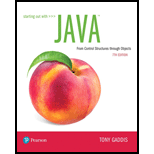
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
7th Edition
ISBN: 9780134802213
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 9, Problem 8AW
Look at the following string:
“cookies>milk>fudge:cake:ice cream”
Write code using the String class’s split method that can be used to extract the following tokens from the string: cookies, milk, fudge, cake, and ice cream.
Expert Solution & Answer

Learn your wayIncludes step-by-step video

schedule07:09
Students have asked these similar questions
Welcome Assignment
### welcome_assignment_answers
### Input - All eight questions given in the assignment.
### Output - The right answer for the specific question.
def welcome_assignment_answers(question):
# The student doesn't have to follow the skeleton for this assignment.
# Another way to implement it is using "case" statements similar to C.
if question == "Are encoding and encryption the same? - Yes/No":
answer = "The student should type the answer here"
elif question == "Is it possible to decrypt a message without a key? - Yes/No":
answer = "The student should type the answer here"
return (answer)
# Complete all the questions.
if __name__ == "__main__":
# use this space to debug and verify that the program works
debug_question = "Are encoding and encryption the same? - Yes/No"
print(welcome_assignment_answers(debug_question))
As you can see, the first two questions are already in the skeleton code. Please follow the first two questions…
Parking Charges Program in java
Write a program that works out the amount a person has to pay for parking in a car park in a tourist town. If they say they are disabled, they are told it is free. Otherwise they enter the number of hours as a whole number (1-8) that they wish to park as well as whether they have an “I live locally” badge or are an old age pensioner both of which leads to a discount. The program tells them the cost to park.
The calculation is done in this program as follows. If they are disabled it is free. Otherwise ... Take number of hours they wish to park and give a basic charge:
• • • •
1 hour: 3.00 pounds 2-4 hours: 4.00 pounds 5-6 hours: 4.50 pounds 7-8 hours: 5.50 pounds
Next modify the resulting charge based on whether they are local or not:
•
If local: subtract 1 pound
If OAP: subtract 2 poundsYour program MUST include methods including ones that
- asks for the hours and returns the basic charge.
- asks whether they live locally /…
Question
Language: JAVA
Keep Guessing
Write a program that plays a number-guessing game to guess a secret number randomly generated within the range 1 and 10. The user will get as many numbers of tries as he/she needs to guess the number. Tell the users if their guess is right or wrong and if their guess is wrong let them try to guess the number again.
You must generate the secret number using the Java Random class. When the user guesses a number he/she will have to take an input of his guessed number from the keyboard. The user will have to take as many inputs as needed to guess the correct secret-number. That means the code will keep looping as long as the guess is different from the secret number. Use a while loop to prompt the user to guess again if the guess is wrong.
Create a class named NumberGuessingGame which will contain the main method, write all your code in the main method.
Sample input and output: Suppose the random number generated is 6.
