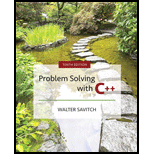
Consider a graphics system that has classes for various figures—rectangles, squares, triangles, circles, and so on. For example, a rectangle might have data members for height, width, and center point, while a square and circle might have only a center point and an edge length orradius, respectively. In a well-designed system, these would be derived from a common class, Figure. You are to implement such a system. The class Figure is the base class. You should add only Rectangle and Triangle classes derived from Figure. Each class has stubs for member functions erase and draw. Each of these member functions outputs amessage telling what function has been called and what the class of the calling object is. Since these are just stubs, they do nothing more than output this message. The member function center calls the erase and draw functions to erase and redraw the figure at the center. Since you have only stubs for erase and draw, center will not do any “cantering” but will call the member functions erase and draw. Also add an output message in the member function center that announces that center is being called. The member functions should take no arguments.
There are three parts to this project:
a. Write the class definitions using no virtual functions. Compile andtest.
b. Make the base class member functions virtual. Compile and test.
c. Explain the difference in results.
For a real example, you would have to replace the definition of each of these member functions with code to do the actual drawing. You will be asked to do this in
Use the following main function for all testing:
//This program tests Programming Project 5. #include <iostream> #include "figure.h" #include "rectangle.h" #include "triangle.h" using std::cout; int main( ) { Triangle tri; tri.draw( ); cout<< "\nDerived class Triangle object calling center( ).\n"; tri.center( ); //Calls draw and center Rectangle rect; rect.draw( ); cout<< "\nDerived class Rectangle object calling center().\n"; rect.center( ); //Calls draw and center return 0; } |

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++ (10th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Concepts Of Programming Languages
Introduction to Programming Using Visual Basic (10th Edition)
Starting Out with Python (4th Edition)
Starting out with Visual C# (4th Edition)
- In this problem you will implement a simple library system based on object-oriented approach (using inheritance, polymorphism and abstract classes). At the library we have items and people are allowed to borrow them for a while or use them in the library premises. We have 3 types of items at the library. Books, Magazines and Compact Discs (CDs). All items have a unique number called serial number, shelf number that the item put on that shelf and shelf index, the index of the item at the shelf. Items at the library have additional different properties changing to item type. For example books have name, publisher name, and author name. CDs have title property and magazines have name, publisher properties. But people are allowed to borrow only books and CDs, but not magazines. Suppose that two kinds of people using library items. These are students and academic staff. Students can borrow only one item at a time, and academic stuffs can borrow at most 3 items at the same time. Also people…arrow_forwardDesign and implement an object-oriented program for displaying a deck of cards. Each card will have a value ranging in order from 1 for an ace, 2 for a two, and so on up to and including 11 for a jack, 12 for a queen, and 13 for the king. The focus of the exercise is to create three classes that represent a card, deck, and hand using composition.arrow_forwardWrite a class named Person with data attributes for a person’s name, address, and telephone number. Provide accessors/getters and mutators/setters for each attribute. Write a displayPerson() to print out the attributes of the Person. Next, write a class named Customer that is a subclass of the Person class. The Customer class should have a data attribute for a customer number, and a Boolean data attribute indicating whether the customer wishes to be on a mailing list. Provide accessors/getters and mutators/setters for each attribute. Write a display customer() to print out the attributes of the Customer. Demonstrate an instance of the Customer class in a simple program. All your programs will be modular. It will have the main() method that is executed first. Submit code that has no syntax error. And test your code, so as to not have logic errors. Each program must contain your name in the comments section and the date you created the code.arrow_forward
- Now we want to create an Object oriented System for a shopping mall. It has Items with name, manufacturer and price. Provide constructors. Getters, setters, toString methods. We add several items to Store. For that create a store class having name and arraylist of items, Provide Constructors, getters/setters and toString(), in addition you have to provide a method add which add new item to the array. The main function that does these things looks as follows: Public static void main(Strings[] args) { Item shampoo2(“pantene”, “Procter & Gamble”,100), noodles(“Noodles”, “knorr”, 50); // add further items. Store abcSuperStore; abcSuperStore.add(shampoo2); abcSuperStore.add(noodles); … abcSuperStore.sale(shampoo2); a bcSuperStore.sale(noodles);arrow_forwardYour task is to implement the Name class. This class represents a person's name. It has three instance variables representing the first, last and middle name (all are string variables). A character instance variable representing a separator (to be used for printing purposes) is also part of the class. The only valid separators we can have are a comma (,), a dash (-), and a pound symbol (#). In addition, a nickname instance variable (string) keeps track of the person's nickname (if any). The output have to match with the image I provided. Please don’t use chegg solution, need a different coding style. Write code in JavaScriptarrow_forwardYour task is to implement the Name class. This class represents a person's name. It has three instance variables representing the first, last and middle name (all are string variables). A character instance variable representing a separator (to be used for printing purposes) is also part of the class. The only valid separators we can have are a comma (,), a dash (-), and a pound symbol (#). In addition, a nickname instance variable (string) keeps track of the person's nickname (if any). The output have to match with the image I provided. Don’t use cheggarrow_forward
- Write an abstract class Student that includes the following hidden attributes: id(int), name(String), major(String) and grade(double), then create setters and getters for them. Write two classes that inherited from Student: ItStudent and ArtStudent. The grade for ItStudent is calculated as: grade = mid*0.30 + project*0.30 + final*0.40 and the grade for ArtStudent is calculated as: grade = mid*0.40 + report*0.10 + final*0.50. Crate main class that achieve the following: a. Declare and initialize an array of five Student objects from both ItStudent and ArtStudent classes. b. Sort the Student objects by grade in descending order using functions. c. Save the sorted objects into text file.arrow_forwardNow we want to create an Object oriented System for a shopping mall. It has Items with name, manufacturer and price. Provide constructors. Getters, setters, toString methods. We add several items to Store. For that create a store class having name and array of items, Provide Constructors, getters/setters and toString(), in addition you have to provide a method add which add new item to the array.arrow_forwardDraw the UML class diagrams for the following classes: An abstract Java class called Person that has the following attributes: a String called idNumber a Date called dateOfBirth representing the date of birth. a String for name A class called VaccineRecord with the following attributes: an enum called type of VaccineType a Date called date a String called batchCode A class called Patient that extends the Person class and has the following attributes and behaviours: an ArrayList that contains VaccineRecord objects called vaccinationRecord a public method called vaccinate that takes a VaccineRecord with the following signature: public void vaccinate(VaccineRecord vaccineRecord); a public boolean method called isVaccinated that returns whether or not the Patient has had a vaccine. A MedicalPractitioner class that extends the Person class and has the following attributes and behaviours: a String called licenseCode a public method called vaccinatePatient that takes as a…arrow_forward
- Draw the UML class diagrams for the following classes: An abstract Java class called Person that has the following attributes: a String called idNumber a Date called dateOfBirth representing the date of birth. a String for name A class called VaccineRecord with the following attributes: an enum called type of VaccineType a Date called date a String called batchCode A class called Patient that extends the Person class and has the following attributes and behaviours: an ArrayList that contains VaccineRecord objects called vaccinationRecord a public method called vaccinate that takes a VaccineRecord with the following signature: public void vaccinate(VaccineRecord vaccineRecord); a public boolean method called isVaccinated that returns whether or not the Patient has had a vaccine. A MedicalPractitioner class that extends the Person class and has the following attributes and behaviours: a String called licenseCode a public method called vaccinatePatient that takes as a…arrow_forwardDraw the UML class diagrams for the following classes: An abstract Java class called Person that has the following attributes: a String called idNumber a Date called dateOfBirth representing the date of birth. a String for name A class called VaccineRecord with the following attributes: an enum called type of VaccineType a Date called date a String called batchCode A class called Patient that extends the Person class and has the following attributes and behaviours: an ArrayList that contains VaccineRecord objects called vaccinationRecord a public method called vaccinate that takes a VaccineRecord with the following signature: public void vaccinate(VaccineRecord vaccineRecord); a public boolean method called isVaccinated that returns whether or not the Patient has had a vaccine. A MedicalPractitioner class that extends the Person class and has the following attributes and behaviours: a String called licenseCode a public method called vaccinatePatient that takes as a…arrow_forwardPrograming language is Java. Define a class Person that represents a person. People have a name, an age, anda phone number. Since people always have a name and an age, your class shouldhave a constructor that has those as parameters. Define a toString() method whichdisplays all of the relevant information using a format like this: “Jim Lahey - Age 57- Phone 9025555555”.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
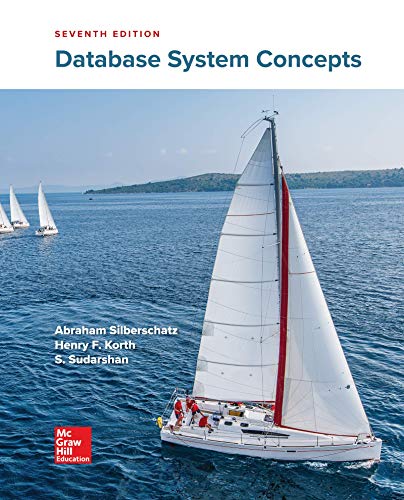
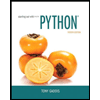
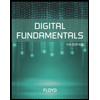
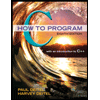
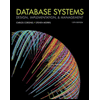
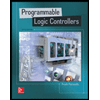