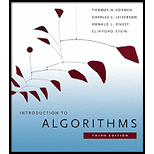
(a)
To explain the nodes that needs to change to insert a key k or delete a node y .
(a)

Explanation of Solution
For the insertion of the key k in the tree it first checks the ancestors of the tress then it traverses the children of that ancestor. The insertion causes some misbalancing situation so the tree needs to maintain the property of tree by changing the nodes.
The deletion of a node y is done by checking the children of the node y and the successor of the node y so that their children are placed into suitable node after deletion of the parent node y.
So, for the efficient execution of the operations it needs some changing in the ancestors of the node to accept the change by the insertion or deletion.
(b)
To gives an
(b)

Explanation of Solution
The algorithm for insertion in persistent tree is given below:
PERSISTENT-TREE-INSERT( T,k )
if
end if.
while
if
else
end if.
end while.
if
else
end if.
end.
The algorithm is used to insert a key k into the node. The algorithm checks the value of the nodes of the tree and then finds the suitable place to insert the key by comparing the value of key with the nodes of the tree.
The algorithm performs the insertion in such a way that it consider the root and start visiting the node of similar depth then it copy the nodes and then make new version of the tree with old and key k .
(c)
To explain the space required for the implementation of PERSISTENT-TREE-INSERT.
(c)

Explanation of Solution
The algorithm consists of the while loop that runs
The iterations of the algorithm run in constant time as they are just simple iteration or conditional iteration. For the purpose of storing all the h it needs some additional space equals to h .
Thus, the space and time required for the algorithm is depends upon the h and equals to
(d)
To prove that the PERSISTENT-TREE-INSERT would require
(d)

Explanation of Solution
The insertion of the a key into tree by using above algorithm is based on the fact that it first copy the original tree then it find the appropriate position for key and insert the key by addition key on the original tree.
The node that points to the appropriate position will copy and then it copy the ancestor of the node and so on until it copy all the connected nodes of the tree
The copying of a node takes constant time and one storage space for each node but there are total n nodes in the tree so it takes total time of n .
Thus, the algorithm takes total of
(e)
To explain the worse-case running time and space is
(e)

Explanation of Solution
The insertion or deletion of the key into tree cause dis-balancing in the tree so it needs to maintain the tree by changing the ancestors and children of the ancestors.
The algorithm allocates space of
For the finding the appropriate position it need to compare and check the values of node and for worse case it is equal to total nodes in
The operation is considering the ancestor of the node where the key is going to be inserted or deleted, the node with their children and ancestors are considered that is another subtree and for the worse case the height of that subtree is equal to the height of tree that is
Therefore, the total space and time requires for the operation is equals to
Want to see more full solutions like this?
Chapter 13 Solutions
Introduction to Algorithms
- In a workspace array, a double linked list may be implemented by utilising just one index next. To locate the backward linkages, we do not need to maintain a distinct field back in the nodes that make up the workspace array. The goal is to insert a member workspace[current] rather than the index of the next item on the list into workspace[current]. difference equals the index of the entry after the current entry less the index of the item before it. We must additionally keep two pointers to subsequent nodes in the list, the current index and the prior index of the node right before current in the linked list.To find the next entry of the list, we calculate workspace[current].difference + previous; Similarly, to find the entry preceding previous, we calculate current − workspace[previous].difference;arrow_forwardImplements clone which duplicates a list. Pay attention, because if there are sublists, they must be duplicated as well. Understand the implementation of the following function, which recursively displays the ids of each node in a list Develop your solution as follows: First copy the nodes of the current list (self) Create a new list with the copied nodes Loop through the nodes of the new list checking the value field If this field is also a list (use isinstance as in the show_ids function) then it calls clone on that list and substitutes the value. Complete the code: def L4(*args,**kwargs): class L4_class(L): def clone(self): def clone_node(node): return <... YOUR CODE HERE ...> r = <... YOUR CODE HERE...> return r return L4_class(*args,**kwargs)arrow_forwardCreate a circular queue using fixed size array. Each index of your queue should store the execution timeof the process. Your task is to provide the implementation of following functions: constructor should receive the array size and create a dynamic array. enqueue (function to insert the element into a queue) dequeue (function to remove the element from the queue) isEmpty (function to check whether the queue is empty or not) isFull (function to check whether the queue is full or not) getLength (function to return the total elements in the queue) getSize (function to return the size of queue) reSize (This function should receive the new size as a parameter and resize the queue as per thenew size) getRear (function to return the last elements from the queue) getMin (function to return the index with least execution time) getMax (function to return the index with maximum execution time) getCountofSimilar (function to return the processes with same execution time) show (this…arrow_forward
- How does a link-based implementation of the List differ from an array-based implementation? Select one: a. All of these b. A link-based implementation does not need to shift entries over to make room when adding a new entry to the List c. A link-based implementation is sized dynamically so it takes up only the memory to hold the current entries d. A link-based implementation does not need to shift entries up to remove a gap when removing an entry from the Listarrow_forwardWrite and implement a recursive version of the binary search algorithm. Also, write a version of the sequential search algorithm that can be applied to sorted lists. Add this operation to the class orderedArrayListType for array-based lists. Moreover, write a test program to test your algorithm.arrow_forwardA double linked list may be implemented in a workspace array by utilising only one index next. That is, we do not need to retain a distinct field back in the workspace array nodes to detect the backward linkages. The goal is to add a member workspace[current] into workspace[current] rather than the index of the next element on the list. The difference is the index of the next item less the index of the entry preceding current. We must additionally keep two pointers to subsequent nodes in the list, the current index and the prior index of the node right before current in the linked list.To find the next entry of the list, we calculate workspace[current].difference + previous; Similarly, to find the entry preceding previous, we calculate current − workspace[previous].difference;arrow_forward
- Two sorted lists have been created, one implemented using a linked list (LinkedListLibrary class) and the other implemented using the built-in Vector class (VectorLibrary class). Each list contains 100 books (title, ISBN number, author), sorted in ascending order by ISBN number. Complete main() by inserting a new book into each list using the respective LinkedListLibrary and VectorLibrary InsertSorted() functions and outputting the number of book copy operations the computer must perform to insert the new book. Each InsertSorted() returns the number of book copy operations the computer performs. Ex: If the input is: Their Eyes Were Watching God 9780060838676 Zora Neale Hurston the output is: Number of linked list book copy operations: 1 Number of vector book copy operations: 95 Which list do you think will require the most operations? Why?Main.cpp #include "LinkedListLibrary.h"#include "VectorLibrary.h"#include "BookNode.h"#include "Book.h"#include <fstream>#include…arrow_forwardCSE LAB The Government of United States of America has decided to issue new currency notes with special protection features to so as to commemorate a great mathematician. They have decided to issue notes summing up to N and all the sums from 1 to N should only made by selecting some of the notes in only one unique way. With n = 5 the sets {1,1,1,1,1}, {1,2,2}, {1,1,3} are valid. Your task is to design a C++ code that output the solution in one line. Sample Input - 100 Sample Output - 3arrow_forwardd. In implementing a Queue using an array, a problem might arise if the Queue is implemented in such a way that items in the Queue are inserted at the next available location and removed from the next leading position, but such that, once deleted, the emptied space is unused. The problem that arises is one where there is free space still in the array, but it is not usable because it is not at the end. Demonstrate this problem with a Queue that is stored in an array of size 5 for the following instructions. Next, explain how you might resolve this problem. Queue q constructor takes 5 as the size of the array = new Queue(5); // assume the Queue q.enqueue(3); q.enqueue(4); q.enqueue(1); q.dequeue( ); q.dequeue( ); q.enqueue(6); q.enqueue(5); q.dequeue( ); // at this point, // there are only 2 item2 in the queue q.enqueue(7); // this enqueue can not occur, why??arrow_forward
- Suppose that you have an array A. A = {2, 3, -5, -8, 6, -1}. Propose a dynamic programming algorithm that checks whether there is a subset with total sum of elements equal to zero. Your algorithm should display the elements of each subset whenever it finds them. Implement your algorithm with Python and explain it in your report file.arrow_forwardWhy is it giving me an error and what do I have to change? PYTHON # Problem 2# Implement a hashtable using an array. Your implementation should include public methods for insertion, deletion, and# search, as well as helper methods for resizing. The hash table is resized when the max chain length becomes greater# than 3 during insertion of a new item. You will be using linear chaining technique for collision resolution. Assume# the key to be an integer and use the hash function h(k) = k mod m where m is the size of the hashtable. You can use# python list methods in your implementation of the chain or you can also use your linked list implementation from# coding assignment 2, problem 1. You can make necessary changes to __hashtable initialization in the __init__ method# if you are using your linked list implementation. The provided code uses python lists for the __hashtable variable. class HashTableChain: def __init__(self, size=10): # Initialize the hashtable with the given…arrow_forwardIn C++, develop an algorithm that adds the value val to a queue object Q. The queue is represented using an array data of size s. The algorithm should assume that the queue is not full. The most recently added item is at index r (rear), and the least recently added item is at index f (front). If the queue is empty, r = f = -1.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
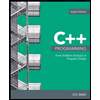