cashier to simulate real-world scenarios.
2. Array Sizes:
• Utilize the length property of arrays to determine the array sizes dynamically.
• For the first two employees, store the array sizes using a byte variable.
• For the other two employees, use the length property directly.
3. Computing Discrepancies:
• Calculate the discrepancies (over, under, or on target) for each cashier's registers.
• Keep track of the discrepancies in pennies and store them in an array of int
data type.
4. Overall Totals and Averages:
• Implement a loop to compute the total and average for each cashier.
• Maintain a separate array for the total amount off and the average for each
cashier.
5. Registers and Pointers:
• Use pointers to navigate through the arrays, incrementing them appropriately for
each shift and cashier.
• Initialize counters using integer data types.
6. Registers and Watches:
• Utilize eax', 'ebx, ecx, and edx registers for processing.
• Add these registers to the Watch to monitor their values during debugging.
7. Division and Averages:
• When computing averages, use the cdq command before using the 'idiv'
command for signed division.
Ensure that only the eax', 'ebx, ecx', and 'edx registers are used in your
processing.
8. Overall Total and Average:
• Calculate the overall total and average for all cashiers.
• Divide by the number of transactions overall, not just the number of cashiers.
9. Java-Specific Considerations:
• Use the appropriate Java syntax and constructs for loops, arrays, and classes.
• Implement exception handling where necessary.
10. Testing:
• Test your program with various sets of data to ensure accuracy and reliability.
• Verify that the computed overall total and average align with the expected results.
Your solution should demonstrate proficiency in Java programming, proper use of
arrays, classes, and registers, as well as adherence to the specified requirements.
Ensure that your program is well-organized and includes comments to explain your
logic and approach.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
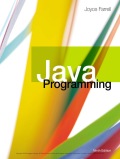
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
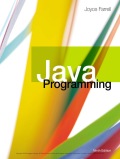
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT