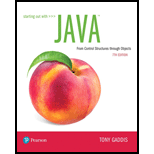
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
7th Edition
ISBN: 9780134802213
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 7, Problem 1PC
Rainfall Class
Write a RainFall class that stores the total rainfall for each of 12 months into an array of doubles. The
- the total rainfall for the year
- the average monthly rainfall
- the month with the most rain
- the month with the least rain
Demonstrate the class in a complete program.
Input Validation: Do not accept negative numbers for monthly rainfall figures.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Rainfall Type
Create a RainFall class that keeps the total rainfall in an array of doubles for each of the 12 months.
Methods in the programme should return the following values:
• the total annual rainfall; • the average monthly rainfall
• the wettest month of the year
• the month with the least amount of rain
Demonstrate the class in its entirety.
Validation of Input:
Accepting negative amounts for monthly rainfall data is not permitted.
Exercise 6 - Loaded Coin Simulation
Make a new class in the Lab2 project called LoadedCoinSim that simulates the toss of a
loaded coin. A coin is loaded when it is constructed such that it is more likely to land on one
value than another. Assume the probability the coin will land head is HEAD_PROB, and declare
that as a constant in your program. Initially, use a value of 0.75, which means 75% chance the
coin will show heads. Given this probability, simulate ten coin tosses and displaying the result.
At the end, display the number of times the coin showed heads.
The output should appear like this:
Toss 1: HEADS
Toss 2: HEADS
Toss 3: HEADS
Toss 4: TAILS
Toss 5: HEADS
Toss 6: HEADS
Toss 7: HEADS
Toss 8: HEADS
Toss 9: TAILS
Toss 10: HEADS
Number of heads
= 8
Sultan Qaboos University
Department of Computer Science
COMP2202: Fundamentals of Object Oriented Programming
Assignment # 2 (Due 5 November 2022 @23:59)
The purpose of this assignment is to practice with java classes and objects, and arrays.
Create a NetBeans/IntelliJ project named HW2_YourId to develop a java program as explained below.
Important: Apply good programming practices:
1- Provide comments in your code.
2- Provide a program design
3-
Use meaningful variables and constant names.
4- Include your name, university id and section number as a comment at the beginning of your code.
5- Submit to Moodle the compressed file of your entire project (HW1_YourId).
1. Introduction:
In crowded cities, it's very crucial to provide enough parking spaces for vehicles. These are usually multistory
buildings where each floor is divided into rows and columns. Drivers can park their cars in exchange for some
fees. A single floor can be modeled as a two-dimensional array of rows and columns. A…
Chapter 7 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Ch. 7.1 - Write statements that create the following arrays:...Ch. 7.1 - Whats wrong with the following array declarations?...Ch. 7.1 - Prob. 7.3CPCh. 7.1 - Prob. 7.4CPCh. 7.1 - Prob. 7.5CPCh. 7.1 - Prob. 7.6CPCh. 7.1 - Prob. 7.7CPCh. 7.1 - Prob. 7.8CPCh. 7.2 - Prob. 7.9CPCh. 7.2 - Prob. 7.10CP