I have chosen a…
Chapter 9 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Ch. 9.2 - Prob. 9.1CPCh. 9.2 - Write an if statement that displays the word digit...Ch. 9.2 - Prob. 9.3CPCh. 9.2 - Write a loop that asks the user, Do you want to...Ch. 9.2 - Prob. 9.5CPCh. 9.2 - Write a loop that counts the number of uppercase...Ch. 9.3 - Prob. 9.7CPCh. 9.3 - Modify the method you wrote for Checkpoint 9.7 so...Ch. 9.3 - Look at the following declaration: String cafeName...Ch. 9.3 - Prob. 9.10CP
Ch. 9.3 - Prob. 9.11CPCh. 9.3 - Prob. 9.12CPCh. 9.3 - Prob. 9.13CPCh. 9.3 - Look at the following code: String str1 = To be,...Ch. 9.3 - Prob. 9.15CPCh. 9.3 - Assume that a program has the following...Ch. 9.4 - Prob. 9.17CPCh. 9.4 - Prob. 9.18CPCh. 9.4 - Prob. 9.19CPCh. 9.4 - Prob. 9.20CPCh. 9.4 - Prob. 9.21CPCh. 9.4 - Prob. 9.22CPCh. 9.4 - Prob. 9.23CPCh. 9.4 - Prob. 9.24CPCh. 9.5 - Prob. 9.25CPCh. 9.5 - Prob. 9.26CPCh. 9.5 - Look at the following string:...Ch. 9.5 - Prob. 9.28CPCh. 9.6 - Write a statement that converts the following...Ch. 9.6 - Prob. 9.30CPCh. 9.6 - Prob. 9.31CPCh. 9 - The isDigit, isLetter, and isLetterOrDigit methods...Ch. 9 - Prob. 2MCCh. 9 - The startsWith, endsWith, and regionMatches...Ch. 9 - The indexOf and lastIndexOf methods are members of...Ch. 9 - Prob. 5MCCh. 9 - Prob. 6MCCh. 9 - Prob. 7MCCh. 9 - Prob. 8MCCh. 9 - Prob. 9MCCh. 9 - Prob. 10MCCh. 9 - To delete a specific character in a StringBuilder...Ch. 9 - Prob. 12MCCh. 9 - This String method breaks a string into tokens. a....Ch. 9 - These static final variables are members of the...Ch. 9 - Prob. 15TFCh. 9 - Prob. 16TFCh. 9 - True or False: If toLowerCase methods argument is...Ch. 9 - True or False: The startsWith and endsWith methods...Ch. 9 - True or False: There are two versions of the...Ch. 9 - Prob. 20TFCh. 9 - Prob. 21TFCh. 9 - Prob. 22TFCh. 9 - Prob. 23TFCh. 9 - int number = 99; String str; // Convert number to...Ch. 9 - Prob. 2FTECh. 9 - Prob. 3FTECh. 9 - Prob. 4FTECh. 9 - The following if statement determines whether...Ch. 9 - Write a loop that counts the number of space...Ch. 9 - Prob. 3AWCh. 9 - Prob. 4AWCh. 9 - Prob. 5AWCh. 9 - Modify the method you wrote for Algorithm...Ch. 9 - Prob. 7AWCh. 9 - Look at the following string:...Ch. 9 - Assume that d is a double variable. Write an if...Ch. 9 - Write code that displays the contents of the int...Ch. 9 - Prob. 1SACh. 9 - Prob. 2SACh. 9 - Prob. 3SACh. 9 - How can you determine the minimum and maximum...Ch. 9 - Prob. 1PCCh. 9 - Prob. 2PCCh. 9 - Prob. 3PCCh. 9 - Prob. 4PCCh. 9 - Prob. 5PCCh. 9 - Prob. 6PCCh. 9 - Check Writer Write a program that displays a...Ch. 9 - Prob. 8PCCh. 9 - Prob. 9PCCh. 9 - Word Counter Write a program that asks the user...Ch. 9 - Sales Analysis The file SalesData.txt, in this...Ch. 9 - Prob. 12PCCh. 9 - Alphabetic Telephone Number Translator Many...Ch. 9 - Word Separator Write a program that accepts as...Ch. 9 - Pig Latin Write a program that reads a sentence as...Ch. 9 - Prob. 16PCCh. 9 - Lottery Statistics To play the PowerBall lottery,...Ch. 9 - Gas Prices In the student sample program files for...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
For each of the following activities, give a PEAS description of the task environment and characterize it in te...
Artificial Intelligence: A Modern Approach
What output will be produced by the following code, when embedded in a complete program? int extra = 2; if (ext...
Problem Solving with C++ (9th Edition)
Write a line of Java code that will give the name ANSWER to the int value 42. In other words, make answer a nam...
Absolute Java (6th Edition)
What is an infinite loop?
Starting out with Visual C# (4th Edition)
This is a number that identifies an item in a list. a. element b. index c. bookmark d. identifier
Starting Out with Python (4th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Rolling Dice Simulator [Java Assignment]Objective: Create a Java program that rolls two dice and displays the results. The program should have two Java classes: one for a single die and another for a pair of dice. Assignment Details: User Input: Ask the user to specify the number of sides they want on each die. Ensure that the user's input is within a reasonable range. Dice Rolling: Simulate rolling the dice using Math.random() based on the user's chosen number of sides. Display the sum of the values rolled, e.g., "5 + 3 = 8." Special Combinations: If the dice roll results in combinations of 2, 7, or 12, print special messages: "1 + 1 = 2 snake eyes!" "3 + 4 = 7 craps!" "6 + 6 = 12 box cars!" Main Method: In the main method, create a pair of dice, roll them, and display the results. Allow the user to decide whether to continue rolling the dice or exit the program. Additional Features: You are welcome to add more features or enhancements to the program if desired.…arrow_forward// LeftOrRight.cpp - This program calculates the total number of left-handed and right-handed // students in a class. // Input: L for left-handed; R for right handed; X to quit. // Output: Prints the number of left-handed students and the number of right-handed students. #include <iostream> #include <string> using namespace std; int main() { string leftOrRight = ""; // L or R for one student. int rightTotal = 0; // Number of right-handed students. int leftTotal = 0; // Number of left-handed students. // This is the work done in the housekeeping() function cout << "Enter an L if you are left-handed, a R if you are right-handed or X to quit: "; cin >> leftOrRight; // This is the work done in the detailLoop() function // Write your loop here. // This is the work done in the endOfJob() function // Output number of left or right-handed students. cout << "Number of left-handed students:…arrow_forwardPart III Write a method that takes a String value from user and parses the given value to double by using Wrapper classes.arrow_forward
- 9. Trivia Game In this programming exercise, you will create a simple trivia game for two players. The program will work like this: Starting with player 1, each player gets a turn at answering 5 trivia questions. (There should be a total of 10 questions.) When a question is displayed, 4 possible answers are also displayed. Only one of the answers is correct, and if the player selects the correct answer, he or she earns a point. After answers have been selected for all the questions, the program displays the number of points earned by each player and declares the player with the highest number of points the winner. To create this program, write a Question class to hold the data for a trivia question. The Question class should have attributes for the following data: A trivia question Possible answer 1 Possible answer 2 Possible answer 3 Possible answer 4 The number of the correct answer (1, 2, 3, or 4) The Question class also should have an appropriate _…arrow_forwardThe code for a class, the code for a method, and the code for while and for loops with more than ones statement is enclosed in ____. /* and */ [ and ] { and }arrow_forward9. Trivia Game In this programming exercise, you will create a simple trivia game for two players. The program will work like this: Starting with player 1, each player gets a turn at answering 5 trivia questions. (There should be a total of 10 questions.) When a question is displayed, 4 possible answers are also displayed. Only one of the answers is correct, and if the player selects the correct answer, he or she earns a point. After answers have been selected for all the questions, the program displays the number of points earned by each player and declares the player with the highest number of points the winner. To create this program, write a Question class to hold the data for a trivia question. The Question class should have attributes for the following data: A trivia question Possible answer 1 Possible answer 2 Possible answer 3 Possible answer 4 The number of the correct answer (1, 2, 3, or 4) The Question class also should have an appropriate _…arrow_forward
- The enum class construct: Select one: a. does not require one to write anything in front of an enumeration value-name b. requires one to use the name of the enumeration, followed by "::" (colon-colon), before each name whenever the enumeration's value-name is used c. requires one to use the name of the enumeration, followed by "." (period), before each name whenever an enumeration's value-name is usedarrow_forwardjava Assignment Description: Write a class named Car that has the following fields: The yearModel field is an int that holds the car’s year model. The make field references a String object that holds the make of the car. The speed field is an int that holds the car’s current speed. In addition, the class should have the following constructor and other methods. The constructor should accept the car’s year and make as arguments. These values should be assigned to the object’s yearModel and make fields. The constructor should also assign 0 to the speed field. Constructor: The constructor should accept no arguments. Constructor: The constructor should accept the car’s year as argument.Assign the value to the object’s yearModel. No need to assign anything to the make and assign 0 to speed. Appropriate mutator methods should set the values in an object’s yearModel, make, and speed fields. Appropriate accessor methods should get the values stored in an object’s yearModel, make, and…arrow_forwardJava Programming Travel Tickets Company sells tickets for airlines, tours, and other travel-related services. Because ticket agents frequently mistype long ticket numbers, Travel Tickets has asked you to write an application that indicates invalid ticket number entries. The class prompts a ticket agent to enter a six-digit ticket number. Ticket numbers are designed so that if you drop the last digit of the number, then divide the number by 7, the remainder of the division will be identical to the last dropped digit. This process is illustrated in the following example: Step 1. Enter the ticket number; for example, 123454. Step 2. Remove the last digit, leaving 12345. Step 3. Determine the remainder when the ticket number is divided by 7. In this case, 12345 divided by 7 leaves a remainder of 4. Step 4. Assign the Boolean value of the comparison between the remainder and the digit dropped from the ticket number. Step 5. Display the result—true or false—in a message box. Accept the…arrow_forward
- Java Program 01 - Theater Ticket System A small ten-seat theater has contracted you to build software which will reserve tickets. The theater has two rows of five seats each. Each row is referred to by number (1 or 2) and each seat in the row is referred to by letter (A, B, C, D, and E). When started, the program should ask the user for the row they prefer (row selection). If that row has no empty seats then the program should ask the user if they prefer the other row, if no then display a message stating the next show is tomorrow. Upon selecting a row, the program should present the user with a choice of available seats in the row. The user can then either choose a seat and print the ticket (in this case a simple message will suffice) or return to row selection. If a ticket is printed the program should return to row selection. The program terminates when all seats are reserved. Please enter a row: 1 Available seats: A B C D E Which seat, enter Q for none?: B You have…arrow_forwardJAVA CODE PLEASE THANK YOU Rectangle by CodeChum Admin A rectangle can be formed given two points, the top left point and the bottom right point. Assuming that the top left corner of the console is point (0,0), the bottom right corner of the console is point (MAX, MAX) and given two points (all "x" and "y" coordinates are positive), you should be able to draw the rectangle in the correct location, determine if it is a square or a rectangle, and compute for its area, perimeter and center point. To be able to do this, you should create a class for a point (that has an x-coordinate and a y-coordinate). Also, create another class called Rectangle. The Rectangle should have 2 points, the top left and the bottom right. You should also implement the following methods for the Rectangle: display() - draws the rectangle on the console based on the sample area() - computes and returns the area of a given rectangle perimeter() - computes and returns the perimeter of a given rectangle…arrow_forwardMonty Hall Problem – Coding Lab In this lab, you will write a code that simulates the Monty Hall Game Show. Thegame host gives the participant the choice of selecting one of three doors. Twodoors has a goat behind them and one door has a prize. The set of choices arerandomized each round. The participant needs to select the door with the prizebehind it. When the participant selects a door, the game host reveals a door with agoat behind it. The game host opens a door (different from the one selected by theparticipant) that has a goat behind it. The participant is then given the option tochange their choice. When you run your code, the code would display a message prompting the user toinput their door choice, labelled as 1, 2, and 3. Then the code will display a doornumber (different from the one the user picked) with a goat behind it and ask theuser if they would like to change their choice. The code then displays a message onwhether the user guessed the correct door. The game then…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
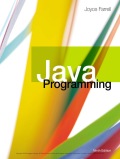
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Program to find HCF & LCM of two numbers in C | #6 Coding Bytes; Author: FACE Prep;https://www.youtube.com/watch?v=mZA3cdalYN4;License: Standard YouTube License, CC-BY