Ch. 7.2 - A program has the following declaration: double[]...Ch. 7.2 - Look at the following statements: int[] a = { 1,...Ch. 7.3 - Prob. 7.13CPCh. 7.3 - Write a method named zero, which accepts an int...Ch. 7.6 - Prob. 7.15CPCh. 7.7 - Recall that we discussed a Rectangle class in...Ch. 7.10 - Prob. 7.17CPCh. 7.11 - What value in an array does the selection sort...Ch. 7.11 - How many times will the selection sort swap the...Ch. 7.11 - Prob. 7.20CPCh. 7.11 - Prob. 7.21CPCh. 7.11 - If a sequential search is performed on an array,...Ch. 7.13 - What import statement must you include in your...Ch. 7.13 - Write a statement that creates an ArrayList object...Ch. 7.13 - Write a statement that creates an ArrayList object...Ch. 7.13 - Prob. 7.26CPCh. 7.13 - Prob. 7.27CPCh. 7.13 - Prob. 7.28CPCh. 7.13 - Prob. 7.29CPCh. 7.13 - Prob. 7.30CPCh. 7.13 - Prob. 7.31CPCh. 7 - In an array declaration, this indicates the number...Ch. 7 - Each element of an array is accessed by a number...Ch. 7 - The first subscript in an array is always. a. 1 b....Ch. 7 - The last subscript in an array is always. a. 100...Ch. 7 - Array bounds checking happens. a. when the program...Ch. 7 - This array field holds the number of elements that...Ch. 7 - Prob. 7MCCh. 7 - This search algorithm repeatedly divides the...Ch. 7 - Prob. 9MCCh. 7 - When initializing a two-dimensional array, you...Ch. 7 - Prob. 11MCCh. 7 - To delete an item from an ArrayList object, you...Ch. 7 - To determine the number of items stored in an...Ch. 7 - True or False: java does not allow a statement to...Ch. 7 - True or False: An arrays sitze declarator can be a...Ch. 7 - Prob. 16TFCh. 7 - True or False: The subscript of the last element...Ch. 7 - Prob. 18TFCh. 7 - True or False: The Java compiler does not display...Ch. 7 - Prob. 20TFCh. 7 - True or False: The first size declarator in the...Ch. 7 - Prob. 22TFCh. 7 - Prob. 23TFCh. 7 - int[] collection = new int[-20];Ch. 7 - Prob. 2FTECh. 7 - Prob. 3FTECh. 7 - Prob. 4FTECh. 7 - Prob. 5FTECh. 7 - The variable names references an integer array...Ch. 7 - The variables numberArray1 and numberArray2...Ch. 7 - Prob. 3AWCh. 7 - In a program you need to store the populations of...Ch. 7 - In a program you need to store the identification...Ch. 7 - Prob. 6AWCh. 7 - Prob. 7AWCh. 7 - Prob. 8AWCh. 7 - Prob. 9AWCh. 7 - Prob. 10AWCh. 7 - Prob. 11AWCh. 7 - Prob. 1SACh. 7 - Prob. 2SACh. 7 - Prob. 3SACh. 7 - Prob. 4SACh. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 7SACh. 7 - Prob. 8SACh. 7 - Prob. 9SACh. 7 - Rainfall Class Write a RainFall class that stores...Ch. 7 - Payroll Class Write a Payroll class that uses the...Ch. 7 - Charge Account Validation Create a class with a...Ch. 7 - Charge Account Modification Modify the charge...Ch. 7 - Prob. 5PCCh. 7 - Drivers License Exam The local Drivers License...Ch. 7 - Magic 8 Ball Write a program that simulates a...Ch. 7 - Grade Book A teacher has five students who have...Ch. 7 - Grade Book Modification Modify the grade book...Ch. 7 - Average Steps Taken A Personal Fitness Tracker is...Ch. 7 - Array Operations Write a program with an array...Ch. 7 - Prob. 12PCCh. 7 - Sorted List of 1994 Gas Prices Note: This...Ch. 7 - Name Search If you have downloaded this books...Ch. 7 - Population Data If you have downloaded this books...Ch. 7 - World Series Champions If you have downloaded this...Ch. 7 - 2D Array Operations Write a program that creates a...Ch. 7 - Prob. 18PCCh. 7 - Trivia Game In this programming challenge, you...Ch. 7 - Prob. 20PC
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Consider the adage Never ask a question for which you do not want the answer. a. Is following that adage ethica...
Using MIS (10th Edition)
Describe the purpose of the access key attribute and how it supports accessibility.
Web Development and Design Foundations with HTML5 (8th Edition)
In Python, you cannot write functions that accept multiple arguments
Starting Out with Python (4th Edition)
The following questions are intended as a guide to the ethical/social/legal issues associated with the field of...
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Show a snippet of PHP code for disconnecting from the database. Explain the meaning of the code.
Database Concepts (7th Edition)
Suppose the insertion sort as presented in Figure 5.11 was applied to the list Gene, Cheryl, Alice, and Brenda....
Computer Science: An Overview (12th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please submit the pseudocode of your program for your project below. Need a class which will contain: Student Name Student Id Student Grades (an array of 3 grades) A constructor that clears the student data (use -1 for unset grades) Get functions for items a, b, and c, average, and letter grade Set functions for items a, n, and c Note that the get and set functions for Student grades need an argument for the grade index. Need another class which will contain: An Array of Students (1 above) A count of number of students in use You need to create a menu interface that allows you to: Add new students Enter test grades Display all the students with their names, ids, test grades, average, and letter grade Exit the program Add comments and use proper indentation. Nice Features: I would like that system to accept a student with no grades, then later add one or more grades, and when all grades are entered, calculate the final average or grade. I would like the system to display the…arrow_forwardAssignment:The BankAccount class models an account of a customer. A BankAccount has the followinginstance variables: A unique account id sequentially assigned when the Bank Account is created. A balance which represents the amount of money in the account A date created which is the date on which the account is created.The following methods are defined in the BankAccount class: Withdraw – subtract money from the balance Deposit – add money to the balance Inquiry on:o Balanceo Account ido Date createdarrow_forwardAssignment: Enhancing Dice Roll Stats Calculator Program Introduction: The project involves creating a program to roll pairs of dice, gathering statistics on the outcomes. Probability differs in the roll of two dice compared to a single die due to varied combinations. Dice Roll Series: User greeted with "Welcome to the Dice Roll Stats Calculator!" and prompted to specify rolls. Java classes: DiceRoller, Indicator, Validator. DiceRoller: Arrays: rollSeries (captures results), statIndicators (stores statistical indicators). Constructor rolls dice as per user request. Methods collect stats and print the report. Indicator class: Contains dicePairTotal and dicePairCount. Displaying Results: Sort statIndicators array before display. Indicator objects sortable by dicePairCount (implements Comparable). Example output:-----------------------dicepair rolltotal count percent---- ------ --------7 5 50%10 2 20%4 2 20%2 1 10% Tasks:…arrow_forward
- # Coding - Simulate a robot Write a program that simulates the movements of a robot. The robot can have three possible movements: turn right turn left advance The robot is placed on a hypothetical infinite grid, facing a particular direction (north, east, south, or west) at a set of `{x,y}` coordinates,e.g., `{3,8}`, with coordinates increasing to the north and east. Create a Class `Robot` that contains a method `execute` which given a number of instructions will calculate the robot's new position, and the the direction in which it is pointing. ## Example The letter-string "RAALAL" means: Turn right Advance twice Turn left Advance once Turn left yet again Say a robot starts at `{7, 3}` facing north. Running this stream of instructions should leave it at `{9, 4}` facing west. ## Inputs and Outputs The argument of the `execute` is `string` in the format `X Y BEARING COMMANDS`. The method should return a one-liner `string` in the format `X Y BEARING`. In the…arrow_forwardJava - Access Specifiers Create a class named Circle that has attributes radius, area, and circumference and make the attributes private. Make a public method that sets the radius and a method that prints all attributes. Ask the user input for radius. Note: Use the PI from the math functions Inputs A line containing an integer 10 Sample Output Radius: 20 Area: 1256.64 Circumference: 125.66arrow_forwardJAVA PROGRAM Chapter 7. PC #1. Rainfall Class Write a RainFall class that stores the total rainfall for each of 12 months into an array of doubles. The program should have methods that return the following: • the total rainfall for the year • the average monthly rainfall • the month with the most rain • the month with the least rain Demonstrate the class in a complete program. Main class name: RainFall (no package name) NOTE, THE MAIN CLASS NAME needs to be Rainfall as mentioned above AND IT HAS TO PASS ALL THE TEST CASES WHEN I UPLOAD IT TO HYPERGRADE. THANK YOU Enter the rainfall amount for month 1:\n1.2ENTEREnter the rainfall amount for month 2:\n2.3ENTEREnter the rainfall amount for month 3:\n3.4ENTEREnter the rainfall amount for month 4:\n5.1ENTEREnter the rainfall amount for month 5:\n1.7ENTEREnter the rainfall amount for month 6:\n6.5ENTEREnter the rainfall amount for month 7:\n2.5ENTEREnter the rainfall amount for month 8:\n3.3ENTEREnter the…arrow_forward
- Ag 1- Random Prime Generator Add a new method to the Primes class called genRandPrime. It should take as input two int values: 1owerBound and upperBound. It should return a random prime number in between TowerBound (inclusive) and upperBound (exclusive) values. Test the functionality inside the main method. Implementation steps: a) Start by adding the method header. Remember to start with public static keywords, then the return type, method name, formal parameter list in parenthesis, and open brace. The return type will be int. We will have two formal parameters, so separate those by a comma. b) Now, add the method body code that generates a random prime number in the specified range. The simplest way to do this is to just keep trying different random numbers in the range, until we get one that is a prime. So, generate a random int using: int randNum = lowerBound + gen.nextInt(upperBound); Then enter put a while loop that will keep going while randNum is not a prime number - you can…arrow_forwardQuadratic equations The form of a quadratic equation is ax2 + bx + c = 0. Write a program that solves a quadratic equation in all cases, including when both roots are complex numbers. Remember that the solutions are x = (-b + sqrt(b2 -4ac))/2a and (-b - sqrt(b2 -4ac))/2a For this you will need to set up the following classes: Complex: Encapsulates a complex number. ComplexPair: Encapsulates a pair of complex numbers. Quadratic: Encapsulates a quadratic equation. – Assume the coefficients are all of type int for this example. SolveEquation: Contains the main method. (I give this to you it is shown below) Along with the usual constructors, accessors and mutators you will need the following additional methods: In the Complex class a method called isReal to determine whether a complex object is real or not returning a boolean value. In the ComplexPair class a method bothIdentical that determines if both complex numbers are identical, returning a boolean value. In the…arrow_forwardCharge Account Validation Using Java programming Create a class with a method that accepts a charge account number as its argument. The method should determine whether the number is valid by comparing it to the following list of valid charge account numbers:5658845 4520125 7895122 8777541 8451277 13028508080152 4562555 5552012 5050552 7825877 12502551005231 6545231 3852085 7576651 7881200 4581002These numbers should be stored in an array. Use a sequential search to locate the number passed as an argument. If the number is in the array, the method should return true, indicating the number is valid. If the number is not in the array, the method should return false, indicating the number is invalid.Write a program that tests the class by asking the user to enter a charge account number. The program should display a message indicating whether the number is valid or invalid.arrow_forward
- Make Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forwardMake Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forwardQuestion Language: JAVA Keep Guessing Write a program that plays a number-guessing game to guess a secret number randomly generated within the range 1 and 10. The user will get as many numbers of tries as he/she needs to guess the number. Tell the users if their guess is right or wrong and if their guess is wrong let them try to guess the number again. You must generate the secret number using the Java Random class. When the user guesses a number he/she will have to take an input of his guessed number from the keyboard. The user will have to take as many inputs as needed to guess the correct secret-number. That means the code will keep looping as long as the guess is different from the secret number. Use a while loop to prompt the user to guess again if the guess is wrong. Create a class named NumberGuessingGame which will contain the main method, write all your code in the main method. Sample input and output: Suppose the random number generated is 6. I have chosen a…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
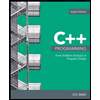
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
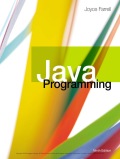
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
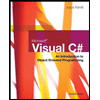
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
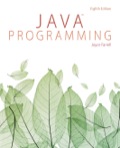
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Java random numbers; Author: Bro code;https://www.youtube.com/watch?v=VMZLPl16P5c;License: Standard YouTube License, CC-BY